Answered step by step
Verified Expert Solution
Question
1 Approved Answer
here is the java code that i am supposed to do. The compiler from school give me that result compiler feed back: import static org.junit.Assert.*;
here is the java code that i am supposed to do. The compiler from school give me that result
compiler feed back:
import static org.junit.Assert.*; import org.junit.Test; public class OrderedStringArrayListTests { @Test public void construct() { OrderedStringArrayList a = new OrderedStringArrayList(); assertEquals("Size should be 0 after construction!", 0, a.size()); } @Test public void insertOneItem() { OrderedStringArrayList a = new OrderedStringArrayList(); assertEquals("Size should be 0 after construction!", 0, a.size()); a.insert("Hello!"); assertEquals("Size should be 1 after one insert!", 1, a.size()); } @Test public void findWithJustOneItem() { int i; OrderedStringArrayList a = new OrderedStringArrayList(); a.insert("Hello!"); i = a.find("Hello!"); assertEquals("Can't properly find the only string that should be in the list!", 0, i ); i = a.find("Hellooooooooo!"); assertEquals("find() expecting -1, but got something else", -1, i ); } @Test public void removeWithJustOneItem() { int i; OrderedStringArrayList a = new OrderedStringArrayList(); a.insert("Hello!"); i = a.find("Hello!"); assertEquals("Can't properly find the only string that should be in the list!", 0, i ); i = a.remove("Hel"+"lo!"); assertEquals("remove() expecting 1, but got something else", 1, i ); } @Test public void removeWithTwoDifferentItems() { int i; OrderedStringArrayList a = new OrderedStringArrayList(); a.insert("Hello!"); a.insert("Zeno."); i = a.find("Hello!"); assertEquals("Can't properly find the only string that should be in the list!", 0, i ); i = a.remove("Hel"+"lo!"); assertEquals("remove() expecting 1, but got something else", 1, i ); } @Test public void removeWhenThereAreDuplicates() { int i; OrderedStringArrayList a = new OrderedStringArrayList(); a.insert("Hello!"); a.insert("Hello!"); a.insert("Hello!"); a.insert("Zeno."); a.insert("Abacus"); i = a.find("Hello!"); assertEquals("find() :: doesn't find the first occurance!", 1, i ); String b = "lo!"; i = a.remove("Hel"+b); assertEquals("remove() expecting 3, but got something else", 3, i ); } @Test public void growing() { int i; OrderedStringArrayList a = new OrderedStringArrayList(); a.insert("Hello!"); a.insert("Hello!"); a.insert("Hello!"); a.insert("Hello!"); a.insert("Hello!"); a.insert("Hello!"); a.insert("Hello!"); a.insert("Hello!"); a.insert("Hello!"); a.insert("Hello!"); a.insert("Zeno."); a.insert("Abacus"); String b = "lo!"; i = a.remove("Hel" + b); assertEquals("Sorting or growing didn't work...", "Abacus", a.get(0)); assertEquals("Sorting or growing didn't work...", "Zeno.", a.get(a.size() - 1)); } @Test public void testCounting() { int i; OrderedStringArrayList a = new OrderedStringArrayList(); a.insert("Hello!"); a.insert("H"+"ello!"); a.insert("Hell"+"o!"); a.insert("Zeno."); a.insert("Abacus"); assertEquals("size() is incorrect.", 5, a.size()); assertEquals("insert() placed an item incorrectly or get() is broken", "Abacus", a.get(0)); assertEquals("insert() placed an item incorrectly or get() is broken", "Hello!", a.get(1)); assertEquals("insert() placed an item incorrectly or get() is broken", "Hello!", a.get(2)); assertEquals("insert() placed an item incorrectly or get() is broken", "Hello!", a.get(3)); assertEquals("insert() placed an item incorrectly or get() is broken", "Zeno.", a.get(4)); assertEquals("countEquivalent() is broken or insert isn't working correctly.", 1, a.countEquivalent(0)); assertEquals("countEquivalent() is broken.", 3, a.countEquivalent(1)); assertEquals("countEquivalent() is broken.", 1, a.countEquivalent(4)); i = a.find("Hello!"); assertEquals("find() :: doesn't find the first occurance!", 1, i ); i = a.remove("Hel"+"lo!"); assertEquals("remove() expecting 3, but got something else", 3, i ); } }
my code:
public class OrderedStringArrayList { private String[] array; int index = 0; public OrderedStringArrayList() { array = new String[10]; } /** * _Part 1: Implement this method._ * * Inserts a new item in the OrderedArrayList. This method should ensure * that the list can hold the new item, and grow the backing array if * necessary. If the backing array must grow to accommodate the new item, it * should grow by a factor of 2. The new item should be placed in sorted * order using insertion sort. Note that the new item should be placed * *after* any other equivalent items that are already in the list. * * @return the index at which the item was placed. */ public int insert(String item) { int j = index; // If should grow if (size() == 0) { while (j > 0 && array[j - 1].compareTo(array[j]) > 0) { array[j-1]= array[j]; array[j] = item; j = j - 1; } index++; return index; } else if (size()< array.length) { String[] temp = new String[array.length * 2]; for (j = 0; j < size(); j++) { temp[j] = array[j]; while (j > 0 && array[j - 1].compareTo(temp[j]) > 0) { array[j - 1] = temp[j]; temp[j] = array[j]; array[j] = item; j = j - 1; }} }return index; } /** * _Part 2 Implement this method._ * * @return the number of items in the list. */ public int size () { return array.length; } /** * _Part 3: Implement this method._ * * Gets an item from the ordered array list. You can assume that this method * will only be called with valid values of index. Specifically, values that * are in the range 0 - (size-1). To impress your friends and build your * street cred, consider adding checks that the index supplied is in fact in * bounds. If it is not, you can raise an IndexOutOfBoundsException. * * @param index the index to get an item from * @return an item at the specified index */ public String get ( int index){ // TODO: implement this return null; } /** * _Part 3: Implement this method._ * * Counts the items in the ordered array list that are equal to the item at * the specified index. Be sure to take advantage of the fact that the list * is sorted here. You should not have to run through the entire list to * make this count. * * @param index an index in the range 0..(size-1) * @return the number of items in the list equal to the item returned by * get(index) */ public int countEquivalent ( int index){ // TODO: implement this return 0; } /** * _Part 4: Implement this method._ * * Finds the location of the first object that is equal to the specified * object. Linear search is sufficient here, but for 5 points of extra credit * implement binary search. * * @param obj an object to search for in the list * @return the first index of an object equal to the one specified, or -1 if * no such object is found. */ public int find (String obj){ // TODO: implement this return 0; } /** * _Part 5: Implement this method._ * * Removes all the objects equal to the specified object. * * @param obj an object equal to the one(s) you'd like to remove * @return the number of objects removed */ public int remove (String obj){ // TODO: implement this return 0; } /** * This method is included for testing purposes. * Typically, you would not want to expose a private instance variable * as we are doing here. However, it does have value when the code is * going through a testing phase. Do not modify or remove this method. * Some Autolab tests may rely upon it. */ public String[] dbg_getBackingStore () { return array; } /** * _Part 6 (optional): Implement this_ * * Exercise your list to demonstrate it works as expected. */ public static void main(String[] args) { OrderedStringArrayList name = new OrderedStringArrayList(); name.insert("Bob"); name.insert("Alex"); // name.insert("Ann"); //name.insert("John"); //name.insert("Jack"); // name.insert("Jordan"); // name.insert("Stephen"); // name.insert("Maggy"); //name.insert("Donald duck"); // name.insert("Casper"); // for (int j = 0; j < name.size(); j++) { System.out.println(name.array[j]); }return ; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
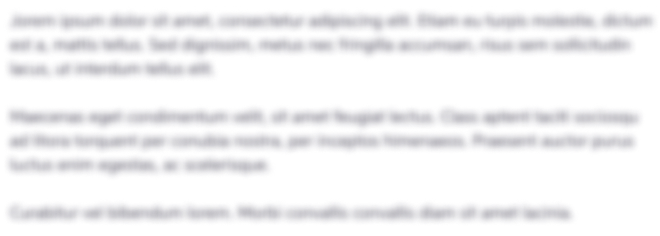
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started