Question
Here is the problem. The code provided below displays nine panels of different colors in a 3 X 3 grid. Whenever the mouse enters a
Here is the problem.
The code provided below displays nine panels of different colors in a 3 X 3 grid. Whenever the mouse enters a panel, it turns magenta; when the mouse leaves, the color reverts back to its original color. Add the following user story: "When the user clicks on the center panel, the colors of the eight surrounding panels rotate to the next panel in a clockwise direction." Remember to TDD.
When complete, add this user story: "When the user consecutively clicks on two of the outer panels, the colors of these panels are swapped."
import java.awt.Color;
import java.awt.GridLayout;
import javax.swing.JFrame;
public class Init {
public JFrame jFrame;
MyJPanel panels[];
Color colors[] = { Color.blue, Color.green, Color.red, Color.white, Color.yellow, Color.black, Color.orange,
Color.cyan, Color.gray };
public Init() {
panels = new MyJPanel[9];
jFrame = new JFrame("NINE SQUARES PROGRAM");
jFrame.setSize(500, 500);
jFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
jFrame.getContentPane().setLayout(new GridLayout(3, 3));
for (int i = 0; i < panels.length; i++) {
panels[i] = new MyJPanel(colors[i]);
jFrame.getContentPane().add(panels[i]);
}
jFrame.setVisible(true);
}
public static void main(String[] args) {
new Init();
}
}
___________________________________________________________________________________________________
import java.awt.Color;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
import javax.swing.JPanel;
@SuppressWarnings("serial")
public class MyJPanel extends JPanel {
private Color myColor;
public MyJPanel(Color myColor) {
this.myColor = myColor;
setBackground(myColor);
addMouseListener(new MouseWatcher());
}
public void setMyColor(Color myColor) {
this.myColor = myColor;
setOriginalColor();
}
public void setSelectedColor() {
setBackground(Color.magenta);
}
public void setOriginalColor() {
setBackground(myColor);
}
class MouseWatcher extends MouseAdapter {
@Override
public void mouseEntered(MouseEvent me) {
setSelectedColor();
}
@Override
public void mouseExited(MouseEvent me) {
setOriginalColor();
}
@Override
public void mouseClicked(MouseEvent me) {
clicked();
}
}
public void clicked() {
}
}
_______________________________________________________________________________________________________________________-
import java.awt.Color;
@SuppressWarnings("serial")
public class CenterPanel extends MyJPanel {
Init init;
public CenterPanel(Color myColor, Init init) {
super(myColor);
this.init = init;
}
@Override
public void clicked() {
}
}
_____________________________________________________________________________________________________
import static org.junit.Assert.assertSame;
import org.junit.Before;
import org.junit.Test;
class InitTest {
Init init;
@Before
public void setUp() {
init = new Init();
}
@Test
public void rotating_colors() {
init.panels[4].clicked();
assertSame(init.colors[3], init.panels[0].getBackground());
assertSame(init.colors[0], init.panels[1].getBackground());
assertSame(init.colors[1], init.panels[2].getBackground());
assertSame(init.colors[6], init.panels[3].getBackground());
assertSame(init.colors[2], init.panels[5].getBackground());
assertSame(init.colors[7], init.panels[6].getBackground());
assertSame(init.colors[8], init.panels[7].getBackground());
assertSame(init.colors[5], init.panels[8].getBackground());
}
}
______________________________________________________________________________________________________________________-
import static org.junit.Assert.assertSame;
import java.awt.Color;
import org.junit.Before;
import org.junit.Test;
class MyJPanelTest {
private static final Color COLOR = Color.BLACK;
private static final Color MAGENTA = Color.magenta;
MyJPanel myJPanel;
@Before
public void setUp() {
myJPanel = new MyJPanel(COLOR);
}
@Test
void set_selected_color() {
myJPanel.setSelectedColor();
assertSame(MAGENTA, myJPanel.getBackground());
}
@Test
void set_original_color() {
myJPanel.setOriginalColor();
assertSame(COLOR, myJPanel.getBackground());
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
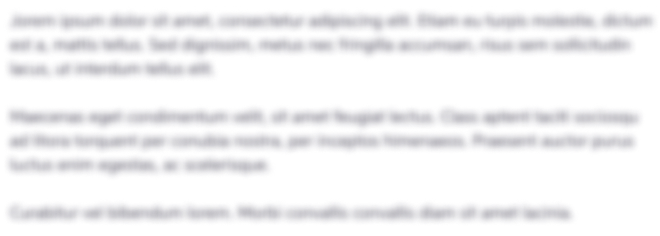
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started