Question
Here is what I have so far: # create a shared list theList = [] def printMenu(): pass def addToList(item): pass def getInput(): print('You have
Here is what I have so far:
# create a shared list
theList = [] def printMenu(): pass def addToList(item): pass def getInput(): print('You have 0 items on your list.') return input('Enter an item or command: ') def printList(): print('Your shopping list:') def emptyList(): pass def startProgram(): print('Welcome to the XYZ Shopping List Program') def endProgram(): print('Thank you for using the XYZ Shoppling List Program.') def main(): startProgram() printMenu() item = getInput() while item != '-x': if item == '-p': printList() elif item == '-h': printMenu() elif item == '-e': emptyList() else: addToList(item) item = getInput() endProgram() main()
And here are the parameters of what I am trying to do
For this assignment, you will create a simple shopping list application. This application will allow the user to add items to a shopping list, review the contents of the list, and remove all items from the list. The implementation uses a list. In the minimal assignment, the shopping list will not persist between runs of the application.
Notice that there is a global variable, theList. This variable is global so that it can be shared by all of the functions in this file. There is no need to use the global keyword at all in this assignment. We will not assign to the variable theList except for when it is created.
The implementation of the application is incomplete. The task for the Minimal version is to complete the application, by implementing the functions as described below.
Before you begin working on the assignment, make sure that you read through the code to understand how it is put together. Also run the program to see what the skeleton does.
Implement the basic functions
printMenu The first task is to implement the printMenu function. This function will print out a menu of the commands that are available. When the printMenu function is called it will print out:
Here are the list of commands: -p : Print the List -e : Empty the List -x : Exit -h : Print this command list
If you run the program, you should now print the menu before asking for the first command.
addToList The next function we will implement in addToList. This function has a parameter, the value to add to the list. This value should be added to the end of the shared variable, theList.
When an item is added to the list, report the addtion.
getInput Notice that the getInput function reports the number of items in the shopping list. It is hard-coded to say that there are zero items in the list. Update the getInput function to display the accurate count of the number of items in the shopping list.
printList Complete the printList function so that it will display the items in the list. Print out the items as a simple bulleted list. For example:
Your shopping list: * pizza * milk * bread
Use a loop to process through the contents of the shared list. Print out each value in the list, prefixing the value with space, space, star, space.
emptyList The final function we need to implement is emptyList. It will not work to set the value of theList to empty square brackets.
def emptyList(): theList = []
Since you cannot set the value of the variable, you will need to manipulate the list object to remove the items within the list. This is the trickiest part of the Minimal version.
When the list is emptied, report this using a simple message.
Fault Tolerance
The application needs to be fault-tolerant. We don't want to add blank items into the shopping list. So, we need check to make sure that there is something in the user input. If the user input is blank (or consists only of white space), print out an error message, and ask again. Here is an example:
Shopping list items cannot be blank.
This is a change you can make within the getInput function. Nothing else will need to change to implement this. Make sure that the program will repeatedly ask for input until something that is not blank is entered.
Do not use recursion. Recursion is when a function calls itself.
Comments
Here is an example of running the completed program. (User input is shown in bold blue.)
Welcome to the XYZ Shopping List Program. Here are the list of commands: -p : Print the List -e : Empty the List -x : Exit -h : Print this command list You have 0 items on your list. Enter your item or command: pizza 'pizza' added to the list. You have 1 items on your list. Enter your item or command: milk 'milk' added to the list. You have 2 items on your list. Enter your item or command: bread 'bread' added to the list. You have 3 items on your list. Enter your item or command: -p Your shopping list: * pizza * milk * bread You have 3 items on your list. Enter your item or command: -h Here are the list of commands: -p : Print the List -e : Empty the List -x : Exit -h : Print this command list You have 3 items on your list. Enter your item or command: -e All items removed from list. You have 0 items on your list. Enter your item or command: Shopping list items cannot be blank. You have 0 items on your list. Enter your item or command: Shopping list items cannot be blank. You have 0 items on your list. Enter your item or command: apples 'apples' added to the list. You have 1 items on your list. Enter your item or command: Shopping list items cannot be blank. You have 1 items on your list. Enter your item or command: -p Your shopping list: * apples You have 1 items on your list. Enter your item or command: -x Thank you for using the XYZ Shopping List Program.
Standard Option
For the Standard assignment, there are three enhancements to the Minimal assignment:
- No duplicates
- Numbered list
- Single Item Removal
No Duplicates
Update the add function to check for duplicates before actually adding the item to the list. If the new item already exists in the list, report the duplicate and do not add it to the list. If it does not exist in the list, add it to the list. Make sure the user can print the list if they just got the duplicate message.
This is an update to the addToList function.
Numbered List
Update the function that prints the list to create a number list rather than a bulleted list. Notice this list is numbered the way people number lists, not the way computers do. (That is, it starts at one, rather than zero.)
Your shopping list: 1. pizza 2. milk 3. bread
This is an update to the printList function.
Single Item Removal
Add an add command that removes a single item from the list. This command can be -r n, where n is the number or value of the item to be removed from the list.
You have 3 items on your list. Enter your item or command: -p Your shopping list: 1. pizza 2. milk 3. bread You have 3 items on your list. Enter your item or command: -r 1 Item 1 removed from the list. You have 2 items on your list. Enter your item or command: -p Your shopping list: 1. milk 2. bread You have 2 items on your list. Enter your item or command: -r bread 'bread' removed from the list. You have 1 items on your list. Enter your item or command: -p Your shopping list: 1. milk
Create a function that will remove a single item from the list. This method will take as a single parameter: which item to be removed. Notice that the parameter could be a pseudo-index value or the string value for the item.
Start by assuming that the parameter is the value to be removed. Check to see if the parameter value is in the list. If it is remove it from the list and report the removal. If the parameter value is not in the list, check to see if you can convert it to a positive integer value. If it cannot be converted, report that the item could not be located. Otherwise check that the "pseudo-index" value is in range. If it is remove the corresponding item and report the removal. If the pseudo-index value is out of range, report an error.
It is probably easiest to create a new function for this functionality.
def removeFromList(item): pass
And then call this function from main.
Update printMenu to reflect the new command.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
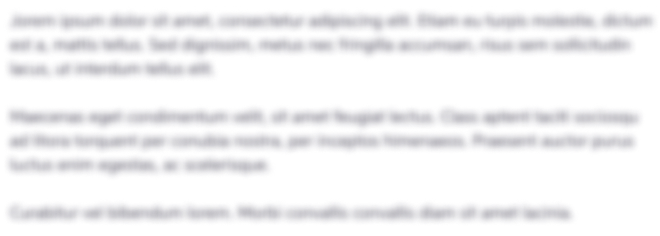
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started