Question
Here signal handler is defined but I need to use it in the main program I did not see the function is used. Can you
Here signal handler is defined but I need to use it in the main program I did not see the function is used. Can you help to correct this code
#include
#include
#include
#include
#include
#include
#include
#include
#include
#include
// This is a structure class that is used to read data from
// the file where character as letter and short integer as the the number
struct pairs
{
char letter;
short int number;
};
// Function to count the number of set bits in a binary representation
int count_set_bits(int num1)
{
int count = 0;
while (num1)
{
count += num1 & 1; // Doing bit-wise comparision with the AND (&) Operator and the number 1
num1 >>= 1; // The binary bit shifts to the right by one digit
}
return count;
}
void timer_handler(int sig)
{
printf("Timer expired. Exiting program. ");
exit(0);
}
int main()
{
char c;
int num;
FILE *file1; // This is where the FILE *file pointer is used to access the file
struct pairs char_int; // We are declaring char_int as the structure
file1 = fopen("error.dat", "rb"); // The file image.dat is being opened and assigned the
// pointer to File 1
if(!file1) // This is a if-condition loop to check if error occurs while opening file
{
printf("File cannot be opened or found ");
return 1;
}
struct sigaction sa;
memset(&sa, 0, sizeof(sa));
sa.sa_handler = &timer_handler;
sigaction(SIGALRM, &sa, NULL);
// Set timer for 60 seconds
alarm(60);
// Read a character-integer pair every second
while (fread(&char_int, sizeof(struct pairs), 1, file1))
{
// Compute the parity of the pair
c = char_int.letter;
num = char_int.number;
int parity = count_set_bits(c) + count_set_bits(num); // Adding counting number of bits set to 1 in both char and integer
if (parity % 2 == 0) // Checking if parity is even using % operator
{
// If parity is even, the data is valid
int original_num = num;
printf("(%c, %d) ",c ,original_num); // This will give the original value of the character and pr
for (int i = 0; i < char_int.number; i++) // This loop is used to display the decoded form of the pairing of character and integer
// by printing the character so many times until it reaches the value of the integer
// It will start from 0 and continues until i is less than integer
{
printf("%c", char_int.letter); // This print function is used to print the character
// retrieved from the file
}
printf(" "); // Each record is printed on a new line
}
else
{
// The parity is odd, so the data is corrupted
printf("Error: Odd parity ");
}
sleep(1); // This uses a sleep system call for the specified number of seconds until interrupted
// Reading 1 character integer pair every second here 1 corresponds to every second
}
fclose(file1); // Closing the file after successfully reading the data
return 0;
}
``
Step by Step Solution
There are 3 Steps involved in it
Step: 1
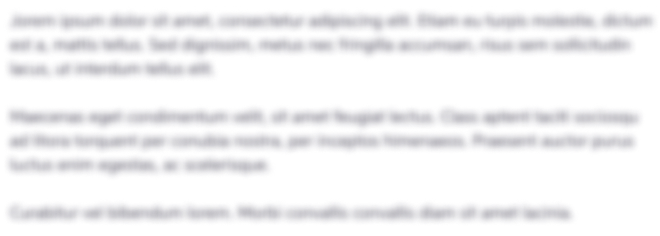
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started