Question
Here you will be writing a function: double Sin(float); to compute the sin of an angle (given in radians) using the Taylor series shown. Your
Here you will be writing a function:
double Sin(float);
to compute the sin of an angle (given in radians) using the Taylor series shown. Your function should find the value of the sin for ANY angle (preferred) or at least angles up to 2 PI (Hint: if you can do the later, the former should be easy) to a precision of 4 decimal places (within 0.00005). You should put the function Sin() in the file called trig.c. You can use the function pos_power() in your exponent.c from Lab 6 and Hw 4, and you should write functions for factorial() and close_enough() in the file called util.c. This last function is given two doubles and returns true if they are within 0.00005 of each other. You can put any other functions you may want in util.c as well. You will need to provide your own .h files for each of these .c files. You should also write a simple test driver to exercise your function in the file driver1.c. You should update the makefile to compile and link driver1.c, trig.c, util.c and exponent.c when you type
make trig
and create the executable called trig.
I got it to work correctly up until 2.14, anything after that and it doesn't output an answer and I don't know why or how to fix it.
TRIG.C
#include #include "trig.h" #include "exponent.h" #include "util.h" #define PI 3.1415
double Sin(double angle) { int i = -1; int close = 2; int count = 0; double total = 0.0; double previous = 0.0;
while(close != 0) { i++; previous = total + (pos_power(-1.0, i) * pos_power(angle, (2 * i) + 1)/ factorial((2 * i) + 1)); i++; total = previous + (pos_power(-1.0, i) * pos_power(angle, (2 * i) + 1)/ factorial((2 * i) + 1)); close = close_enough(total, previous); } return total; }
EXPONENT.C
#include #include "exponent.h"
double pos_power(double x, int y) { double result = 1.0;
while(y != 0) { result *= x; --y; } return result; }
UTIL.C
#include #include "util.h" double factorial(int a) { int subtotal = 1;
while(a > 1) { subtotal = subtotal * a; a = a - 1; } return subtotal; }
int close_enough(double a, double b) { double c = a - b;
if(c < 0) { c = c * (-1); } if(c <= 0.00005) return 0; else return 1; }
DRIVER1.C
#include #include "trig.h"
int main() { double x; double ans; printf("Enter x value in degrees: "); while(scanf("%lf", &x) != EOF) { ans = Sin(x); printf("Value of sin(%lf) = %lf ", x, ans); printf("Enter x value in degrees: "); } printf(" "); }
SAMPLE OUTPUT:
Values of sin(x)
sin(0) = 0, sin(1) = 0.841471, sin(1.57) = 1.000000, sin(3.14) = 0.001593, sin(6.28) = -0.003185,
sin(10) = -0.544021, sin(100) = -0.506366, sin(1000) = 0.826880
sin(-10) = 0.544021, sin(-100) = 0.506366
This was a hint my TA gave me:
Just make use of the periodicity of sine function: sin(2k? + x) = sin(x).
You can repeatedly add 2? to x (for negative x) or subtract 2? from x (for positive x) until x falls into the range of [0, 2 ?]. Another approach is to use modular operator "%" to obtain the remainder of x divided by 2?.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
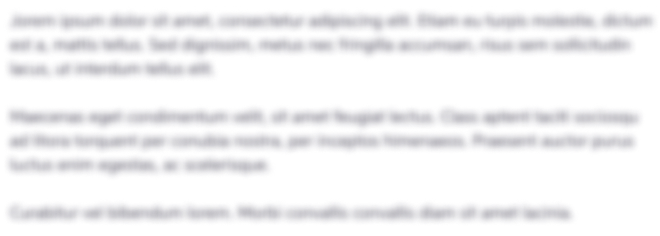
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started