Question
Here's my assignment Add to the project a CheckingAccount class and a LongTermSavingsAccount class that each inherit from Account and supports the following: Checking accounts
Here's my assignment
Add to the project a CheckingAccount class and a LongTermSavingsAccount class that each inherit from Account and supports the following:
Checking accounts have the same properties as an Account and also have a 10-digit routing number (that could start with a 0 and is included in its identification)
Checking accounts can also have a negative balance, but as soon as that happens, the account is flagged as negative and does not allow for further withdrawals. More funds can be added to the account to get it out of the negative state
Long term savings accounts have the same properties as an Account and a flag that indicates whether or not it is enabled for deposit and withdraw transactions
If the flag is set to false, no deposits or withdraws are allowed
If the flag is set to true, only withdraws are allowed. Deposits remain disabled
For both classes, ensure all the appropriate set/get, constructors and toString methods are defined
For the main program, ensure all methods and object instantiations are tested
i'm having trouble with the rest of my program. here's what i have so far. i am stuck on the longtermsavingsaccount class
package exercise6; import java.time.LocalDateTime; /** * * @author johnnysantillan */ public class Account { protected String id; protected double balance; protected DateTime dateCreated; protected void setDateCreated() { LocalDateTime now = LocalDateTime.now(); dateCreated = new DateTime(now.getMonthValue(), now.getDayOfMonth(), now.getYear(), now.getHour(), now.getMinute(), now.getSecond()); } public Account() { id = ""; balance = 0.0; setDateCreated(); }
public Account(String i, double b) { id = i; balance = b; setDateCreated(); } public Account(Account a) { id = a.getId(); balance = a.getBalance(); dateCreated = a.getDateCreated(); } public String getId() { return id; } public double getBalance() { return balance; } public DateTime getDateCreated() { return new DateTime(dateCreated); } public void setId(String i) { id = i; }
public boolean deposit(double amount) { balance += amount; return true; } public boolean withdraw(double amount) { if (amount > balance) return false;
balance -= amount; return true; } public String toString() { return String.format("%s: $%.2f and was created on %s", id, balance, dateCreated); } }
public class CheckingAccount extends Account { private String routingNumber; private double overdraft; public CheckingAccount() { super(); routingNumber = ""; overdraft = 0; } public CheckingAccount(String i, double b, double oDraft) { super(i, b); overdraft = oDraft; } public double getOverdraftAmount() { return (overdraft); } public void setOverdraftAmount() { if(balance < overdraft) System.out.println("Your Account Is Overdrawn"); } @Override public boolean withdraw(double amount) { if (balance - amount > getOverdraftAmount()) return false; balance = balance - amount; return true; } }
public class SavingsAccount extends Account { private double interestRate; private int withdrawCount;
public SavingsAccount() { super(); interestRate = 0.0; withdrawCount = 0; }
public SavingsAccount(String i, double b, double ir) { super(i, b); interestRate = ir; withdrawCount = 0; }
public SavingsAccount(SavingsAccount a) { super(a); interestRate = a.getInterestRate(); withdrawCount = a.getWithdrawCount(); }
public double getInterestRate() { return interestRate; }
public int getWithdrawCount() { return withdrawCount; } public boolean deposit(double amount) { balance += amount; return true; } @Override public boolean withdraw(double amount) { if (amount > balance || withdrawCount == 3) return false; balance -= amount; withdrawCount++; return true; }
public boolean withdraw() { return true; } public void setInterestRate(double i) { interestRate = i; } public void applyInterest() { balance += balance * (interestRate / 100); } public void incrementWithdrawCount() { withdrawCount++; } public void resetWithdrawCount() { withdrawCount = 0; } public String toString() { return String.format("%s: $%.2f at %.2f%% has %d withdraws and was created on %s", id, balance, interestRate, withdrawCount, dateCreated); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
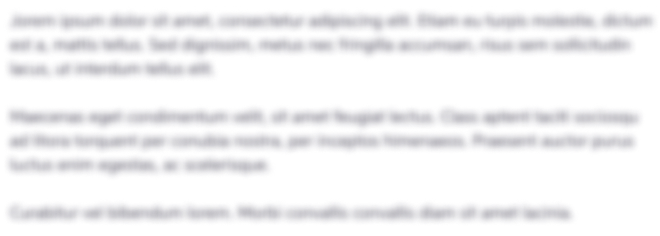
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started