Question
Hey, I need help with this easy C++ code. These are very short, thank you in advance. I will upvote. Exercise 3 IMPLEMENT IT USING
Hey, I need help with this "easy" C++ code. These are very short, thank you in advance. I will upvote.
Exercise 3
IMPLEMENT IT USING AN ENHANCED FOR LOOP (ForEach)
In this exercise, we are going to count how many Students are taking the course ICOM or CIIC.
Complete the method vector
NOTE: DO NOT USE THE TERNARY OPERATION NOR SUBMIT COMMENTS FOR THE SOLUTION
//Test
Student s4(3, "Ariel", FEMALE,"CIIC", 4.0); Student s5(4, "Mulan", FEMALE, "ICOM", 3.1); Student s6(5, "Aladin", MALE, "CIIC", 3.1); vectortestVector2; testVector2.push_back(s4); testVector2.push_back(s5); testVector2.push_back(s6); vector result = Student::countStudents(testVector2, "ICOM"); cout << "ID of Students with code: " << Student::toString(result) << endl;
//Result
ID of Students with code: {4,}
Exercise 4
USE A DO/WHILE LOOP
In this exercise, we are going to find the Students that have a high GPA, depending on the criteria.
Complete the method vector
NOTE: DO NOT SUBMIT ANY COMMENTS ON THE SOLUTION
//Test
Student s4(3, "Ariel", FEMALE,"CIIC", 4.0); Student s5(4, "Mulan", FEMALE, "ICOM", 3.56); Student s6(5, "Aladdin", MALE, "CIIC", 3.1); vectortestVector2; testVector2.push_back(s4); testVector2.push_back(s5); testVector2.push_back(s6); vector temp = Student::findStudents(testVector2, 3.50); cout << "Students with a high GPA: " << Student::toString(temp) << endl;
//Result
Students with a high GPA: {{3,Ariel,F,4.000000},{4,Mulan,F,3.560000},}
Code starts here
Three(3) src
main.cpp, Student.cpp, Student.h
//main.cpp
#include "Student.h"
using namespace std;
int main() {
Student s1(0, "Bienve", MALE, 3.0);
Student s2(1, "Jose Juan", MALE, 2.8);
Student s3(2, "Ana", FEMALE, 3.5);
Student s4(3, "Ariel", FEMALE,"CIIC", 4.0);
Student s5(4, "Mulan", FEMALE, "ICOM", 3.56);
Student s6(5, "Aladdin", MALE, "CIIC", 3.1);
vector
testVector1.push_back(s1);
testVector1.push_back(s2);
testVector1.push_back(s3);
vector
testVector2.push_back(s4);
testVector2.push_back(s5);
testVector2.push_back(s6);
cout << "---------TESTS---------" << endl;
cout << " ----Exercise #3----" << endl;
vector
cout << "ID of Students with code: " << Student::toString(result) << endl;
cout << " ----Exercise #4----" << endl;
vector
cout << "Students with a high GPA: " << Student::toString(temp) << endl;
}
//Student.cpp
#include "Student.h"
using namespace std;
/*
* EXERCISE #3
*
* IMPLEMENT IT USING AN ENHANCED FOR LOOP (ForEach)
*
* Given the course code (CIIC or ICOM), you must return a vector
* that contains ONLY the unique ID of the Students that have on record
* a that course code.
*/
vector
vector
//YOUR CODE HERE
return result;
}
/*
* EXERCISE #4
*
* IMPLEMENT USING A DO...WHILE LOOP
*
* Return a vector that contains all the Students that have a GPA greater
* or equal to the GPA passed as the parameter
*
* Assume the list contains at least one element
*/
vector
//YOU CODE HERE
return v;
}
}
//Student.h
#include
#include
#include
using namespace std;
enum Gender{
MALE,
FEMALE
};
class Student{
private:
long id; // Unique ID
string name; // Name of student
Gender gender; // Gender of student
string courseCode; // Course code (CIIC or ICOM)
double gpa; // GPA of student
public:
Student(long id, const string &name, Gender gender, double gpa){
this->id = id;
this->name = name;
this->gender = gender;
this->courseCode = "";
this->gpa = gpa;
}
Student(long id, const string &name, Gender gender, string courseCode, double gpa){
this->id = id;
this->name = name;
this->gender = gender;
this->courseCode = courseCode;
this->gpa = gpa;
}
Student(){}
static string toString(Student& s){
string genderLetter = (s.gender == MALE ? "M" : "F");
return string("{" + to_string(s.id) + "," + s.name + "," + genderLetter + "," + to_string(s.gpa) + "}");
}
static string toString(vector
string result = "{";
for (Student s : v) {
result += toString(s) + ",";
}
result += "}";
return result;
}
static string toString(vector
string result = "{";
for (long id : v) {
result += to_string(id) + ",";
}
result += "}";
return result;
}
// Getters
long getID(){return id;}
string getName(){return name;}
Gender getGender(){return gender;}
double getGPA(){return gpa;}
string getCourseCode(){return courseCode;}
//Setters
void setName(string name){this->name = name;}
void setGender(Gender gender){this->gender = gender;}
void setGPA(double gpa){this->gpa = gpa;}
void setCourseCode(string code){this->courseCode = code;}
// EXERCISES
static vector
static void removeByID(vector
};
Step by Step Solution
There are 3 Steps involved in it
Step: 1
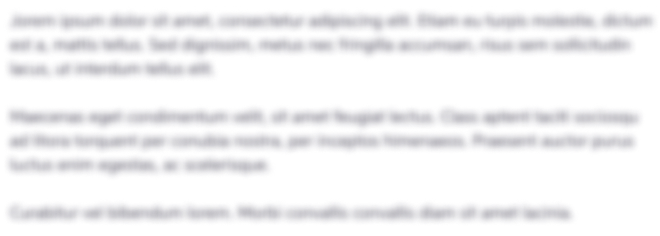
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started