Question
Hey, I would be grateful for assistance with part c) of this question. I have included my code for part a) and b) . Note
Hey, I would be grateful for assistance with part c) of this question. I have included my code for part a) and b) .
Note each classes developed in this question must have get and set methods for each field and any other constructors and methods you deem appropriate.
a) Create a CalculateGrade interface which specifies two methods
i) calculateGradeAverage
ii) assignGrade
b) Design a Class which represents a Student which implements CalculateGrade. It is to have the following fields, Name, Student Number, Course, Current Year , Registration Status, An Arraylist of marks for the 10 topics the student is studying as part of their Course. You are to implement i) calculateGradeAverage to calculate the average of all the subject marks recorded for the student and ii) assignGrade to output a corresponding grade for the calculated grade average according to the scheme
A grade for a grade average over 80
B grade for a grade average greater than or equal to 70 and less than 80
C grade for a grade average greater than or equal to 60 and less than 70
D grade for a grade average greater than or equal to 50 and less than 60
E grade for a grade average greater than or equal to 40 and less than 50
F grade for a grade average less than 40
c) Design an Undergraduate subclass of the Student class. It is to have the following extra fields: stream which indicates the particular stream of a course a student is following e.g. data Analytics in a Computer Science course, and a field representing their Final year project topic. You are to have a method that works out the initials of an Undergraduate and displays these. You are also to have a method which displays a warning for any Undergraduate whose grade average is less than a D grade.
//My Java code for parts a) and b)
CalculateGrade.java
public interface CalculateGrade {
public double calculateGradeAverage();
public void assignGrade();
}
Student.java
import java.util.ArrayList;
public class Student implements CalculateGrade{
// Data members
private String name;
private int studentNumber;
private String course;
private int year;
private String status;
private ArrayList
// Constructor to initialize data members
public Student(String name, int studentNumber, String course, int year, String status, ArrayList
this.name = name;
this.studentNumber = studentNumber;
this.course = course;
this.year = year;
this.status = status;
this.marks = marks;
}
// Getter and setter methods
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getStudentNumber() {
return studentNumber;
}
public void setStudentNumber(int studentNumber) {
this.studentNumber = studentNumber;
}
public String getCourse() {
return course;
}
public void setCourse(String course) {
this.course = course;
}
public int getYear() {
return year;
}
public void setYear(int year) {
this.year = year;
}
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public ArrayList
return marks;
}
public void setMarks(ArrayList
this.marks = marks;
}
@Override
public double calculateGradeAverage() {
double average = 0;
// Iterate over marks list
// Add marks to variable average
for(Integer m:marks) {
average+=m;
}
// Divide average by size of marks list
// Return average
average = average/marks.size();
return average;
}
@Override
public void assignGrade() {
char grade;
// Call function and get average
// Set grade value from the given conditions
double average = calculateGradeAverage();
if(average>=80) {
grade = 'A';
}else if(average>=70 & average<80) {
grade = 'B';
}else if(average>=60 & average<70) {
grade = 'C';
}else if(average>=50 & average<60) {
grade = 'D';
}else if(average>=40 & average<50) {
grade = 'E';
}else {
grade = 'F';
}
System.out.println("Average = "+average);
System.out.println("Grade = "+grade);
}
// Print student details
public String toString() {
return "Name: "+this.name+
" Student Number: "+this.studentNumber+
" Course: "+this.course+
" Year: "+this.year+
" Status: "+this.status+
" Marks: "+this.marks;
}
}
Q.java
import java.util.ArrayList;
import java.util.Arrays;
public class Q {
public static void main(String[] args) {
// Two samples cases
Student student1 = new Student("Nancy",101,"CSE",2018,"Successful",
new ArrayList
System.out.println(student1);
student1.assignGrade();
System.out.println();
Student student2 = new Student("Maddy",102,"CSE",2018,"Successful",
new ArrayList
System.out.println(student2);
student2.assignGrade();
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
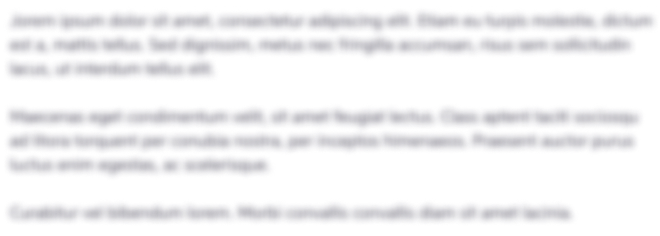
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started