Question
Hey there, I am supposed to implement the functions described in the LinkedList class. Most of them have been implemented but I am having a
Hey there, I am supposed to implement the functions described in the LinkedList class. Most of them have been implemented but I am having a hard time figuring out the final function (toString) and would love some help. Thanks!
----------------------------------------------------------------------------------------------------------------------------------------------------------------
#pragma once //#include "LinkedList.h"
template class Node {
// Node(T value)
//public: // get and set functions that allow me to change the private variables
public:
T data; Node* next;
Node(T value, Node* n = NULL) { data = value; next = n; }
T getData(){ return data; }
void setData(T newdata){ data = newdata; }
Node* getNext(){ return next; }
void setNext(Node* n){ next = n; } };
----------------------------------------------------------------------------------------------------------------------------------------------------------------
#pragma once #include "LinkedListInterface.h" #include "Node.h"
template class LinkedList : public LinkedListInterface {
/*public LLI{ }*/
//store pointer somewhere in here that points to the head
public:
Node* head;
LinkedList(){head = NULL;} ~LinkedList(){ clear(); }
bool hasDup(T value){ Node* dupCheck = head; while(dupCheck != NULL){ if (dupCheck->data == value){ return true; } dupCheck = dupCheck->next; } return false; } /* insertHead A node with the given value should be inserted at the beginning of the list. Do not allow duplicate values in the list. */ virtual void insertHead(T value) { if (hasDup(value)){ return; } if (head == NULL){ head = new Node(value); } else{ // to do...duplicates Node* temp = new Node(value); temp->next = head; head = temp; } }
/* insertTail A node with the given value should be inserted at the end of the list. Do not allow duplicate values in the list. */ virtual void insertTail(T value) { if (head == NULL){ insertHead(value); return; }
if (hasDup(value)){ return; } Node* temp = head; while(temp->next != NULL){ temp = temp->next; } if (temp->next == NULL){ Node* newNode = new Node(value); temp->next = newNode; newNode->next = NULL; } }
/* insertAfter A node with the given value should be inserted immediately after the node whose value is equal to insertionNode. A node should only be added if the node whose value is equal to insertionNode is in the list. Do not allow duplicate values in the list. */ virtual void insertAfter(T value, T insertionNode) { if (hasDup(value) == true){ return; } Node* curr = head; while(curr != NULL){ if (curr->data == insertionNode){ Node* newNode = new Node(value); newNode->next = curr->next; curr->next = newNode; } curr = curr->next; } } /* remove The node with the given value should be removed from the list. The list may or may not include a node with the given value. */ virtual void remove(T value) { Node* curr = head; Node* deleteMe = head; if (head->data == value){ // check to see if it's the head we want to remove head = curr->next; delete curr; return; } while(curr->next != NULL){ if (curr->next->data == value){ deleteMe = curr->next; curr->next = deleteMe->next; deleteMe->next = NULL; delete deleteMe; return; } curr = curr->next; } }
/* clear Remove all nodes from the list. */ virtual void clear(){ while (head != NULL){ Node* n = head->next; delete head; head = n; }
} /* at Returns the value of the node at the given index. The list begins at index 0. If the given index is out of range of the list, return NULL; */ virtual T at(int index) { if (index >= size() || index < 0){ return NULL; } else{ Node* n = head; int i = 0; while (n != NULL){ if(i == index){ return n->data; } i++; n = n->next; } } } /* size Returns the number of nodes in the list. */ virtual int size() { Node* n = head; int i = 0; while (n != NULL){ n = n->next; i++; } return i; }
/* toString Returns a string representation of the list, with the value of each node listed in order (Starting from the head) and separated by a single space There should be no trailing space at the end of the string
For example, a LinkedList containing the value 1, 2, 3, 4, and 5 should return "1 2 3 4 5" */ virtual string toString{ ;
};
};
Step by Step Solution
There are 3 Steps involved in it
Step: 1
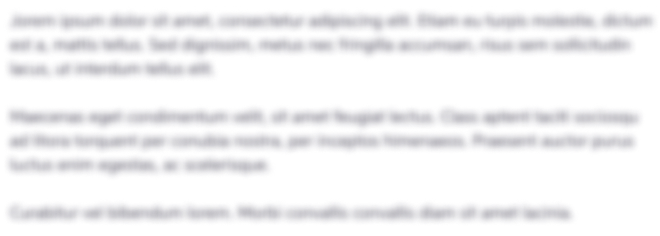
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started