Question
Hi can someone help me with this one error line 13 /* Chapter 9: Maintaining a Phone Book with ArrayLists Date: September 19, 2017 Programmer:
Hi can someone help me with this one error line 13
/* Chapter 9: Maintaining a Phone Book with ArrayLists Date: September 19, 2017 Programmer: Purpose: This program uses arraylists to maintain a phone book */ import javax.swing.*; import java.awt.*; import java.awt.event.*; import java.util.*; public class PhoneBook extends JFrame implements ActionListener { ArrayList nameList; ArrayList phoneList; String name; String phone; double phoneNum; int index; JPanel northPanel = new JPanel(); JPanel centerPanel = new JPanel(); JPanel southPanel = new JPanel(); JLabel nameLabel = new JLabel("Name"); JTextField nameField = new JTextField(20); JLabel phoneLabel = new JLabel("Phone"); JTextField phoneField = new JTextField(20); JButton addBttn = new JButton("Add"); JButton deleteBttn = new JButton("Delete"); JButton updateBttn = new JButton("Update"); JButton findBttn = new JButton("Find"); public PhoneBook() { super ("Phone Book"); Container c = getContentPane(); c.setLayout(new BorderLayout()); northPanel.setLayout(new FlowLayout(FlowLayout.LEFT)); centerPanel.setLayout(new FlowLayout(FlowLayout.LEFT)); southPanel.setLayout(new FlowLayout()); northPanel.add(nameLabel); northPanel.add(nameField); centerPanel.add(phoneLabel); centerPanel.add(phoneField); southPanel.add(addBttn); southPanel.add(deleteBttn); southPanel.add(updateBttn); southPanel.add(findBttn); c.add(northPanel,BorderLayout.NORTH); c.add(centerPanel,BorderLayout.CENTER); c.add(southPanel,BorderLayout.SOUTH); addBttn.addActionListener(this); deleteBttn.addActionListener(this); updateBttn.addActionListener(this); findBttn.addActionListener(this); } public static void main(String[] args) { try { UIManager.setLookAndFeel("com.sun.java.swing.plaf.motif.MotifLookAndFeel"); } catch(Exception ex) { JOptionPane.showMessageDialog(null,"The UIManager couldn't setthe motif look and feel","Error",JOptionPane.ERROR_MESSAGE); } PhoneBook f = new PhoneBook(); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); f.setSize(300,140); f.setLocation(350,350); f.setVisible(true); } public void actionPerformed(ActionEvent e) { index = 0; boolean nameAdded; boolean phoneAdded; name = new String (nameField.getText()); phone = new String (phoneField.getText()); nameList = new ArrayList(index); phoneList = new ArrayList(index); if(e.getSource() == addBttn) { nameAdded = nameList.add(name); phoneAdded = phoneList.add(phone); if(nameList.contains(name)) { JOptionPane.showMessageDialog(null,"The name "+name+" and the phone number "+phone+" was successfully added","Info",JOptionPane.INFORMATION_MESSAGE); clear(); } } if(e.getSource() == deleteBttn) { if(validName()) { int phoneIndex = getIndex(); nameList.remove(phoneIndex); phoneList.remove(phoneIndex); } { JOptionPane.showMessageDialog(null,"The name "+nameField.getText()+" was successfully removed ","Info",JOptionPane.INFORMATION_MESSAGE); clear(); } } if(e.getSource() == updateBttn) { } if(e.getSource() == findBttn) { int findIndex = getIndex(); phoneField.setText((String)phoneList.get(findIndex)); } } public void clear() { nameField.setText(""); phoneField.setText(""); nameField.requestFocus(); } public int getIndex() { int index=0; if(validName()) { for(int i = 0;i { if(nameField.getText().equals(nameList.get(i))) index = i; } } else { JOptionPane.showMessageDialog(null,"The list does not contain the name "+nameField.getText(),"Info",JOptionPane.INFORMATION_MESSAGE);
} return index; }
public boolean validName()
{
boolean validName = false;
if(nameList.contains(nameField.getText()))
validName = true;
return validName;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
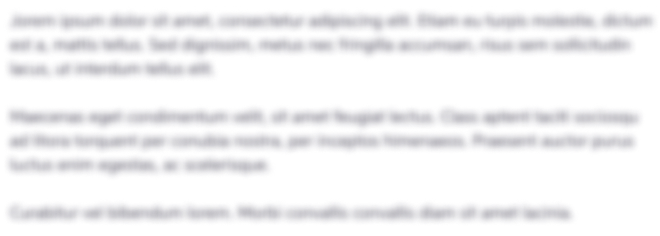
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started