Question
Hi! Can you help me fix my code for it to work? Thank you! #include using namespace std; class Coins { private: int quarters; int
Hi! Can you help me fix my code for it to work? Thank you!
#include
using namespace std;
class Coins { private: int quarters; int dimes; int nickels; int pennies; public: Coins(int quarters, int dimes, int nickels, int pennies) { quarters = 0; dimes = 0; nickels = 0; pennies = 0; } };
void ShowMenu() { cout << "---------ATM--------" << endl; cout << "D - Deposit Coins in bank" << endl; cout << "W - Withdraw coins from bank" << endl; cout << "S- Show account balance" << endl; cout << "Q - Quit" << endl; cout << "--------------------" << endl; cout << "Enter your selection: "; }
void EnterCoins() { int q; int d; int n, int p; cout << "Enter number of Quarters: "; cin >> q; cout << "Enter number of Dimes: "; cin >> d; cout << "Enter number of Nickels: "; cin >> n; cout << "Enter Nickels of Pennies: "; cin >> p; }
void ShowAccountBalance() { cout << "=========Account=========" << endl; cout << "Quarters:" << quarters << endl; cout << "Dimes:" << dimes << endl; cout << "Nickels:" << nickels << endl; cout << "Pennies:" << pennies << endl; cout << "=========================" << endl; }
void DepositCoins(Coins amount) { quarters = quarters + amount.quarters; dimes = dimes + amount.dimes; nickels = nickels + amount.nickels; pennies = pennies + amount.pennies; }
void WithdrawCoins(Coins amount) { quarters = quarters - amount.quarters; dimes = dimes - amount.dimes; nickels = nickels - amount.nickels; pennies = pennies - amount.pennies;
}
bool CoinsAvailable(Coins amount) { if (quarters >= amount.quarters && dimes >=amount.dimes && nickels >=amount.nickels && pennies >= amount.pennies) { return true; } else { return false; }
}
int main() { int quarters; int dimes; int nickels; int pennies; Coins bank(5,10,15,20); while(true) { bank.ShowMenu(); char choice; cin >> choice; if (choice == "D" || choice == 'd') { bank.EnterCoins(); } else if ( choice == 'W' || choice == 'w') { bank.WithdrawCoins(); } else if ( choice == 'S' || choice == 's') { bank.ShowAccountBalance(); } else if ( choice == 'Q' || choice == 'q') { return 0; } else { cout << "Invalide Choice!" << endl; return ShowMenu(); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
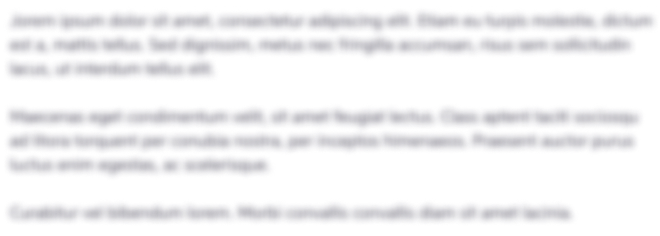
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started