Question
Hi! Can you help me write the code for this problem. Please write as basic as you can. Thank you! Splitting Strings The goal of
Hi! Can you help me write the code for this problem. Please write as basic as you can. Thank you!
Splitting Strings The goal of this assignment is to show how pointers can be used to efficiently manipulate strings. You will demonstrate this by splitting one string into two without copying the original strings contents. Objective Use pointers to split a string containing two words into two strings, without moving or copying the characters in the original array. Your program will do the following: 1. At startup, program creates a character array of size 50 called buf. 2. The program asks the user to enter two words. The two words are entered on a single line and are separated by a space. Hint: Use cin.getline(char[], int). 3. A function you will write, called FindSpace, is called. It takes in a char array and looks for the space between the two words. When the space is found, a pointer is returned that points to the address of the array slot containing the space. 4. Take the pointer returned by FindSpace and assign it to a pointer variable. Use the pointer variable to assign the value of the null terminator character \0 to that memory location. 5. Add one to the pointer so that it now points to the address containing the first character of the second word. 6. Use cout to display the contents of buf. This displays the first word. 7. Use cout to display the contents of your pointer variable. This displays the second word. Refer to the Expected Output for details. Requirements 1. Define a function called FindSpace. It takes in a single parameter, an array of characters that stores a C-string. It returns a char* that points to the index/slot of the array containing a space character. 2. If no spaces are found, the FindSpace function returns a valid pointer address to the array slot containing the \0. This results in the first word being the entire original string and the second work being an empty string. 3. The contents of buf may not be copied or moved, but you will need to overwrite the space character with the null terminator character \0 to complete the assignment. 4. All code should comply with good code style practices.
Useful Information
1. When the user enters two words, they are stored into buf as a C-string. In memory it looks like this:
H e l l o W o r l d \0
[0] [1] [2] [3] [4] [5] [6] [7] [8] [9] [10] [11]
buf == &[0] // Recall that the array variable is a pointer to the first slot of the array.
2. When FindSpace is called and buf is passed to it as the parameter, it returns a pointer that points to the address of the space character. In this case, it is slot 5. char* ptr = FindSpace (buf); ptr == &buf[5]; // ptr has address at buf slot 5. 3. Change ptr value to \0 to replace space character at buf[5]. After the change, buf looks like this in memory. Observe that buf now has two null terminator characters. Basically, the character array now contains two C-strings.
H e l l o \0 W o r l d \0
[0] [1] [2] [3] [4] [5] [6] [7] [8] [9] [10] [11]
4. Add one to ptrs address so that ptr now points to &buf[6]. ptr can now be treated as if it is the beginning of C-string character array. cout already knows how to treat character arrays that end with \0.
Expected Output
Enter two words: Hello World
1st word: Hello
2nd word: World
Step by Step Solution
There are 3 Steps involved in it
Step: 1
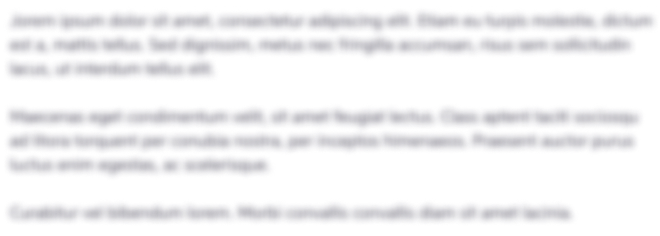
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started