Question
*Hi, could I have help with the following question? The completed program should follow the given instructions and Program Template as closely as possible, and
*Hi, could I have help with the following question? The completed program should follow the given instructions and Program Template as closely as possible, and produce an output exactly like the Sample Output*
In this assignment, you will be practicing the concepts of inheritance: extending a class, subclass, or superclass. You will also be practicing calling methods from the superclass in the subclass.
There are 3 classes you should implement:
1. Document class (superclass, general class)
2. Email class (subclass, more specialized class)
3. Driver/tester class
Write a detailed explanation in block comments for each class and every method.
Document class
Document |
-String content |
+Document (String text) +getContent(): String +setContent(String text): void +toString() : String +getLContentLength(): int +contains(String keyword) : boolean +equals(Document other) |
Document class Methods
The following methods must be implemented. Some of them might not be called.
public Document (String text): this constructor accepts a parameter of type String and initializes the instance variable content to this parameter
public String getContent(): this method returns the instance variable content
setContent(String newContent(): This method changes the instance variable content to the new content
toString() : This method creates a String representing an object of Document and then returns it.
public int getContentLength(): this method returns the length of the String content and returns it. to find the length of a String the length method from the String class should be used. content.length() will give you the number of the letters in the variable content.
public Boolean contains (String keyword): this method returns true if the instance variable content contains the keyword. you can use the contains method from the string class. content.contains(keyword) should be used in this method
public Boolean equals (Document other): this method compares two objects of type Document. returns true if the two Document objects are the same and returns false otherwise. The code that can be used is: this.content.equalsIgnoreCase(other.content)
Email class (this class extends the Document class)
-String sender -String recipient -Date date //date of the email -String subject // email subject -String cc //list of the people to be copied -boolean iSent //specifies if the email has been sent |
+Email (String text, String sender, String recipient, String subject, String cc) +public send (): void +public forward (String recipient, String cc) +public getIsSent(): Boolean +public getSender(): String +public getRecipient(): String +public getSubject(): String +public getCC(): String +public getDate(): Date +public setSender(String sender): void +public setRecipient(String recipient) +public setSubject(String subject): void +public setCC(String cc): void +public toString(): String +public modifyContent(String newText): void +public forward(String rec, String sender String cc): Email +public toString(): String |
Email class Methods
The following methods must be implemented. Some of them might not be called.
public Email (String text, String sender, String recipient, String subject, String cc): This constructor initializes the instance variables to the given parameters. To set the instance variable "date" use the code: date = new Date().
public void send(): This method sets the instance variable "isSent" to true, meaning that this email has been sent.
public boolean getSent(): Returns the instance variable "isSent."
public String getSender(): Returns the instance variable "sender."
public String getRecipiant(): Returns the instance variables "recipient."
public String getSubject(): Returns the instance variable "subject."
public String getCC(): Returns the instance variable "cc."
public Date Date(): Returns the instance variable "date."
public void setSender(String s): This method checks the instance variable "isSent." If it is false, that means the email hasn't been sent yet and the sender can still be modified. If it's true, the sender cannot be modified because the email was already sent. The following code can be used:
public void setSender(String s){ if(isSent == false) sender = s; else System.out.println(This email has been sent and cannot be modified);
public void setRecipient(String r): This method checks the instance variable "isSent." If it is false, that means the email hasn't been sent yet and the recepient can still be modified. If it's true, the recepient cannot be modified because the email was already sent.
public void setSubject(String s): This method checks the instance variable "isSent." If it is false, that means the email hasn't been sent yet and the subject can still be modified. If it's true, the subject cannot be modified because the email was already sent.
public void setCC(String c): This method checks the instance variable "isSent." If it is false, that means the email hasn't been sent yet and the cc can still be modified. If it's true, the cc cannot be modified because the email was already sent.
public String toString(): This method creates a String representing the Email object. The String must include the sender, recipient, subject, date and the content of the email. Note that the instance variable content is declared in the Document class, and you need to call the toString method from the Document class: super.toString() should be used in this method
public void modifyContent (String s): this method must check the instance variable isSent. if it is false that means the email has not been sent yet and the content can be modified. Otherwise the cc cannot be modified since the email has already been sent. Since instance variable content is declared in the Document class (Super class), therefore to modify the content this code is needed: super.setContent(s)
public Email forward (String rec, String sender String cc): This method is going to forward an email that was previously sent to the new recipients and cc. This method must create a new Email object. Use the following code:
Email f = new Email (this.getText(), sender, rec, this.subject, cc);
f.date = new Date();
f.isSent = true;
return f;
Driver class
Some of the code for this class has been provided. After you are done implementing the Document and Email classes, add the following to the Driver class.
This class should utilize all the methods implemented in the Email class.
create two objects of the Email class called e1 and e2
send the email e1
call the setter methods on the email e1
forward the email e1
display the email e1
forward the email e1
call the setter methods on the email e2
modify the content on the email e2
call the method getDocumentLength on the email e2
call the method contains on the email e2 with the word of your choice.
send the email e2
display the email e2
Sample Output:
Program Template:
Sender: John Smith Recipient: Alex CC: Subject: Meeting Date: Wed Sep 1515:37:48 PDT 2021 Content: Hello everyone, we will have a meeting tomorrow at 10 The word tomorrow was found in the email The content of this email is: Hello everyone, we will have a meeting tomorrow at 10 This email has been sent and the content cannot be modified This email has been sent and the recipient cannot be changed forwarded message Sender: John Recipient: Alex CC: Maria Subject: Meeting Date: Wed Sep 15 is: 157:48 PDT 2021 Content: Hello everyone, we will have a meeting tomorrow at 10 The number of the letters in the email is: 53 mport java.util.*; ublic class DocumnetYourLastName //
Step by Step Solution
There are 3 Steps involved in it
Step: 1
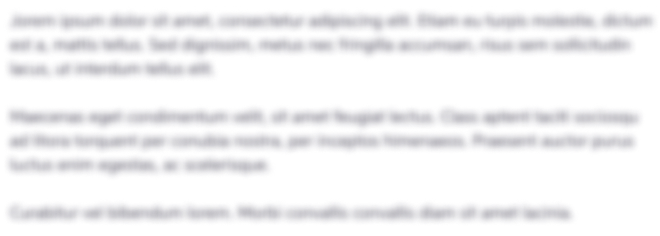
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started