Question
Hi guys. please help me modify this code to avoid plagiarism. If you just simply change the variable name only, i will downvote you. This
Hi guys. please help me modify this code to avoid plagiarism. If you just simply change the variable name only, i will downvote you. This code is about binary search tree.
Code:
import java.util.*; class BstNode{ int value; BstNode left,right; public BstNode(int value){ this.value = value; left = right = null; } }
class BST { // member variable BstNode root; // Constructor BST(){ root = null; } // getter for root node public BstNode getRoot(){ return root; } // method to add nodes to bst, iterative version public void add(int inputValue){ // creating a new temp node BstNode tempNode = new BstNode(inputValue); // pointer to traverse the bst to find the right place for the new node BstNode cur = root; // parent of tempNode will be prev node BstNode prev = null; // traversing through bst while (cur != null) { // marking current node to prev node prev = cur; // if incoming value is less than current value, // then place of tempNode will be in left subtree else right subtree if(inputValue <= cur.value) cur = cur.left; else cur = cur.right; } // in case root is null then prev will also be null if (prev == null) root = tempNode; // test to assign tempNode to prev's left child or right child else if (inputValue <= prev.value) prev.left = tempNode; else prev.right = tempNode; // printing about tempNode that is added to the bst System.out.println("New Node added with value: "+inputValue); } // inorder: left > root > right public void inorderTraversal(BstNode rootNode){ // base case check if (rootNode != null){ // left child inorderTraversal(rootNode.left); // printing current node System.out.print(rootNode.value + " "); // right child inorderTraversal(rootNode.right); } } // postorder: left > right > root public void postOrderTraversal(BstNode rootNode){ if (rootNode != null){ // left child postOrderTraversal(rootNode.left); // right child postOrderTraversal(rootNode.right); // printing current node System.out.print(rootNode.value + " "); } } // preorder: root > left > right public void preOrderTraversal(BstNode rootNode){ if (rootNode != null){ // printing current node System.out.print(rootNode.value + " "); // left child preOrderTraversal(rootNode.left); // right child preOrderTraversal(rootNode.right); } } // levelorder: level by level public void levelOrderTraversal(){ // declaring queue LinkedList
/* 55 / \ 40 60 / \ / \ 10 45 58 80 */ public class Main { public static void main(String[] args) { // creating the above sample tree BST bst = new BST(); // adding nodes to bst bst.add(55); bst.add(40); bst.add(60); bst.add(80); bst.add(58); bst.add(10); bst.add(45);
// printing inorder way System.out.println(" Inorder traversal: "); bst.inorderTraversal(bst.getRoot()); // print preorder way System.out.println(" Preorder traversal: "); bst.preOrderTraversal(bst.getRoot()); // print postorder way System.out.println(" Postorder traversal: "); bst.postOrderTraversal(bst.getRoot()); // print levelorder way System.out.println(" LevelOrder traversal: "); bst.levelOrderTraversal(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
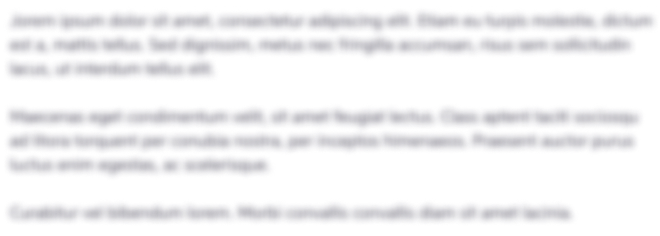
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started