Question
Hi, how can I convert the data saved and restore it from a .txt file instead by a binary file from pointers? I need it
Hi, how can I convert the data saved and restore it from a .txt file instead by a binary file from pointers? I need it as .txt file because it shows strange characters in the file when I try to open normally with a text editor.
Code:
#include
using std::cout;
using std::cin;
using std::endl;
using std::ios;
#include
using std::fstream;
#include
//struct definition
struct animal {
char text[100];
animal* yes;
animal* no;
};
//function templates
void deallocateTree(animal*);
void saveTree(animal*, fstream&);
void restoreTree(animal*, fstream&);
int main() {
animal* root = new animal;
strcpy(root->text, "Elephant");
root->yes = 0;
root->no = 0;
// restore tree from a disk file
fstream fin;
fin.open("Animal.dat", ios::in|ios::binary);
restoreTree(root, fin); // create an empty root node before calling this
fin.close();
while(true) {
char buf[100];
cout << "Think of an animal and I'll try to guess it! Okay? Y/N: ";
cin >> buf;
if (toupper(buf[0]) == 'N')
break;
animal* p = root;
while(p) {
if(p->yes == 0) {
bool correctGuess;
while (true) {
cout << p->text << " Y/N: ";
cin >> buf;
if (toupper(buf[0]) == 'Y') {
cout << "Damn, I'm good!" << endl;
correctGuess = true;
break;
}
else if (toupper(buf[0]) == 'N') {
correctGuess = false;
break;
}
else if (toupper(buf[0]) == 'E') {
cin.ignore(1000,10);
cout << "New answer: ";
cin.getline(p->text,100);
}
}
if (correctGuess == true)
break;
char a[100], q[100], yN[100];
cout << "What animal are you thinking of? ";
cin >> a;
cin.ignore(1000,10);
cout << "What yes/no question differentiates " << p->text << " from " << a << "? ";
cin.getline(q, 100);
cout << "What response is correct for " << a << " -- Y/N? ";
cin >> yN;
cin.ignore(1000,10);
animal* y = new animal;
animal* n = new animal;
if (toupper(yN[0]) == 'Y') {
strcpy(y->text, a);
strcpy(n->text, p->text);
}
else if (toupper(yN[0]) == 'N') {
strcpy(n->text, a);
strcpy(y->text, p->text);
}
strcpy(p->text, q);
y->yes = 0;
n->yes = 0;
y->no = 0;
n->no = 0;
p->yes = y;
p->no = n;
break;
}
else {
while (true) {
char yN[100];
cout << p->text << " Y/N ";
cin >> yN;
cin.ignore(1000,10);
if (toupper(yN[0]) == 'Y') {
p = p->yes;
break;
}
else if (toupper(yN[0]) == 'N') {
p = p->no;
break;
}
else if (toupper(yN[0]) == 'E') {
cout << "New question: ";
cin.getline(p->text,100);
}
else if (toupper(yN[0]) == 'D') {
deallocateTree(p->yes);
deallocateTree(p->no);
strcpy(p->text, "Elephant");
p->yes = 0;
p->no = 0;
break;
}
}
}
}
}
// save tree to a disk file
fstream fout;
fout.open("Animal.dat", ios::out|ios::binary);
saveTree(root, fout);
fout.close();
deallocateTree(root);
}
void deallocateTree(animal* a) {
if (!a) return;
deallocateTree(a->yes);
deallocateTree(a->no);
delete a;
}
// function definitions -- save and restore
void saveTree(animal* a, fstream& out) { // saves tree to disk file
if (a) {
out.write((char*)a, sizeof(animal));
saveTree(a->yes, out);
saveTree(a->no, out);
}
}
void restoreTree(animal* a, fstream& in) { // loads tree from disk file
in.read((char*)a, sizeof(animal));
if(a->yes) {
restoreTree(a->yes = new animal, in);
restoreTree(a->no = new animal, in);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
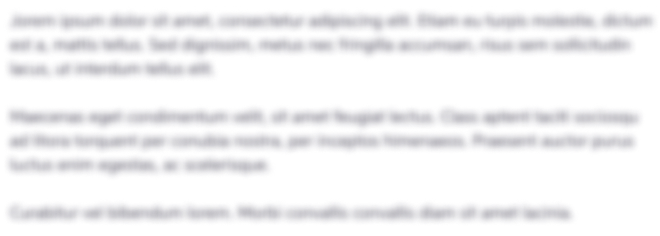
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started