Question
Hi, I am taking a Data Structures and Algorithms class in Java this semester. We were just introduced to Stacks and Queues abd there is
Hi,
I am taking a Data Structures and Algorithms class in Java this semester. We were just introduced to Stacks and Queues abd there is one part of the assignment that i am confused with. I have attached the description of the problem and notes from the instructor along with the code for the Deque and QueueX class.
Thanks!
Assignment:
Note from Professor:
When you define class QueueD and class StackD, you only need a Deque as data member to store data in queue or stack. You do not need to define other array as data member because Deque itself has an array for storing data. Definition looks like:
public class QueueD {
private Deque dq;
//constructor and other methods
}
Then, you will use dq to call Deque's method to implement QueueD's insert/remove and StackD's push/pop.
QueueX Class:
public class QueueX
private int maxSize;
private T[] queArray;
private int front; //front of the queue
private int rear; //rear of the queue
private int nItems;
//--------------------------------------------------------------
public QueueX(int s) // constructor
{
maxSize = s;
queArray = (T[])new Object[maxSize];
front = 0;
rear = -1;
nItems = 0;
}
//--------------------------------------------------------------
public void insert(T item) // put item at rear of queue
{
if(rear == maxSize-1) // deal with wraparound
rear = -1;
queArray[++rear] = item; // increment rear and insert
nItems++; // one more item
}
//--------------------------------------------------------------
public T remove() // take item from front of queue
{
T temp = queArray[front++]; // get value and increment front
if(front == maxSize) // deal with wraparound
front = 0;
nItems--; // one less item
return temp;
}
//--------------------------------------------------------------
public T peek() // peek at front of queue
{
return queArray[front];
}
//--------------------------------------------------------------
public boolean isEmpty() // true if queue is empty
{
return (nItems==0);
}
//--------------------------------------------------------------
public boolean isFull() // true if queue is full
{
return (nItems==maxSize);
}
//--------------------------------------------------------------
public int size() // number of items in queue
{
return nItems;
}
//--------------------------------------------------------------
} // end class QueueX
Deque class:
public class Deque { private int maxSize; private long[] dekArray; private int left; private int right; private int nItems;
//-------------------------------------------------------------- public Deque(int s) // constructor { maxSize = s; dekArray = new long[maxSize]; int center = maxSize/2 - 1; left = center+1; // left and right right = center; // start out "crossed" nItems = 0; } // other methods // Method to check if the queue is empty public boolean isEmpty(){ if(nItems == 0) return true; else return false; } // Method to check if the queue is full public boolean isFull(){ if(nItems == maxSize) return true; else return false; } // Method to insert to left public void insertLeft(long data){ if(isFull()||left == 0) System.out.println("Left Array is full"); else{ left--; dekArray[left] = data; nItems++; } } // Method to insert to right public void insertRight(long data){ if(isFull()||right == maxSize-1) System.out.println("Right Array is full"); else{ right++; dekArray[right] = data; nItems++; } } // Method to remove from left public long removeLeft(){ long data = -1; if(isEmpty()){ return -1; } else if(left t a queue class qusue Here you are 2. In the lectures (textbook), we used an array to i asked to implement a queue class gueueD that is based on the Deque class defined in Problem 1. It means you need to use composition and make ueueD has-a Deque. This queue class should have the same methods and capabilities as the Queuex class. Write a driver class eueDApp to test class Queuel t a queue class qusue Here you are 2. In the lectures (textbook), we used an array to i asked to implement a queue class gueueD that is based on the Deque class defined in Problem 1. It means you need to use composition and make ueueD has-a Deque. This queue class should have the same methods and capabilities as the Queuex class. Write a driver class eueDApp to test class Queuel
Step by Step Solution
There are 3 Steps involved in it
Step: 1
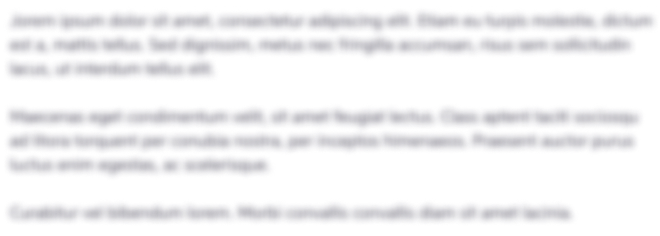
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started