Answered step by step
Verified Expert Solution
Question
1 Approved Answer
hi i have a typescript program that gives the user a list of numbers then the user inputs the number that is trying to find
hi i have a typescript program that gives the user a list of numbers then the user inputs the number that is trying to find and the program is going to tell the user the location of the number , the program works fine but the only problem is the try and catch when i input a string it should say "input an integer" but it doenot do that how can i fix it?
code:
import * as readline from 'readline' | |
function compareNumbers (a: number, b: number) { | |
// https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/sort | |
return a - b | |
} | |
function getRandomInt (min: number, max: number) { | |
// https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Math/random | |
min = Math.ceil(min) | |
max = Math.floor(max) | |
return Math.floor(Math.random() * (max - min) + min) | |
} | |
function binarySearch (array: number[], searchNum: number, | |
lowIndex: number, highIndex: number) { | |
// this function is a binary search engine. | |
if (lowIndex > highIndex) { | |
return -1 | |
} else { | |
const medium: number = Math.round((highIndex + lowIndex) / 2) | |
let returnValue: number | |
if (array[medium] > searchNum) { | |
returnValue = binarySearch(array, searchNum, | |
lowIndex, medium - 1) | |
return returnValue | |
} else if (array[medium] < searchNum) { | |
returnValue = binarySearch(array, searchNum, | |
medium + 1, highIndex) | |
return returnValue | |
} else { | |
return medium | |
} | |
} | |
} | |
const generatedNum :number[] = new Array(250) | |
for (let i: number = 0; i < generatedNum.length; i++) { | |
generatedNum[i] = getRandomInt(0, 1000) | |
} | |
generatedNum.sort(compareNumbers) | |
console.log(generatedNum) | |
const rl = readline.createInterface({ | |
input: process.stdin, | |
output: process.stdout | |
}) | |
rl.question( | |
'What number are you searching for in the array (integer between 0 and 999): ' | |
, function (input) { | |
try { | |
const answer: number = binarySearch(generatedNum, parseInt(input), 0, 250) | |
if (answer === -1) { | |
console.log('The number is not present in the array.') | |
} else { | |
console.log('The number is present at index ' + answer + '.') | |
} | |
} catch (exeption) { | |
console.log('please insert an integer.') | |
} | |
rl.close() | |
console.log(' Done.') | |
}) |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
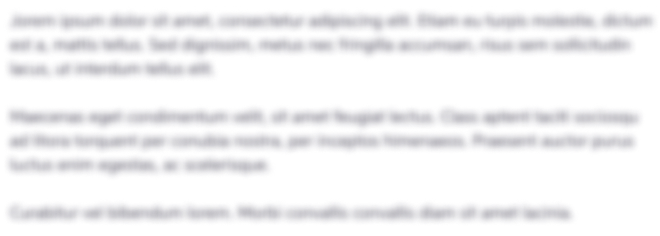
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started