Answered step by step
Verified Expert Solution
Question
1 Approved Answer
hi , i have to do matrix multiplication in scala, but i have no previous experience in scala. Can you please help me with this?
hi i have to do matrix multiplication in scala, but i have no previous experience in scala. Can you please help me with this?
Part Matrix Multiplicaiton of Coordinate Matrices
Small Matrices
Define two small matrices M and N
val M ArrayArray Array
val N ArrayArray Array
Convert the small matrices to RDDs
val MRDDSmall scparallelizeMzipWithIndex.flatMap case row i row.zipWithIndex.map case value jitoInt, jtoInt value
val NRDDSmall scparallelizeNzipWithIndex.flatMap case row i row.zipWithIndex.map case value jitoInt, jtoInt value
TODO Function to perform matrix multiplication of RDDs
def COOMatrixMultiply M: RDDIntIntDouble N: RDDIntIntDouble: RDDIntIntDouble
Your Code
val RRDDSmall COOMatrixMultiplyMRDDSmall, NRDDSmall
RRDDSmall.collect.foreachprintln
Validate the result
def manualMatrixMultiplyM: ArrayArrayDouble N: ArrayArrayDouble: ArrayArrayDouble
val result Array.ofDimDoubleMlength, Nlength
for
i Mindices
j Nindices
k Mindices
resultij Mik Nkj
result
val resultmanual RRDDSmall.collectmap case i j valuei j valuesortBy case i ji j
val resultArray Array.ofDimDoubleMlength, Nlength
for i j value resultmanual
resultArrayij value
val expectedResult manualMatrixMultiplyM N
assertresultArraydeep expectedResult.deep, "Result mismatch"
Large Datasets
Generate Random Coordinate Matrices
import scala.util.Random
Function to generate random coordinate matrices for large datasets
def randomCOOMatrix n: Int, m: Int : RDDIntIntDouble
val max
val l Random.shuffle until ntoList
val r Random.shuffle until mtoList
scparallelizel
flatMap i val rand new Random
rmap j itoInt,jtoIntrand.nextDoublemax
Set the dimensions of matrices M and N as x
val n
val m
val MRDDLarge randomCOOMatrixnm
val NRDDLarge randomCOOMatrixmn
TODO Set the Dimensions of the Matrices
Set the dimensions of matrices M and N as x
val n
val m
val MRDDLarge randomCOOMatrixnm
val NRDDLarge randomCOOMatrixmn
Perform Multiplication
val RRDDLarge COOMatrixMultiplyMRDDLarge, NRDDLarge
Notice the time it takes to multiply two x matrices
RRDDLarge.count
Part Matrix Multiplication of Block Matrices
Small Block Matrix Multiplication
import org.apache.spark.mllib.linalg.distributed.
Convert small coordinate matrices to block matrices
val MBlockMatrix new CoordinateMatrixMRDDSmall.map case i j value MatrixEntryi j valuetoBlockMatrix
val NBlockMatrix new CoordinateMatrixNRDDSmall.map case i j value MatrixEntryi j valuetoBlockMatrix
MBlockMatrix.blocks.collect.foreachprintln
NBlockMatrix.blocks.collect.foreachprintln
Perform multiplication of small block matrices
val RBlockSmall MBlockMatrix.multiplyNBlockMatrix
RBlockSmall.blocks.collect.foreachprintln
Large Block Multiplication
TODO Set the Block Size
set the number of rows and columns per block to be
val rb
val cb
Convert large coordinate matrices to block matrices where each block has rows and columns
val MBlockMatrixLarge new CoordinateMatrixMRDDLarge.map case i j value MatrixEntryi j valuetoBlockMatrixrbcb
val NBlockMatrixLarge new CoordinateMatrixNRDDLarge.map case i j value MatrixEntryi j valuetoBlockMatrixcbcb
MBlockMatrixLarge.validate
NBlockMatrixLarge.validate
assertMBlockMatrixLarge.numRowBlocks "Result mismatch"
assertMBlockMatrixLarge.numColBlocks "Result mismatch"
assertNBlockMatrixLarge.numRowBlocks "Result mismatch"
assertNBlockMatrixLarge.numColBlocks "Result mismatch"
val RBlockLarge MBlockMatrixLarge.multiplyNBlockMatrixLarge
Notice the time it takes to multiply two x block matrices
RBlockLarge.blocks.count
Bonus: Provide a Faster Implementation of Matrix Multiplication than Block Matrix Multiplication! points
Please help me with this :
Step by Step Solution
There are 3 Steps involved in it
Step: 1
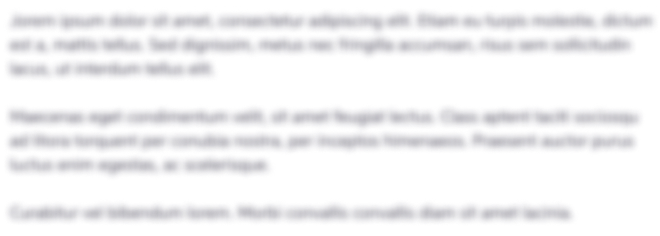
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started