Question
Hi I just wanted to know how I would go about doing this. It basically asks for you to search for a movie title among
Hi I just wanted to know how I would go about doing this. It basically asks for you to search for a movie title among either of two excel files (the .jpeg files below with the titles are pictures of the excel files).The requirements for the project are in the image files for the project guidelines below starting with the page titled: 'ECE 242: Data Structures and Algorithms- Fall 2017 Project 5: FunWithMovies (Advanced Sorting and HashTable)'.
class App1{
public static void main(String args[]){
// declare instance of EasyIn class
EasyIn easy = new EasyIn();
// other variables
MovieArray dvdlist;
String filename;
int maxsize;
long startTime,endTime;
System.out.println();
System.out.println("Welcome to Movie App 1");
System.out.println("====================== ");
System.out.println();
//////////////////// Load movie database from command line
System.out.println("Enter the name of the movie database:");
filename = easy.readString();
System.out.println("Enter the max size of the array to store the database:");
maxsize = easy.readInt();
dvdlist = new MovieArray(filename,maxsize); // initialize database
System.out.println("Size of database "+dvdlist.getSize());
////////////// Sorting the database
System.out.println(" Which advanced sorting algo? (0: Insertionsort; 1:Shellsort, 2:Mergesort, 3:Quicksort, 4:Heapsort)");
int sort=easy.readInt(); // ask before starting counting time
startTime = System.currentTimeMillis(); // capture time
///////////// Select the right option
switch(sort)
{
case 0: // implement insertionsort
dvdlist.insertionSort();
break;
case 1: // implement shellsort
dvdlist.shellSort();
break;
case 2: // implement mergesort
dvdlist.mergeSort();
break;
case 3: // implement quicksort
dvdlist.quickSort();
break;
case 4: // implement heapsort
dvdlist.heapSort();
break;
default:
System.out.println("Selection Invalid!");
System.exit(0);
}
endTime = System.currentTimeMillis(); // capture time
///////////// Save sorted Database
dvdlist.save("dvdlist.csv-sorted");
System.out.println(" OK database sorted in "+(endTime-startTime)+"ms and save in file '"+filename+"-sorted'");
System.out.println(" Goodbye!");
}
}
class App2{
public static void main(String args[]){
// declare instance of EasyIn class
EasyIn easy = new EasyIn();
// other variables
MovieArray dvdlist;
String filename;
int maxsize;
long startTime,endTime;
System.out.println();
System.out.println("Welcome to Movie App 2");
System.out.println("====================== ");
System.out.println();
//////////////////// Load movie database from command line
System.out.println("Enter the name of the movie *sorted* database:");
filename = easy.readString();
System.out.println("Enter the max size of the array to store the database:");
maxsize = easy.readInt();
dvdlist = new MovieArray(filename,maxsize); // initialize database
System.out.println("Size of database "+dvdlist.getSize());
////////////// Searching the database
System.out.println("How many random titles you would like to search?:");
int nrnd=easy.readInt();
startTime = System.currentTimeMillis(); // capture time
int totalStep=0;
for (int i=0;i
totalStep=totalStep+dvdlist.getStep();
endTime = System.currentTimeMillis(); // capture time
System.out.println(" Ok search done in "+(endTime-startTime)+"ms");
System.out.println("Total number of search step is "+totalStep);
System.out.println("Average number of search step is "+totalSteprnd);
System.out.println(" Goodbye!");
}
}
class App3{
public static void main(String args[]){
// declare instance of EasyIn class
EasyIn easy = new EasyIn();
// other variables
MovieArray dvdlist;
String filename;
int maxsize;
long startTime,endTime;
System.out.println();
System.out.println("Welcome to Movie App 3");
System.out.println("====================== ");
System.out.println();
//////////////////// Load movie database from command line
System.out.println("Enter the name of the movie database:");
filename = easy.readString();
System.out.println("Enter the max size of the array to store the database:");
maxsize = easy.readInt();
dvdlist = new MovieArray(filename,maxsize); // initialize database
System.out.println(" Size of database "+dvdlist.getSize());
////////////// create the hash table
HashTable htab=new HashTable(dvdlist);
System.out.println(" OK HashTable created, maxSize is "+htab.getMaxSize());
System.out.println("it found "+htab.getCol()+" collisions"+" with "+htab.getMaxProbe()+" maximum probe length");
if (htab.getMaxSize()
htab.saveHashTable(filename+"-hash");
System.out.println("HashTable save in file '"+filename+"-hash'");
}
////////////// Search the database
/// Reload the array database (may have been erased while creating the hashtable)
dvdlist = new MovieArray(filename,maxsize); // initialize database
System.out.println(" How many random titles you would like to search?:");
int nrnd=easy.readInt();
startTime = System.currentTimeMillis(); // capture time
int totalStep=0;
for (int i=0;i
totalStep=totalStep+htab.getStep();
endTime = System.currentTimeMillis(); // capture time
System.out.println(" Ok search done in "+(endTime-startTime)+"ms");
System.out.println("Total number of search step is "+totalStep);
System.out.println("Average number of search step is "+totalSteprnd);
System.out.println(" Goodbye!");
}
}
// Simple input from the keyboard for all primitive types. ver 1.0 // Copyright (c) Peter van der Linden, May 5 1997. // corrected error message 11/21/97 // // The creator of this software hereby gives you permission to: // 1. copy the work without changing it // 2. modify the work providing you send me a copy which I can // use in any way I want, including incorporating into this work. // 3. distribute copies of the work to the public by sale, lease, // rental, or lending // 4. perform the work // 5. display the work // 6. fold the work into a funny hat and wear it on your head. // // This is not thread safe, not high performance, and doesn't tell EOF. // It's intended for low-volume easy keyboard input. // An example of use is: // EasyIn easy = new EasyIn(); // int i = easy.readInt(); // reads an int from System.in // float f = easy.readFloat(); // reads a float from System.in
import java.io.*; import java.util.*;
class EasyIn { static InputStreamReader is = new InputStreamReader( System.in ); static BufferedReader br = new BufferedReader( is ); StringTokenizer st;
StringTokenizer getToken() throws IOException { String s = br.readLine(); return new StringTokenizer(s); }
boolean readBoolean() { try { st = getToken(); return new Boolean(st.nextToken()).booleanValue(); } catch (IOException ioe) { System.err.println("IO Exception in EasyIn.readBoolean"); return false; } }
byte readByte() { try { st = getToken(); return Byte.parseByte(st.nextToken()); } catch (IOException ioe) { System.err.println("IO Exception in EasyIn.readByte"); return 0; } }
short readShort() { try { st = getToken(); return Short.parseShort(st.nextToken()); } catch (IOException ioe) { System.err.println("IO Exception in EasyIn.readShort"); return 0; } }
int readInt() { try { st = getToken(); return Integer.parseInt(st.nextToken()); } catch (IOException ioe) { System.err.println("IO Exception in EasyIn.readInt"); return 0; } }
long readLong() { try { st = getToken(); return Long.parseLong(st.nextToken()); } catch (IOException ioe) { System.err.println("IO Exception in EasyIn.readLong"); return 0L; } }
float readFloat() { try { st = getToken(); return new Float(st.nextToken()).floatValue(); } catch (IOException ioe) { System.err.println("IO Exception in EasyIn.readFloat"); return 0.0F; } }
double readDouble() { try { st = getToken(); return new Double(st.nextToken()).doubleValue(); } catch (IOException ioe) { System.err.println("IO Exception in EasyIn.readDouble"); return 0.0; } }
char readChar() { try { String s = br.readLine(); return s.charAt(0); } catch (IOException ioe) { System.err.println("IO Exception in EasyIn.readChar"); return 0; } }
String readString() { try { return br.readLine(); } catch (IOException ioe) { System.err.println("IO Exception in EasyIn.readString"); return ""; } }
// This method is just here to test the class public static void main (String args[]){ EasyIn easy = new EasyIn();
System.out.print("enter char: "); System.out.println("You entered: " + easy.readChar() );
System.out.print("enter String: "); System.out.println("You entered: " + easy.readString() );
System.out.print("enter boolean: "); System.out.println("You entered: " + easy.readBoolean() );
//System.out.print("enter byte: "); //System.out.println("You entered: " + easy.readByte() );
System.out.print("enter short: "); System.out.println("You entered: " + easy.readShort() );
System.out.print("enter int: "); System.out.println("You entered: " + easy.readInt() );
System.out.print("enter long: "); System.out.println("You entered: " + easy.readLong() );
System.out.print("enter float: "); System.out.println("You entered: " + easy.readFloat() );
System.out.print("enter double: "); System.out.println("You entered: " + easy.readDouble() ); }
}
import java.io.*;
import java.util.*;
class HashTable{
/// TO COMPLETE
///////////////////////////////////////////////////////////////
/////////////// get the first prime number after number 'min'
///////////////// (from TextBook Chapter 11) /////////////////
//////////////////////////////////////////////////////////////
private int getPrime(int min)
// returns 1st prime > min
{
for(int j = min+1; true; j++)
// for all j > min
if( isPrime(j) )
// is j prime?
return j;
// yes, return it
}
// -------------------------------------------------------------
private boolean isPrime(int n)
// is n prime?
{
for(int j=2; (j*j
// for all j
if( n % j == 0)
// divides evenly by j?
return false;
// yes, so not prime
return true;
// no, so prime
}
}
class Movie{
// Common private variables
// Constructor: construct the Movie class with the 3 given parameters
/////////////////////////////////////////////////////////
// methods
/////////////////////////////////////////////////////////
}
import java.io.*; import java.util.*;
/////////////////////////////////////////////////////////////////////////// class MovieArray{
}
ECE 242: Data Structures and Algorithms- Fall 2017 Project 5: FunWithMovies (Advanced Sorting and HashTable) Due Date: Deadline: see website Description The goal of this project is to scarch a dvd movie database. You will use two implementations for the database: (i) a simple array of object Move) a hashtable. For the simple array, the search is performed using binary search so the database must first be sorted. You will be implementing all the various advanced sorting algorithms we have seen in class (ShellSort, MergeSort, Quicksort and Heapsort). For the hashtable, we propose to handle possible collisions using open addressing with double hashing The project includes multiple files: 1. a large database containing 300,547 random movies "dvdlist.csv" 2. a large database containing 300,547 sorted movies by title "dvdlist-sortedosv 3. Appi.java to App3.java: three application files (provided- do not change them) 4. Movie.java file containing the class object Movie (to complete) 5. MovieArray.java file containing the class object MovieArray with various methods (to complete) 6. HashTable.java file containing the class hashtable data structure with various methods to complete) 7. EasyIn.java file containing the class used to read user input from keyboard (provided) All the functionality of the application files (presented in detail below) should be successfully implemented to obtain full credit. You need to proceed step-by-step, application by application. A list of methods to implement is described at the end of each section Appl.java The application is loading a non-sorted list of dvd movies that will be stored in an array. The movie is defined by six different fields: (title,studio,price,rating,year genre) separated by the sign in the input file The user may select the maximum size of the array, and this size should be greater than the number of movies in the file in order to store the entire database. However, a small size means that only few lines of the file would be considered, which is also good for debugging. The user is then asked to select between five sorting algorithms: 0- insertion sort (simple algorithm implemented in project1), and four advanced sorting algorithms: 1-shellsort, 2-mergesort, 3-quicksort and 4-heapsort. The sorted database (sorted by movie title) will be saved in a file with the extension "-sorted" Let us look at the first 15 items of the file dvdlist.csv: Freaks (Mr. Fat-w Video/ On Demand DVD-R) IMr. Fat-W Videol14.99INRI 19321Horror Contract (2006/ Blu-ray) / Proposition (2005/ Blu-ray) lFirst Look 136.981RIVARI VAR Greatest Discoveries With Bill Nye: EvolutionlDiscovery Education l59.951NRIUNK ISpecial Interest Total Nonstop Action Wrestling Presents: TNA: Genesis 2011ITNAI 19.98INR 12011lSpecial Interest Forgotten Noir, Vol. 13: Kit Parker Double Features: Breakdown Eye WitnessIKit Parker Filmsl 14.99 INRIVARIDrama Isaku (NuTech Digital) #1: Rika l NuTech Digital 119.95 IMA 17119971 Anime ESPN: 199 LivesIESPN I 24.95 I NRI UNKI Sports Two Men In Town (2014/ Blu-ray)ICohen Media Groupl24.99 RI2014|Drama Nathalie Granger (2-Disc w Book) IBlaq Outl69.95INRI 1972 1Foreign It's Nice Up NorthlChicken UKI29.98 INR I 2006 IConedy ECE 242: Data Structures and Algorithms- Fall 2017 Project 5: FunWithMovies (Advanced Sorting and HashTable) Due Date: Deadline: see website Description The goal of this project is to scarch a dvd movie database. You will use two implementations for the database: (i) a simple array of object Move) a hashtable. For the simple array, the search is performed using binary search so the database must first be sorted. You will be implementing all the various advanced sorting algorithms we have seen in class (ShellSort, MergeSort, Quicksort and Heapsort). For the hashtable, we propose to handle possible collisions using open addressing with double hashing The project includes multiple files: 1. a large database containing 300,547 random movies "dvdlist.csv" 2. a large database containing 300,547 sorted movies by title "dvdlist-sortedosv 3. Appi.java to App3.java: three application files (provided- do not change them) 4. Movie.java file containing the class object Movie (to complete) 5. MovieArray.java file containing the class object MovieArray with various methods (to complete) 6. HashTable.java file containing the class hashtable data structure with various methods to complete) 7. EasyIn.java file containing the class used to read user input from keyboard (provided) All the functionality of the application files (presented in detail below) should be successfully implemented to obtain full credit. You need to proceed step-by-step, application by application. A list of methods to implement is described at the end of each section Appl.java The application is loading a non-sorted list of dvd movies that will be stored in an array. The movie is defined by six different fields: (title,studio,price,rating,year genre) separated by the sign in the input file The user may select the maximum size of the array, and this size should be greater than the number of movies in the file in order to store the entire database. However, a small size means that only few lines of the file would be considered, which is also good for debugging. The user is then asked to select between five sorting algorithms: 0- insertion sort (simple algorithm implemented in project1), and four advanced sorting algorithms: 1-shellsort, 2-mergesort, 3-quicksort and 4-heapsort. The sorted database (sorted by movie title) will be saved in a file with the extension "-sorted" Let us look at the first 15 items of the file dvdlist.csv: Freaks (Mr. Fat-w Video/ On Demand DVD-R) IMr. Fat-W Videol14.99INRI 19321Horror Contract (2006/ Blu-ray) / Proposition (2005/ Blu-ray) lFirst Look 136.981RIVARI VAR Greatest Discoveries With Bill Nye: EvolutionlDiscovery Education l59.951NRIUNK ISpecial Interest Total Nonstop Action Wrestling Presents: TNA: Genesis 2011ITNAI 19.98INR 12011lSpecial Interest Forgotten Noir, Vol. 13: Kit Parker Double Features: Breakdown Eye WitnessIKit Parker Filmsl 14.99 INRIVARIDrama Isaku (NuTech Digital) #1: Rika l NuTech Digital 119.95 IMA 17119971 Anime ESPN: 199 LivesIESPN I 24.95 I NRI UNKI Sports Two Men In Town (2014/ Blu-ray)ICohen Media Groupl24.99 RI2014|Drama Nathalie Granger (2-Disc w Book) IBlaq Outl69.95INRI 1972 1Foreign It's Nice Up NorthlChicken UKI29.98 INR I 2006 IConedyStep by Step Solution
There are 3 Steps involved in it
Step: 1
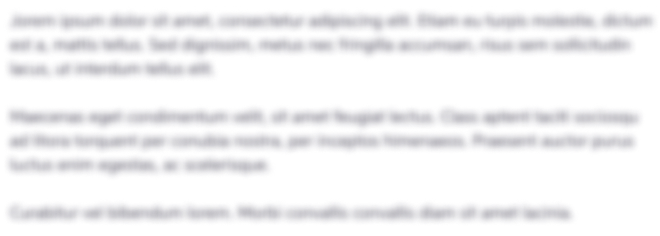
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started