Question
Hi I need a fix in my program. The program needs to finish after serving the customers from the queue list. Requeriments: Headers: DynamicArray.h #ifndef
Hi I need a fix in my program. The program needs to finish after serving the customers from the queue list.
Requeriments:
Headers:
DynamicArray.h
#ifndef DynamicArray_h
#define DynamicArray_h
#include
using namespace std;
template
class DynamicArray
{
V* values;
int cap;
V dummy;
public:
DynamicArray(int = 2);
DynamicArray(const DynamicArray&);
~DynamicArray() { delete[] values; }
int capacity() const { return cap; }
void capacity(int);
V operator[](int) const;
V& operator[](int);
DynamicArray& operator=(const DynamicArray&);
};
template
DynamicArray::DynamicArray(int cap)
{
this->cap = cap;
values = new V[cap];
for (int index = 0; index
values[index] = V();
}
}
template
V DynamicArray::operator[](int index) const
{
if (index = cap)
return V(); // a copy
return values[index]; // a copy
}
template
V& DynamicArray::operator[](int index)
{
if (index
return dummy; // a copy
}
else if (index >= cap) {
capacity(2 * index);
}
return values[index]; // a copy
}
template
void DynamicArray::capacity(int newCap) {
V* temp = new V[newCap];
// get the lesser of the new and old capacities
int limit = min(newCap, cap);
// copy the contents
for (int i = 0; i
temp[i] = values[i];
}
// set added values to their defaults
for (int i = limit; i
temp[i] = V();
}
// deallocate original array
delete[] values;
// switch newly allocated array into the object
values = temp;
// update the capacity
cap = newCap;
}
template
DynamicArray::DynamicArray(const DynamicArray& original)
{
cap = original.cap; // still copy
values = new V[cap]; // not copy, is new
for (int i = 0; i
values[i] = original.values[i];
}
}
template
DynamicArray& DynamicArray::operator=(const DynamicArray& original)
{
if (this != &original) //check if copy or not, better not be tho
{
// same as destructor
delete[] values;
// same as copy constructor
cap = original.cap;
values = new V[cap]; // not copy, is new
for (int i = 0; i
values[i] = original.values[i];
}
}
return *this; // return self reference
}
#endif
Queue.h
#ifndef QUEUE_QUEUE_H //checks if 'QUEUE_QUEUE_H' has been defined
#define QUEUE_QUEUE_H //define 'QUEUE_QUEUE_H' to use in the program
template //use a template class called 'V'
class Queue //class Queue
{
struct Node
{
V value;
Node* next;
};
Node* firstNode; //head pointer to the first node
int siz = 0; //variable necessary to trak the number of nodes
Node* lastNode; /ecssary to create a private data member
public:
Queue(); // may have a defaulted parameter, tested
void push(const V&);
V& front(); // return a mutable reference to the oldest node
V& back(); // return a mutable reference to the newest node
void pop(); // remove the oldest node
int size() const { return siz; } //return the values of the size
bool empty() const { return siz == 0; } /eccesary to empty the values
void clear(); /ecessary to clear the values
~Queue() { clear(); }
Queue& operator=(const Queue&);
Queue(const Queue&);
};
template
Queue::Queue()
{
lastNode = 0;
siz = 0;
}
//function to push the values from the stack
template
void Queue::push(const V& value)
{
Node* temp = new Node{ value }; // C++11
if (lastNode) lastNode->next = temp;
else firstNode = temp;
lastNode = temp;
++siz;
}
//template to put the values to the front of the stack
template
V& Queue::front()
{
return firstNode->value;
}
//function necessary to put the values on the back of the stack
template
V& Queue::back() {
return lastNode->value;
}
//function necessary to pop the values fromn the stack
template
void Queue::pop()
{
if (firstNode)
{
Node* p = firstNode;
firstNode = firstNode->next;
delete p;
--siz;
}
if (siz == 0)
{
lastNode = 0;
}
}
//function necessary to clear the values from the stack
template
void Queue::clear()
{
while (firstNode)
{
Node* p = firstNode;
firstNode = firstNode->next;
delete p;
--siz;
}
lastNode = 0;
}
//function necessary to copy the values
template
Queue::Queue(const Queue& original)
{
firstNode = 0;
lastNode = 0; // temporary tail
siz = original.siz;
for (Node* p = original.firstNode; p; p = p->next)
{
Node* temp = new Node;
temp->value = p->value;
temp->next = 0;
if (lastNode) lastNode->next = temp;
else firstNode = temp;
lastNode = temp;
}
}
//function necessary to create values if they are not stored in the original queue
template
Queue& Queue::operator=(const Queue& original)
{
if (this != &original)
{
// deallocate existing list
while (firstNode)
{
Node* p = firstNode->next;
delete firstNode;
firstNode = p;
}
// build new queue
lastNode = 0; // temporary tail
for (Node* p = original.firstNode; p; p = p->next)
{
Node* temp = new Node;
temp->value = p->value;
temp->next = 0;
if (lastNode) lastNode->next = temp;
else firstNode = temp;
lastNode = temp;
}
siz = original.siz;
}
return *this;
}
#endif
Code:
#include
#include
#include
#include
using namespace std;
#include
#include
#include "MyDynamicArray.h"
#include "MyQueue.h"
struct Customer {
char ID;
int arrivalT;
int endT;
};
int getRandomNumberOfArrivals(double);
char genID(char&);
int randTimeAdd(int, int);
int main()
{
srand(time(0));
rand();
int numServers = 0, waitMaxLength = 0, minServTime = 0, maxServTime = 0, clockStopTime = 0;
double avgArrivalRate = 0.5;
char curAlpha = 'A';
ifstream fin;
fin.open("simulation.txt");
int switchCount = 0;
while (fin.good()) {
string input;
getline(fin, input);
switch (switchCount)
{
case 0:
numServers = atoi(input.c_str());
cout
break;
case 1:
avgArrivalRate = atof(input.c_str());
break;
case 2:
waitMaxLength = atoi(input.c_str());
cout
break;
case 3:
minServTime = atoi(input.c_str());
cout
break;
case 4:
maxServTime = atoi(input.c_str());
cout
break;
case 5:
clockStopTime = atoi(input.c_str());
cout
break;
default:
throw("UH OH - PROBLEM");
}
switchCount++;
}
Queue custQ;
DynamicArray nowServing;
DynamicArray serversStatus;
for (int time = 0; ; time++) {
// handle all services scheduled to complete at this clock time
for (int i = 0; i
if (serversStatus[i]) { //means is busy
if (nowServing[i].endT == time) {
serversStatus[i] = false;
}
}
}
// handle new arrivals -- can be turned away if wait queue is at maximum length!
if (time
int numArrive = getRandomNumberOfArrivals(avgArrivalRate);
for (int i = 0; i
if (custQ.size()
Customer c;
c.ID = genID(curAlpha);
c.arrivalT = time;
custQ.push(c);
}
}
}
// for idle servers, move customer from wait queue and begin
for (int i = 0; i
if (!serversStatus[i] && !custQ.empty()) {
nowServing[i] = custQ.front();
custQ.pop();
nowServing[i].endT = time + randTimeAdd(minServTime, maxServTime);
serversStatus[i] = true;
}
}
//output the summary
//output the current time
//output a visual representation of the servers and the wait queue
cout
cout
cout
cout
for (int i = 0; i
string show = " ";
if (serversStatus[i]) {
show = nowServing[i].ID;
}
cout
if (i == 0) {
Queue tempQ = custQ;
cout
while (!tempQ.empty()) {
cout
tempQ.pop();
}
}
cout
}
int numIdle = 0;
for (int i = 0; i
if (!serversStatus[i]) {
numIdle++;
}
}
if (numIdle == numServers && time >= clockStopTime) {
break;
}
do {
cout
} while (cin.get() != ' ');
}
system("pause");
}
int randTimeAdd(const int a, const int b) {
return a + (rand() % b);
}
char genID(char& curAlpha) {
if (curAlpha == 'Z') {
curAlpha = 'A';
return 'Z';
}
return curAlpha++;
}
int getRandomNumberOfArrivals(double averageArrivalRate) {
int arrivals = 0;
double probOfnArrivals = exp(-averageArrivalRate);
for (double randomValue = (double)rand() / RAND_MAX;
(randomValue -= probOfnArrivals) > 0.0;
probOfnArrivals *= averageArrivalRate / static_cast(++arrivals));
return arrivals;
}
txt file
4 2.5 8 3 10 50
Create a struct to represent a customer object. Include these data members: (1) an ID tag as explained below and (2) service end time. Service end time is the whole number clock time that the customer's service is scheduled to end -- it's calculated when their service begins, as explained later. . The ID tag for the customer is a single letter of the alphabet, A-Z. Assign A to the first-created customer, B to the next, and so on. After the 26th customer is assigned Z, start the IDs over again that is, assign A to the 27th customer. Use the Q&A section of the module to share ideas of how to manage this. Customers arrive at the specified average arrival rate from the beginning of the simulation until the specified clock time at which new arrivals stop. After that time there are no new arrivals, but the simulation continues, allowing the wait queue to empty and the servers to become idle Read 6 input values from a text file simulation.txt that you will write -- one value per line. Do NOT submit this file Use a queue object to represent the wait queue. The queue should store customer objects. Create the nowServing array of customer objects to represent the customers being served. When a customer is removed from the wait queue, you'll copy that customer to the nowServing array. Include another corresponding array of boolean values, whose value is true if the server at that index position is busy serving a customer, false otherwise, indicating that the server is idle. (There's more than one way to accomplish this, so use a different way if you wish). Use your MyDynamicArray and submit its H file with your solution without modification. As soon as a customer starts being helped by a server, the service time interval is determined as a random number in the range from the minimum service time interval to the maximum service time interval. Add the randomly-determined service time interval to the current clock time to compute the future clock time when ervice will end. The possible values for service time interval are whole numbers between the minimum service time and maximum service time, inclusive, all equally likely. If the minimum service time and maximum service time are the same, the service time interval is always the same. If the minimum service time is 1 and the maximum service time is 6, the possible service times are 1, 2, 3, 4, 5, and 6 all equally
Step by Step Solution
There are 3 Steps involved in it
Step: 1
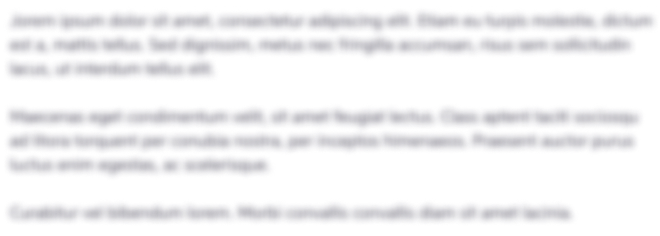
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started