Question
Hi. I need help in my c++ assignement. Rewrite your most recent high scores program so that each name/score pair is stored in a
Hi. I need help in my c++ assignement.
"
Rewrite your most recent high scores program so that each name/score pair is stored in a struct named highscore. Your program should meet the following requirements.
The highscore struct should have two fields:
an int named score
and a char array named name. The char array should have 24 elements, making the maximum length of the name 23. (If you prefer to use a char pointer and a dynamically allocated array, that is fine as well. However, this may result in a number of complications, so be prepared for the challenge.)
The data should be stored in a single array, a dynamically allocated array of highscore structs.
Your program should use three functions that accept the array of highscore structs:
void initializeData(highscore scores[], int size) void sortData(highscore scores[], int size) void displayData(const highscore scores[], int size)
You may use any sort algorithm, but I would recommend using the selection sort from lesson 9.6. Don't use C++'s sort() function, but you can use the swap() function.
Note that when you swap your array elements, you can swap the entire struct. You don't need to swap the name and the score separately."
Old assignement/code to be edited is here:
"
#include
// function prototypes void initializeArrays(string names[], int scores[], int size); void sortData(string names[], int scores[], int size); void displayData(const string names[], const int scores[], int size);
int main()
{ int size;
string *names; names = new string[size]; // string names[SIZE];
int *scores; scores = new int[size]; // int scores[SIZE];
cout << "How many scores will you be entering?: "; cin >> size;
initializeArrays(names, scores, size); sortData(names, scores, size); displayData(names, scores, size);
// Free dynamically allocated memory
delete[] scores; delete[] names; }
// This function takes user input and stores it in a names array and a scores array respectivly. The size integer limits the size of the names and scores array.
void initializeArrays(string names[], int scores[], int size) { string tempName;
for (int i = 0; i < size; i++){
cout << "Enter the name for score #" << i + 1 << ": "; cin >> names[i]; cout << "Enter " << names[i] << "'s score: "; cin >> scores[i]; } }
// This function take the names array, scores array and the size integer as input. It sorts both arrays based on a dual selection sort algorithm. The arrays are sorted in descending order and maintain syncronization. Output is the two sorted arrays.
void sortData(string names[], int scores[], int size) { int startScan, maxIndex; string tempId; double maxValue;
for (startScan = 0; startScan < (size - 1); startScan++){ maxIndex = startScan; maxValue = scores[startScan]; tempId = names[startScan]; for (int index = startScan + 1; index < size; index++){ if (scores[index] > maxValue){ maxValue = scores[index]; tempId = names[index]; maxIndex = index; } } scores[maxIndex] = scores[startScan]; names[maxIndex] = names[startScan]; scores[startScan] = maxValue; names[startScan] = tempId; } }
// This function takes the sorted names array, sorted scores array, and the size int as input. Using a simple for loop it displays the both arrays, in descending order, in an easy to read format.
void displayData(const string names[], const int scores[], int size) { cout << "Top Scorers:" << endl;
for (int i = 0; i < size; i++){ cout << setw(8) << names[i] << ":" << setw(8) << scores[i] << endl; }
}
"
Step by Step Solution
There are 3 Steps involved in it
Step: 1
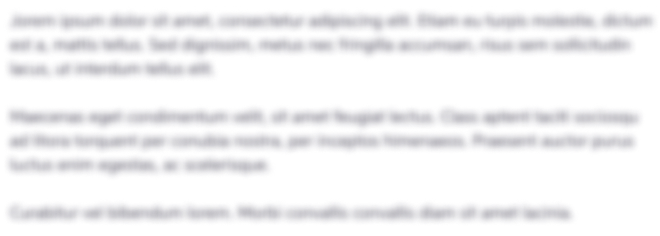
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started