Question
Hi. I need help on my C++ homework. I just need help on Part 3 of this assignment. I've also already finished VEHICLE.H and VEHICLE.CPP
Hi. I need help on my C++ homework. I just need help on Part 3 of this assignment. I've also already finished VEHICLE.H and VEHICLE.CPP which you can find at the very bottom. I now need help with getting code for SHOWROOM.H, DEALERSHIP.H, DEALERSHIP.CPP, and most ****importantly*** SHOWROOM.CPP.
Last thing, can you make the code organized too?
Thanks for the help!
-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
INSTRUCTIONS:
----
-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
ALREADY WRITTEN CODE FOR VEHICLE.H AND VEHICLE.CPP:
---VEHICLE.H---
#ifndef VEHICLE_H #define VEHICLE_H #include
/* Vehicle class */ class Vehicle {
private: string make; string model; unsigned int year; float price; unsigned int mileage; public: Vehicle(); Vehicle(string make, string model, int year, float price, int mileage);
void Display(); string GetYearMakeModel(); float GetPrice(); };
#endif
---VEHICLE.CPP---
#include "Vehicle.h"
// Default constructor, intializes variables to default values Vehicle::Vehicle() {
// set the default values for member variables make = "COP3503" ; model = "Rust Bucket"; year = 1900; price = 0; mileage = 0; }
// Parameterized constructor to set the values for member variables Vehicle::Vehicle(string make, string model, int year, float price, int mileage) { this->make = make; this->model = model; this->year = year; this->price = price; this->mileage = mileage;
}
void Vehicle::Display() { std::cout
}
string Vehicle::GetYearMakeModel() { string yearMakeModel = ""; yearMakeModel = to_string(year) + " " + make + " " + model; return yearMakeModel; }
float Vehicle::GetPrice() { return price; }
Overview The purpose of this assignment is give you some experience writing classes in C++, the various special functions they make use of (such as copy constructors, assignment operators, and destructors), as well as an introduction to dynamically allocating memory within those classes. New Keywords / Language concepts - Classes - conceptually similar to other languages - The std::vector class - similar to Java's ArrayList class, an expandable container - The std::string class - similar in many ways to strings in most every language Description This program will represent a hypothetical car dealership, which consists of showrooms that contain the vehicles for sale. To that end, there are three classes you will be writing: - Vehicle - Showroom - Dealership For this assignment, main.cpp will be provided for you, so you don't have to worry about the structure of the program. Instead, you can focus solely on the structure of the classes and their interactions. Vehicle The Vehicle class is the basic container of this assignment. You will need to store the following data as private data members of the class: - A std::string to store the make of the vehicle (such as Mazda, Toyota, etc) - A std::string to store the model of the vehicle (such as Mustang, Model S, F-150, etc) - An unsigned integer to store the year - A float to store the price - An unsigned integer to store the number of miles the vehicle has been driven // Default constructor, initializes variables to default values Vehicle(); Vehicle(string make, string model, int year, float price, int mileage); // Print out the vehicle's details in a single line: // 1973 Ford Mustang $9500113000 void Display(); // create and return a string in the form of "YEAR MAKE MODEL" // Example: "1970 Ford Mustang" string GetYearMakeModel(); // Return the price float GetPrice(); Default values and constructors While the definition of "appropriate defaults" may vary from one scenario to the next, for this assignment you can use these values as your defaults: These defaults are chosen arbitrarily for this assignment-for your own projects, you can of course choose anything that you like. Showroom The Showroom class is a bit more sophisticated. Its purpose is to store a collection of Vehicle objects. Each Showroom that you create could have a different number of Vehicles (depending on its size), so for this assignment we'll use a vector. Your Showroom should contain variables for the following: - The name of the Showroom - A vector> to store Vehicle objects - A maximum capacity of the showroom (we don't want to add Vehicles beyond this limit) In addition, you should create the following functions: // Default constructor (all parameters have default values) Showroom(string name = "Unnamed Showroom", unsigned int capacity =0); // Accessor vector GetVehicleList(); // Behaviors void AddVehicle(Vehicle v); void ShowInventory( ); float GetInventoryValue(); Dealership The Dealership class in some ways is very similar to the Showroom. Instead of Vehicles, it will store a vector of Showroom objects. It will also need a name and a capacity. In addition, you will need functions: // Constructor Dealership(string name = "Generic Dealership", unsigned int capacity = 0 ); // Behaviors void AddShowroom(Showroom s); float GetAveragePrice(); void ShowInventory(); Relevant Reading zyBooks chapters: Strings Arrays / Vectors (specifically the section on vectors) Objects and Classes User-Defined Functions (specifically the section on Pass by Reference) Canvas->Pages->strings - a more in-depth look at strings Tips A few tips about this assignment: - You can print out a tab character (the escape sequence ' \t ) to help line up the output. - Don't try to tackle everything all at once. Work on one class at a time. Can't really have a Dealership without a Showroom, which really needs Vehicles... - An extension of that: work on one function, one class variable at a time. Create a constructor, initialize a single variable, test that out. When that works, move to the next part, and so on. Example Output Default constructoroutput 1900 COP3503 Rust Bucket $0.000 Unnamed Showroom is empty! Generic Dealership is empty! Average car price: $0.00 Showroom is full! Cannot add Dodge Caravan 1992 Vehicles in Example Showroom 2018 Bugatti Chiron $12447.00 4 2013 Chrysler Sebring $1819.00 22987 Dealership output and error message when Dealership is full Dealership is full, can't add another showroom! Vehicles in Room One 1998 Dodge Neon \$500.00 932018 Vehicles in Room Two 2001 Ford Escort \$2000.00 125900 2004 Ford F-150 \$500.00 7392 Average car price: \$1000.00 Calling just the GetAveragePrice() function of the dealership Using just the GetAveragePrice() function Average price of the cars in the dealership: $19272.81 In this part you will write the final class, the Dealership. This classes utilizes both the Showroom and Vehicle class, so be sure to have those completed first. main.cpp is provided for you. is marked as read only Current file: main.cpp - \begin{tabular}{l|l} \#include "Vehicle.h" & main.cpp \\ \#include "Showroom.h" & \\ \#include "Dealership.h" & Vehicle.h \\ \#include & \\ \#include & Showroom.h \\ using namespace std; & \\ void TestOne(Vehicle vehicles[]); & Vehicle.cpp \\ void TestTwo(Vehicle vehicles[]); & \\ void TestThree(Vehicle vehicles[]); & Dealership.h \\ void TestFour(Vehicle vehicles[]); & \\ void TestFive(Vehicle vehicles[]); & Dealership.cpp \\ void TestSix(Vehicle vehicles[]); & \\ \hline intmain(){Showroom.cpp \end{tabular} // Initialize some data. It's hard-coded here, but this data could come from a file, database, etc Vehicle vehicles [ ] = \{ Vehicle("Ford", "Mustang", 1973, 9500, 113000), Vehicle("Mazda", "CX-5", 2017, 24150, 5900), Vehicle("Dodge", "Charger", 2016, 18955, 9018), Vehicle("Tesla", "Model S", 2018, 74500, 31), Vehicle("Toyota", "Prius", 2015, 17819, 22987), Vehicle("Nissan", "Leaf", 2016, 12999, 16889), Vehicle("Chevrolet", "Volt", 2015, 16994, 12558), \} // Set the precision for showing prices with 2 decimal places cout std:: fixed std: : setprecision(2); int testNum; cin testNum; if ( testNum ==1 ) TestOne(vehicles); else if (testNum ==2 ) TestTwo(vehicles); else if (testNum ==3 ) TestThree(vehicles); else if (testNum =4 ) TestFour(vehicles); else if (testNum ==5 ) TestFive(vehicles); else if (testNum =6 ) TestSix(vehicles); void TestFour(Vehicle vehicles [ ) \{ // Showrooms to store the vehicles Showroom showroom("Primary Showroom", 3); showroom. AddVehicle(vehicles[0]); showroom. AddVehicle(vehicles[1]); showroom.AddVehicle(vehicles[6]); Showroom secondary("Fuel-Efficient Showroom", 4); secondary. AddVehicle(vehicles[4]); secondary.AddVehicle(vehicles [5]); Showroom third("Fuel-Efficient Showroom", 4); third.AddVehicle(vehicles[3]); third.AddVehicle(vehicles[3]); third. AddVehicle(vehicles [3]); // A "parent" object to store the Showrooms Dealership dealership("COP3503 Vehicle Emporium", 3); dealership. AddShowroom(showroom); dealership. AddShowroom(secondary); dealership.AddShowroom(third); cout "Average price of the cars in the dealership: \( \$ " \ll \)quot; dealership.GetAveragePrice(); void TestFive(Vehicle vehicles [ ) \{ // Showrooms to store the vehicles Showroom showroom("Primary Showroom", 6); showroom. AddVehicle(vehicles[0]); showroom.AddVehicle(vehicles[1]); showroom. AddVehicle(vehicles [2]); showroom.AddVehicle(vehicles[3]); showroom.AddVehicle(vehicles[4]); showroom.AddVehicle(vehicles[5]); Showroom secondary("Fuel-Efficient Showroom", 4); secondary. AddVehicle(vehicles[4]); secondary. AddVehicle(vehicles[5]); secondary.AddVehicle(vehicles[5]); Showroom third("Fuel-Efficient Showroom", 4); third.AddVehicle(vehicles [3]); third. AddVehicle(vehicles [4]); third. AddVehicle(vehicles[5]); third.AddVehicle(vehicles[6]); // A "parent" object to store the Showrooms Dealership dealership("COP3503 Vehicle Emporium", 3); dealership.AddShowroom(showroom); dealership. AddShowroom(secondarv)Step by Step Solution
There are 3 Steps involved in it
Step: 1
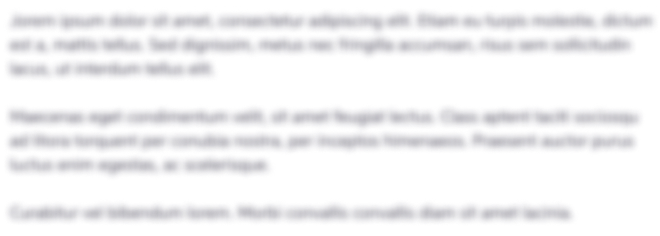
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started