Question
Hi I need help on this java assingment for my Operating Systems class. Thank you! Recall that to solve a readers/writers problem, create a multi-thread
Hi I need help on this java assingment for my Operating Systems class. Thank you!
Recall that to solve a readers/writers problem, create a multi-thread program to simulate the problem. The following conditions must be satisfied:
1. Any number of readers may simultaneously read the file.
2. Only one writer at a time may write to the file.
3. If a writer is writing to the file, no reader may read it.
Implement following two solutions and output the execution sequences for given test scenarios:
1. Readers Have Priority
Test your simulators behavior for
a. 5 readers and 1 writer
b. 3 readers and 3 writers
c. 1 reader and 5 writers
2. Writers Have Priority
Test your simulators behavior for
a. 5 readers and 1 writer
b. 3 readers and 3 writers
c. 1 reader and 5 writers
Classes that came with assignment:
class TwoThreadsTest { public static void main (String[] args) { new SimpleThread("Jamaica").start(); new SimpleThread("Fiji").start(); } }
Class 2:
class SimpleThread extends Thread { public SimpleThread(String str) { super(str); } public void run() { for (int i = 0; i < 10; i++) { System.out.println(i + " " + getName()); try { sleep((int)(Math.random() * 1000)); } catch (InterruptedException e) {} } System.out.println("DONE! " + getName()); } }
Class 3:
import java.util.concurrent.Semaphore; //Solving the mutual exclusion problem using Semaphore class class Process2 extends Thread { private int id; private Semaphore sem; public Process2(int i, Semaphore s) { id = i; sem = s; } private int random(int n) { return (int) Math.round(n * Math.random() - 0.5); } private void busy() { try { sleep(random(500)); } catch (InterruptedException e) { } } private void noncritical() { System.out.println("Thread " + id + " is NON critical"); busy(); } private void critical() { System.out.println("Thread " + id + " entering critical section"); busy(); System.out.println("Thread " + id + " leaving critical section"); } public void run() { for (int i = 0; i < 2; ++i) { noncritical(); try { sem.acquire(); } catch (InterruptedException e) { // ... } critical(); sem.release(); } } public static void main(String[] args) { final int N = 4; System.out.println("Busy waiting..."); //Semaphore(int permits, boolean fair) Semaphore sem = new Semaphore(1, true); Process2 p[] = new Process2[N]; for (int i = 0; i < N; i++) { p[i] = new Process2(i, sem); p[i].start(); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
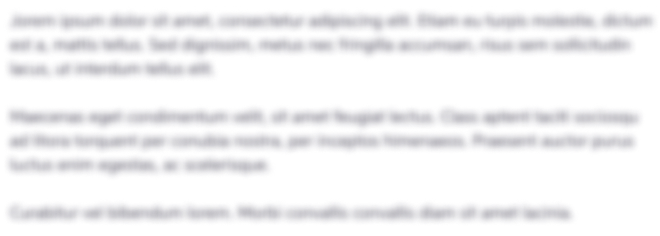
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started