Question
Hi I need help with a Clock class in java I need to create a method to add and make another method to subtract two
//class Clock
public class Clock { private int hr; //store hours private int min; //store minutes private int sec; //store seconds
//Default constructor //Postcondition: hr = 0; min = 0; sec = 0 public Clock() { setTime(0, 0, 0); System.out.println("Inside the version with parameters! "); }
//Constructor with parameters, to set the time //The time is set according to the parameters //Postcondition: hr = hours; min = minutes; sec = seconds public Clock(int hours, int minutes, int seconds) { setTime(hours, minutes, seconds); System.out.println("Inside the version with parameters! ");
}
//Method to set the time //The time is set according to the parameters //Postcondition: hr = hours; min = minutes; sec = seconds public void setTime(int hours, int minutes, int seconds) { if (0 => hr = hours; else hr = 0;
if (0 => min = minutes; else min = 0;
if (0 => sec = seconds; else sec = 0; }
//Method to return the hours //Postcondition: The value of hr is returned. public int getHours() { return hr; }
//Method to return the minutes //Postcondition: The value of min is returned. public int getMinutes() { return min; }
//Method to return the seconds //Postcondition: The value of sec is returned. public int getSeconds() { return sec; }
//Method to print the time //Postcondition: Time is printed in the form hh:mm:ss. public void printTime() { if (hr System.out.print("0"); System.out.print(hr + ":");
if (min System.out.print("0"); System.out.print(min + ":");
if (sec System.out.print("0"); System.out.print(sec); }
//Method to increment the time by one second //Postcondition: The time is incremented by one second. //If the before-increment time is 23:59:59, the time //is reset to 00:00:00. public void incrementSeconds() { sec++;
if (sec > 59) { sec = 0; incrementMinutes(); //increment minutes } }
//Method to increment the time by one minute //Postcondition: The time is incremented by one minute. //If the before-increment time is 23:59:53, the time //is reset to 00:00:53. public void incrementMinutes() { min++;
if (min > 59) { min = 0; incrementHours(); //increment hours } }
//Method to increment the time by one hour //Postcondition: The time is incremented by one hour. //If the before-increment time is 23:45:53, the time //is reset to 00:45:53. public void incrementHours() { hr++;
if (hr > 23) hr = 0; }
//Method to compare the two times //Postcondition: Returns true if this time is equal to // otherClock; otherwise returns false public boolean equals(Clock otherClock) { return (hr == otherClock.hr && min == otherClock.min && sec == otherClock.sec); }
//Method to copy the time //Postcondition: The instance variables of otherClock are // copied into the corresponding data members // of this time. // hr = otherClock.hr; min = otherClock.min; // sec = otherClock.sec. public void makeCopy(Clock otherClock) { hr = otherClock.hr; min = otherClock.min; sec = otherClock.sec; }
//Method to return a copy of the time //Postcondition: A copy of the object is created // and a reference of the copy is returned. public Clock getCopy() { Clock temp = new Clock();
temp.hr = hr; temp.min = min; temp.sec = sec;
return temp; }
public String toString() { String str = "";
if (hr str = "0"; str = str + hr + ":";
if (min str = str + "0" ; str = str + min + ":";
if (sec str = str + "0"; str = str + sec;
return str; } public void addTimes(Clock Clockb, Clock myClock) { hr += Clockb.getHours(); min +=Clockb.getMinutes(); sec +=Clockb.getSeconds(); //Fixing overflow problem if(sec > 59) { min++; sec %=60; } if(min>59) { hr++; min%=60; } if(hr>60) { hr %=3600; } System.out.println(hr + "hr" + min + "min" + sec + "sec");
} public void subTimes(Clock Clockb, Clock myClock) { int totalSeconds, totalSeconds2; totalSeconds=Clockb.hr-(3600*min*60-sec); totalSeconds2=myClock.hr*3600; totalSeconds=Clockb.min-(sec*60); totalSeconds=myClock.min*sec; totalSeconds=Clockb.min-(sec * 60); totalSeconds=myClock.sec*sec; System.out.println(hr + "hr" +min +"min" + sec +"sec");
} }
import java.util.*;
public class TestProgClock { static Scanner console = new Scanner(System.in);
public static void main(String[] args) { Clock myClock = new Clock(5,4,30); //Line 1 Clock yourClock = new Clock(0,0,0); //Line 2 Clock sumClock = new Clock(0,0,0); int hours; //Line 3 int minutes; //Line 4 int seconds; //Line 5
System.out.println("Line 6: myClock: " + myClock); //Line 6
System.out.println("Line 7: yourClock: " + yourClock); //Line 7
yourClock.setTime(5,45,16); //Line 8
System.out.println("Line 13: After setting the " + "time, yourClock: " + yourClock); //Line 9
if (myClock.equals(yourClock)) //Line 10 System.out.println("Line 11: Both times " + "are equal."); //Line 11 else //Line 12 System.out.println("Line 13: The two times " + "are not equal"); //Line 13
System.out.print("Line 14: Enter the hours, " + "minutes, and seconds: "); //Line 14
hours = console.nextInt(); //Line 15 minutes = console.nextInt(); //Line 16 seconds = console.nextInt(); //Line 17 System.out.println(); myClock.setTime(hours,minutes,seconds); //Line 19
System.out.println("Line 20: New myClock: " + myClock); //Line 20
myClock.incrementSeconds(); //Line 21 //Line 18
System.out.printf("Line 22: After incrementing " + "the clock by one second, " + "myClock: %s%n", myClock); //Line 22
yourClock.makeCopy(myClock); //Line 23
System.out.println("Line 24: After copying " + "myClock into yourClock, " + "yourClock: " + yourClock); //Line 24 System.out.println("MyClock= " + myClock); //Line 18 sumClock.addTimes(yourClock, myClock); System.out.println("sumClock= " + sumClock); System.out.println("MyClock= " + myClock); //Line 18 myClock.subTimes(yourClock, myClock); System.out.println("diffClock= " + myClock); }//end main }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
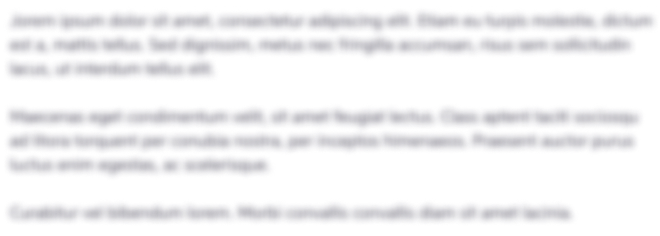
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started