Question
Hi, I need help with this assignment: write a Java program to simulate playing a number tile game Number tiles are squares with a number
Hi, I need help with this assignment:
write a Java program to simulate playing a number tile game
Number tiles are squares with a number from 1 to 9 at each side. A board for the game is a sequence of number tiles satisfying this property:
The right side of each tile (except the last) = the left side of the next tile on the board
There are 2 players in the game, both of which are the computer. Each player starts with a "hand" of 5 number tiles. A move is made by a player removing tile from the hand and placing it on the board, if possible. A tile may be placed on the board in any of 3 ways:
As the new first tile
Between two adjacent tiles
As the new last tile
A number tile in the players hand can be rotated 90 degrees but once placed on the board its position is fixed
If none of the tiles in the hand can be placed on the board, then one random tile is added to the player's hand
A round is when player1 makes a move and then player2 makes a move (to make the game fair)
The game ends when all tiles are removed from one (or both) player's hand(s). If one player's hand is empty and the other player's hand still contains tiles, then the player with the empty hand is the winner. If both players hands are empty, then the game ends in a tie
III. Specifications
Your program will consist of 5 classes
The NumberTile class models a number tile
The Hand class models a players hand of number tiles
The Board class models the board where tiles are inserted from the hands
The TileGame class conducts the game
Test class with main method Use the skeletons of the NumberTile, Hand, Board, and TileGame classes, available online. Do not add or remove any methods, and do not modify any of the method declarations. Simply code the bodies of the method stubs where necessaryYour main method will do this and only this: Create a TileGame object, call the play() method, and then print the results returned by the toString() method. After each game, ask whether the user wants to see another. To get credit, you must use the declared ArrayList in each class as your principal data structure. To get credit, all instance variables in every class must be declared private.
These are the classes:
NumberTile.java
import java.util.ArrayList ; /** * A NumberTile is a square tile with an int between 1 and 9, inclusive, on * each side */ public class NumberTile { private ArrayListtile ; /** * Create a NumberTile object using 4 random ints in the range 1..9 */ public NumberTile() { } /** * Rotate this tile 90 degrees clockwise */ public void rotate() { } /** * Get the number on the left side of this tile * @return the number on the left side of this tile */ public int getLeft() { // DO NOT MODIFY THIS METHOD! // ========================= return tile.get(0) ; } /** * Get the number on the right side of this tile * @return the number on the right side of this tile */ public int getRight() { // DO NOT MODIFY THIS METHOD! // ========================= return tile.get(2) ; } /** * Return a String representation of this tile in the form * * 4 * 5 7 * 1 * * @return the tile as a multi-line String */ public String toString() { // temporary return statement so skeleton will compile and run return null; } } // end of NumberTile class
Hand.java
import java.util.ArrayList; /** * A Hand is a collection of NumberTiles. Tiles may be removed from * the Hand and new tiles added to it */ public class Hand { private ArrayListhand ; private static int HAND_SIZE = 5 ; // starting hand size /** * Create a new Hand of HAND_SIZE tiles */ public Hand() { } /** * Create a new Hand as an exact duplicate (i.e. a "deep copy") of another * @param toBeCopied the Hand to be copied */ public Hand(Hand toBeCopied) { } /** * Get the tile at a specified index in this Hand * @param index the index (position) of the tile to be returned * @return the NumberTile at the specified index */ public NumberTile get(int index) { // temporary return statement so skeleton will compile and run return null ; } /** * Get the size of this Hand * @return the number of tiles in the Hand */ public int getSize() { // temporary return statement so skeleton will compile and run return -999 ; } /** * Add a new tile to this Hand */ public void addTile() { } /** * Remove the tile at a specified index (position) from this Hand * @param index the index (position) of the tile to be removed * */ public void removeTile(int index) { } /** * Is this Hand empty? * @return true if this Hand is empty (contains no tiles); otherwise false * */ public boolean isEmpty() { // temporary return statement so skeleton will compile and run return true ; } /** * Get a String representation of this Hand * @return the NumberTiles in this Hand as a multi-line String */ public String toString() { // DO NOT MODIFY THIS METHOD! // ========================== return hand.toString() ; // call toString of ArrayList class } }
Board.java
import java.util.ArrayList; /** * A Board is a collection of Number Tiles */ public class Board { private ArrayListboard ; /** * Create a new Board with one NumberTile */ public Board() { } /** * Get the tile at a specified index on this Board * @param index the index (position) of the tile to be returned * @return the NumberTile at the specified index */ public NumberTile getTile (int index) { // temporary return statement so skeleton will compile and run return null; } /** * Get the size of this Board * @return the number of tiles on the Board */ public int getSize() { // temporary return statement so skeleton will compile and run return -999 ; } /** * Add a new tile to this Board at a specified index (position) * @param index the index (position) at which to insert the tile * @param tile the tile to be inserted */ public void addTile(int index, NumberTile tile) { } /** * Get a String representation of this Board * @return the NumberTiles on the Board as a multi-line String */ public String toString() { // DO NOT MODIFY THIS METHOD! // ========================== return board.toString() ; // call toString of ArrayList class } }
TileGame.java
public class TileGame { // instance variable declarations go here // Create a Tilegame object public TileGame() { } // Play the game public void play() { } // If the current tile fits in the board, returns the index at which // it will be inserted. If the tile does not fit, returns -1 private int getIndexForFit(NumberTile tile) { // temporary return statement so skeleton will compile and run return -999; } // Make a move from a hand. If a tile in the hand fits on the board // then remove it from the hand and place it in the board. The tile may // be rotated up to 3 times. If no tile from the hand fits, then add // another tile to the hand private void makeMove(Hand hand) { } // Get the results of the game as a humongous multi-line String containing // both starting hands, the final board, both final hands, and a message // indicating the winner // HINT: call the toString methods of the Hand and Board classes public String toString() { // temporary return statement so skeleton will compile and run return "Hi Mom!" ; } } // end of TileGame class
Step by Step Solution
There are 3 Steps involved in it
Step: 1
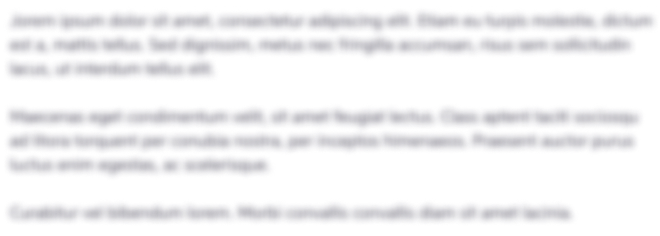
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started