Question
Hi I need the main method and database connection. Thank you so much. You are asked to write a Java program for the programming quiz
Hi I need the main method and database connection. Thank you so much.
You are asked to write a Java program for the programming quiz system. There are two types of questions: Multiple Choice Question and Ture/False Question. User can create questions using the system; and preview the quiz, which display all questions in the system one by one. During the preview, the user can attempt the quiz by entering his/her answers to questions. The system will then immediately check the answer and calculate. After attempting all questions, the total score will be displayed.
You must create a class Quiz, which has the main method to run the program.
You must read/write from/to the database provided in this question (Question.accdb). This MS Access file contains a table called Questions. It contains the following fields: a. ID (Automatically generated integer by MCAccess) b. QText (Short Text): The question text c. Answer (Short Text): i. If this is a multiple-choice question, Answer stores all options separated by ##. There is a leading * for the correct option. ii. If this is a True/False question, Answer stores the string Ture or False. d. Point (Single): It is a float point number representing the point of this question. e. Type (Short Text): MC or TF denoting multiple-choice or True/False questions respectively.
Requirement: 1. The output of the program MUST EXACTLY the same as the above sample run of in character level. 2. You must create classes according to the following class diagram (Note: italic font refers to abstract methods/classes, while normal font refers to concrete methods/classes):
Question Class: - This class represents the generic form of question. It contains the question text (qText) and the point of a question (point). - The grade method, which is an abstract method, has a parameter, answer (String). It returns the points of the question if the answer is correct; zero otherwise. - The getCorrectAnswer method, which is an abstract method, has no parameter. It returns a String representing the correct answer to the question. MCQuestion Class - It is a subclass of Question. - It represents a multiple-choice question. A multiple-choice question may have 3-5 options. - Each element of the instance variable options refers to an option in this question. - The instance variable answer is a single-character string. It saves the correct answer (A, B, C, D or E) to this question.
- The method setOptions(String options) has the answer field from the database (explained below) as the parameter. It will add all options to the instance variable options and set the instance variable answer. - The constructor MCQuestion(String qText, String options, double point) creates an MCQuestion object by setting the qText, adding option to options , setting the correctAnswer to answer and point by reading the QText, Answer, and Point fields from the database. TFQuestion Class - It is a subclass of Question. - It presents a True/False question. - The constructor TFQuestion(String qText, boolean answer, double point) creates an TFQuestion object by setting the qText, answer, and point by reading the qText, Answer, and Point fields from the database.
A sample run of the program is shown as below (Green text refers to user input): Please choose (c)reate a question, (p)review or (e)xit >> c Enter the type of question (MC or TF) >> MC Enter the question text >> Each primitive type in Java has a corresponding class contained in the java.lang package. These classes are called ____ classes. How many options? 4 Enter Option A (Start with * for correct answer) >> case Enter Option B (Start with * for correct answer) >> primitive Enter Option C (Start with * for correct answer) >> *type-wrapper Enter Option D (Start with * for correct answer) >> show How many points? 3 Please choose (c)reate a question, (p)review or (e)xit >> c Enter the type of question (MC or TF) >> MC Enter the question text >> A(n) ____ variable is known only within the boundaries of the method. How many options? 5 Enter Option A (Start with * for correct answer) >> method Enter Option B (Start with * for correct answer) >> *local Enter Option C (Start with * for correct answer) >> double Enter Option D (Start with * for correct answer) >> instance Enter Option E (Start with * for correct answer) >> global How many points? 2 Please choose (c)reate a question, (p)review or (e)xit >> c Enter the type of question (MC or TF) >> TF
Enter the question text >> Java is a free-form programming language. Answer is True or False? True How many points? 1 Please choose (c)reate a question, (p)review or (e)xit >> p Each primitive type in Java has a corresponding class contained in the java.lang package. These classes are called ____ classes. (3.0 Points) A: case B: primitive C: type-wrapper D: show Enter your choice >> A You are wrong. The correct answer is C. A(n) ____ variable is known only within the boundaries of the method. (2.0 Points) A: method B: local C: double D: instance E: global Enter your choice >> B You are correct! Java is a free-form programming language. (1.0 Points) True(T) or False(F) >> F You are wrong. The correct answer is true. The quiz ends. Your score is 2.0. Please choose (c)reate a question, (p)review or (e)xit >> c Enter the type of question (MC or TF) >> MC Enter the question text >> A(n) ____ constructor is one that requires no arguments. How many options? 3 Enter Option A (Start with * for correct answer) >> class Enter Option B (Start with * for correct answer) >> *default Enter Option C (Start with * for correct answer) >> explicit How many points? 2 Please choose (c)reate a question, (p)review or (e)xit >> c Enter the type of question (MC or TF) >> TF Enter the question text >> Javascript and Java are the same. Answer is True or False? False How many points? 0.5 Please choose (c)reate a question, (p)review or (e)xit >> p Each primitive type in Java has a corresponding class contained in the java.lang package. These classes are called ____ classes. (3.0 Points) A: case B: primitive C: type-wrapper D: show Enter your choice >> C You are correct! A(n) ____ variable is known only within the boundaries of the method. (2.0 Points) A: method B: local C: double D: instance E: global Enter your choice >> B You are correct! Java is a free-form programming language. (1.0 Points) True(T) or False(F) >> T You are correct! A(n) ____ constructor is one that requires no arguments. (2.0 Points) A: class B: default C: explicit Enter your choice >> C You are wrong. The correct answer is B. Javascript and Java are the same. (0.5 Points) True(T) or False(F) >> F You are wrong. The correct answer is false. The quiz ends. Your score is 6.5. Please choose (c)reate a question, (p)review or (e)xit >> e Goodbye!
-------------------------
So I have my supper class and subclasses.
public abstract class Question {
private String qText;
private double point;
public Question() {
this.qText = "";
}
public Question(String qText) {
this.qText = qText;
}
/**
* @return the qText
*/
public String getqText() {
return qText;
}
/**
* @param qText the qText to set
*/
public void setqText(String qText) {
this.qText = qText;
}
/**
* @return the point
*/
public double getPoint() {
return point;
}
/**
* @param point the point to set
*/
public void setPoint(double point) {
this.point = point;
}
public abstract double grade(String answer);
public abstract String getCorrectAnswer();
}
MCQuestion.java:
import java.util.ArrayList;
public class MCQuestion extends Question{
private ArrayList
private String answer;
public MCQuestion() {
this.options = new ArrayList();
}
/**
* @param qText
* @param options
* @param answer
*/
public MCQuestion(String qText, ArrayList
super(qText);
this.options = options;
this.setPoint(point);;
}
/**
* @return the options
*/
public ArrayList
return options;
}
/**
* @param options the options to set
*/
public void setOptions(ArrayList
this.options = options;
}
/**
* @param options the options to set
*/
public void setOptions(String options) {
this.options.add(options);
}
/**
* @return the answer
*/
public String getAnswer() {
return answer;
}
/**
* @param answer the answer to set
*/
public void setAnswer(String answer) {
this.answer = answer;
}
@Override
public double grade(String answer) {
if(getCorrectAnswer().equalsIgnoreCase(answer))
return getPoint();
else
return 0;
}
@Override
public String getCorrectAnswer() {
return answer;
}
}
TFQuestion.java:
public class TFQuestion extends Question {
private boolean answer;
public TFQuestion() {
super();
}
/**
* @param answer
*/
public TFQuestion(String qText, boolean answer, double point) {
super(qText);
this.answer = answer;
this.setPoint(point);
}
/**
* @return the answer
*/
public boolean isAnswer() {
return answer;
}
/**
* @param answer the answer to set
*/
public void setAnswer(boolean answer) {
this.answer = answer;
}
@Override
public double grade(String answer) {
if(getCorrectAnswer().equalsIgnoreCase(answer))
return getPoint();
else
return 0;
}
@Override
public String getCorrectAnswer() {
return String.valueOf(answer);
}
}
Wong, Ivan WI File Table Tell me Fields ab Question: Database- C:\Temp\Question.accdb (Acces... Home Create External Data Database Tools Help 21 al View Paste Filter Refresh Find 2. Y All X Views Clipboard Sort & Filter Records Find All Ac... Questions ID QText Answer Point Search. New) Tables Ques Text Formatting Type Click to Add- 0 No Hill Search Record 1 of 1 Datasheet View Figure 1: No records at the beginning Question Database - CATemp\Question accobs (Acces Table Tools Wong. Ivan WI File Home Create Extemal Data Database Tools Help Fields Table Tell me 21 Calibri (Detail) - 11 View BIU Paste Filter Refresh Find All- A. Views Clipboard Sort & Filter Records Find Text Formatting All Ac... Questions ID Search QText Answer Point Type 8 Each primitive t case##primitive##*type-wrapper##show 3 MC Tables (New) 0 JIM Click to Search Record 11 of 1 Datasheet View Figure 2: After creating the first question in the sample runStep by Step Solution
There are 3 Steps involved in it
Step: 1
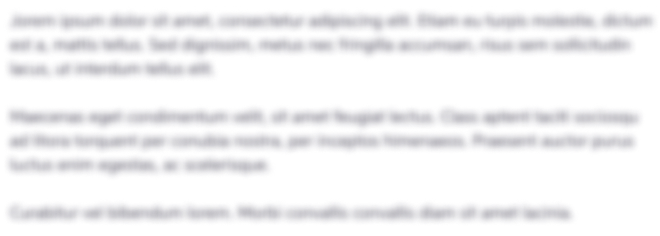
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started