Question
Hi, i was given this c++ program that doesnt compile, and my job is to implement the farm class so it does compile. Im just
Hi, i was given this c++ program that doesnt compile, and my job is to implement the farm class so it does compile. Im just not sure where to start working. Ive been giving two files but one of them is a .cpp file and the other is .h file and the main program. Im just not sure what program i should be working on. I feel like im over thinking it, sorry if im asking a very simple question.
Here are the programs
// Homework8.cpp : This file contains the 'main' function. Program execution begins and ends there.
//
#include "pch.h"
#include
#include "Farm.h"
#include
using namespace std;
int main()
{
// You can't change main. I'm not even going to look at main when I grade so if you change the names of the methodsthey just won't work.
// This is actually a super common pattern for new programmers at a job. You are given one small part of a large system and you need
// to make sure it works according to the larger system's needs.
Farm myFarm;
cout << "Name of farm? ";
string name;
cin >> name;
myFarm.SetName(name);
myFarm.PlantPotatoes(14);
cout << "I have " << myFarm.GetPotatoCount() << " potatoes";
myFarm.PlantSquash(27);
cout << "I have " << myFarm.GetSquashCount() << " squash...es?";
cout << "I have " << myFarm.GetTotalCount() << " things planted total.";
return 0;
}
//////////////////////
// Farm.cpp
#include "pch.h" #include "Farm.h"
Farm::Farm() {
}
Farm::~Farm() {
}
/////////////////
// Farm.h
#pragma once
class Farm
{
public:
Farm();
~Farm();
// This project doesn't compile at the start. Main has called a bunch of methods on Farm that don't exist.
// You have to write them. "Stubs" will help here. If you make a stub for each method, then you can write
// and test them one at a time instead of waiting until the whole program compiles. Remember, a stub
// is a method that does nothing. It just has the minimum amount of code to make it compile. Usually
// that means completely empty, but if the method returns a value the stub may have a "return 0;" or something.
// Make sure your properties are private and your methods are public.
//FOR PROTOYPES
};
Step by Step Solution
There are 3 Steps involved in it
Step: 1
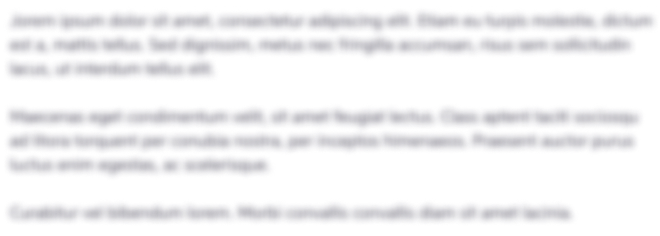
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started