Question
Hi, I'm having some problems with my Dictionary.c code. When I try to run DictionaryClient.c, there is a segmentation fault when I call the delete()
Hi, I'm having some problems with my Dictionary.c code. When I try to run DictionaryClient.c, there is a segmentation fault when I call the delete() function. I've included the DictionaryClient.c and the Dictionary.h programs along with the expected output of DictionaryClient.c. Please help me rewrite my code.
Dictionary.c:
#include
typedef struct NodeObj{ char* key; char* value; struct NodeObj* next; } NodeObj;
typedef NodeObj* Node;
Node newNode(char* k, char* v){ Node N = malloc(sizeof(NodeObj)); assert(N !=NULL); N->key = k; N->value = v; N->next = NULL; return (N); }
void freeNode(Node* pN){ if (pN !=NULL && *pN != NULL){ free(*pN); *pN = NULL; } }
typedef struct DictionaryObj{ Node head; int numItems; } DictionaryObj;
Dictionary newDictionary(void){ Dictionary D = malloc(sizeof(DictionaryObj)); assert(D !=NULL); D->head = NULL; D->numItems = 0; return D; }
void freeDictionary(Dictionary* pD){ if (pD != NULL && *pD != NULL){ if(!isEmpty(*pD)) makeEmpty(*pD); free(*pD); *pD =NULL; } }
int isEmpty(Dictionary D){ if (D ==NULL){ fprintf(stderr, "Stack Error: calling isEmpty() on NULL Dictionary reference " ); exit(EXIT_FAILURE); } return (D->numItems==0); }
int size(Dictionary D){ if (D ==NULL){ fprintf(stderr, "Stack Error: calling size() on NULL Dictionary reference " ); exit(EXIT_FAILURE); } return D->numItems; }
char* lookup(Dictionary D, char* k){ Node N = D->head; while(N != NULL){ if(strcmp(N->key, k)==0){ return N->value; } N = N->next; } return NULL; }
void insert(Dictionary D, char* k, char* v){ Node N = D->head; if(D==NULL){ fprintf(stderr, "Stack Error: calling insert() on NULL Dictionary reference "); exit(EXIT_FAILURE); } if(lookup(D, k) !=NULL){ fprintf(stderr, "error: key collusion "); exit(EXIT_FAILURE); } if(D->head == NULL){ N = Node(k, v); D->head = N; D->numItems ++; } else{ while(N->next != NULL){ N = N->next; } N->next = Node(k, v); D->numItems ++; } }
void delete(Dictionary D, char* k){ Node N = D->head; if(D==NULL){ fprintf(stderr, "Stack Error: calling delete() on NULL Dictionary reference "); exit(EXIT_FAILURE); } if(lookup(D, k) ==NULL){ fprintf(stderr, "error: key not found "); exit(EXIT_FAILURE); } if(D->numItems key, k) ==0){ Node temp = D->head; D->head = N->next; D->numItems --; freeNode(&temp); } else{ while(strcmp(N->key, k) !=0){ N = N->next; } Node temp = N-> next; N->next = N->next->next; freeNode(&temp); D->numItems --; } } }
void makeEmpty(Dictionary D){ if( D==NULL ){ fprintf(stderr, "Stack Error: calling makeEmpty() on NULL Dictionary reference "); exit(EXIT_FAILURE); } if( D->numItems==0 ){ fprintf(stderr, "Stack Error: calling makeEmpty() on empty Dictionary "); exit(EXIT_FAILURE); } D->numItems = 0; freeNode(&D->head);
}
void printDictionary(FILE* out, Dictionary D){ Node N; if( D==NULL ){ fprintf(stderr, "Stack Error: calling printDictionary() on NULL Dictionary reference "); exit(EXIT_FAILURE); } for(N=D->head; N!=NULL; N=N->next){ fprintf(out, "%s %s ", N->key, N->value); }
}
Expected Output of DictionaryClient.c
Step by Step Solution
There are 3 Steps involved in it
Step: 1
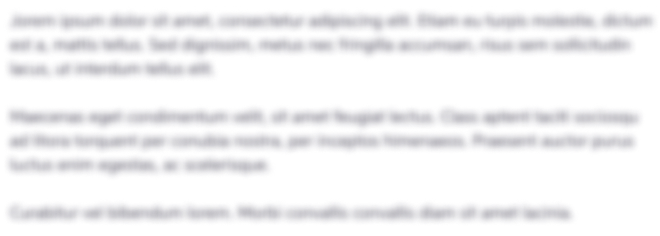
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started