Question
Hi I'm having trouble understanding this fill in the blank coding question, the result is to make the code look like the following output, i
Hi I'm having trouble understanding this fill in the blank coding question, the result is to make the code look like the following output, i will bold the blank spaces -
input -
13030 3 3829249010769 9 4750 3 78032825 6 653026 5 183318152 6 213739 4 316 2 50 2 13030 3 301834 5 19 2 9806 3 12 2
output -
THE SITUATION HAS GOTTEN BLEAK
PLEASE MEET ME BY THE ARENA
AT ONE AM
instructions -
You and your roommate are creating a secret code.
You have a rather simple scheme to express strings of letters as numbers. You realize that since there are only 26 different letters, a string of letters can be viewed as a number in base 26! For example, consider the word
COMPUTER
You realize that we can just assign numeric values to the letters with A = 0, B = 1, C = 2, , Z = 25 and then treat each letter location as the ones place, the 26 place, the 262 place and so forth. Thus, the value of COMPUTERwould be:
2x267 + 14x266 + 12x265 + 15x264 + 20x263+ 19x262 + 4x261 + 17x260.
The "code" for the word computer would be this sum and the number of letters in the word. We will compute this using our "convert" function:
long long convert(char word[], int length);
One process to calculate the necessary sum is something called Horner's method. Instead of calculating any exponents, utilize the following idea for each letter in the string word:
long long value = 0;
int i;
for (i=0; i value = 26*value + (word[i] - 'A'); At the end of this code, value will equal the corresponding calculation shown for computer above. Notice that when we subtract the ASCII value of an uppercase letter from the Ascii value of 'A', we naturally get the appropriate numerical value from 0 to 25. Our program should also be able to decode words encoded using this process. This will be done using our "printText" function: void printText(long long value, int length); To decode a word we can repeatedly mod by 26 and divide by 26 to peel off each letter from the end, one by one. Rather than provide the code, a small example will be illustrated below: Consider the number 1371, knowing the length of the word it encoded was 3: 1371 % 26 = 19 (T), 1371 / 26 = 52 52 % 26 = 0 (A), 52 / 26 = 2 2 % 26 = 2 (C) 2 / 26 = 0 The corresponding message was "CAT", reading the letters from the bottom to the top. Note that to take an integer, x, in between 0 and 25, here is how you can store the corresponding uppercase letter in the character array word, index i: word[i] = (char)(x + 'A'); code - #include #define MAX_LEN 14 long long convert(char word[], int length); void printText(long long value, int length); int main() { int choice; char filename[ MAX_LEN ]; FILE *ifp; printf("Would you like to (1) encode or (2) decode? "); scanf("%d", &choice); if (choice == 1) { //Get input file name. printf("Please enter the input file name, to encode. "); scanf("%s", filename); ifp = fopen(filename, "r"); // Convert each word, one by one. while (fscanf(ifp, "%s", word) != EOF) { len = strlen(word); res = convert(word, len); printf("%lld %d ", res, len); } fclose(ifp); } else { //Get input file name. printf("Please enter the input file name, to decode. "); scanf("%s", filename); ifp = fopen(filename, "r"); // Convert each pair of numbers, one by one. while (fscanf(ifp, "%lld%d", &value, &len) != EOF) { printText(value, len); } fclose(ifp); } return 0; } long long convert(char word[ ], int length) { long long value = [ Select ] ["0", "1", "length", "length-1", "length+1"] ; int i; for(i=0; [ Select ] ["i == length", "i > length", "i >= length", "i <= length", "i < length"] ; i++) value = [ Select ] ["26*value", "26*value + (word[i] - 'A')", "(word[i] - 'A')", "13*value + (word[i] - 'A')", "26*value + (word[i] - 'a')"] ; [ Select ] ["return 0;", "return 1;", "return long long;", "return value;", "return length;", "return word;", "//no statement is needed here"] } void printText(long long value, int length) { char word[ MAX_LEN ]; int i; for(i = [ Select ] ["0", "1", "length", "length-1", "length+1"] ; i>=0; i--) { word[ i ] = ( [ Select ] ["char", "string", "int", "long", "long long"] ) ( [ Select ] ["value*26", "value%26", "value/26", "value+26", "value-26"] + 'A'); value [ Select ] ["+=", "-+", "*=", "/=", "%="] 25; } word[ [ Select ] ["0", "1", "length", "length-1", "length+1"] ] = '\0'; printf("%s ", [ Select ] ["value", "length", "word", "MAX_LEN", ""A""] ); return; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
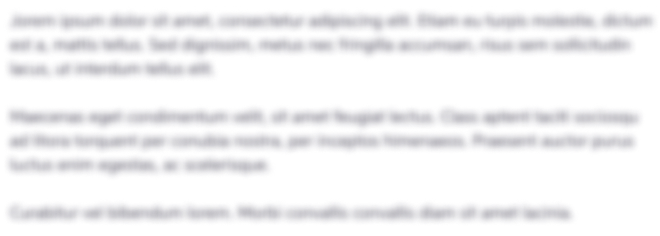
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started