Question
hi im working in java and need help with junit test cases for one of my projects this is my first time working with them.
hi im working in java and need help with junit test cases for one of my projects this is my first time working with them. the project was to create and 8 by 8 tic tac toe board in which you had to get 5 in a row to win. they want us to create test cases for
checkspace(BoardPosition)
Use Path Testing to develop your test cases for checkSpace. Remember that if you have an if condition that uses ands or ors to handle complex conditionals, you must check every possible way that conditional could be handled, not just every way it could evaluate.
placeMarker(BoardPosition)
Use Equivalence and Boundary testing to test placeMarker. Remember that input that goes against your requires clause is not included in any Equivalence classes. Since our requires clause eliminates all BoardPositions that are not on the GameBoard, and all BoardPositions that are unavailable, we only have one equivalence class for our placeMarker function. However, this equivalence class has several boundary test cases. Make a test case for every boundary, and also 2 nonboundary test cases. For each test case make a test method in Junit.
checkForWinner(BoardPosition)
Develop a thorough set of test cases for checkForWinner. You do not need to use any specific method to do so, just make sure you are testing your function thoroughly. Include routine and challenging cases. Remember, you will not be able to test your private functions, so you need to make sure you test them by testing checkForWinner. This means testing for horizontal, vertical and both diagonal wins.
checkForDraw()
This method really only has two possibilities, it either is a tie, or it isnt. Test a couple of cases for the not a tie possibility by picking some routine and interesting cases that could be tricky for the code to catch.
Checking for Equivalence
In order to see if our test cases have passed or failed we need to be able to check for equivalence. However placeMarker does not return a value for us to evaluate. We only know if placeMarker works by checking to see if the marker appears in the 2D array. The 2D array is a private member of GameBoard, so we do not have access to it. How can we check to see if our GameBoard is correct? In order to test for equivalence, you will need to use the GameBoard.toString function. If the marker was placed successfully, you know what should be returned by the toString method. You can create your own 2D array with a marker in the appropriate position, and then use for loops to build the formatted string for that 2D array in the same way that we do inside the toString function. You can then compare this string to the string returned by toString and see if they are the same.
this is my game board class
package cpsc2150.labs.lab10; public class GameBoard { private char[][] table; private final static int TABLE_VOLUME = 8; private final static int TO_WIN = 5; /** * constructor for the board * @ensures that the board is set to a blank space */ GameBoard(){ table = new char[TABLE_VOLUME][TABLE_VOLUME]; for (int i = 0; i < 8; i++) { for (int j = 0; j < 8; j++) { table[i][j] = ' '; } } } /** * checks the position entered by the players * @param pos the marker that wil be checked if that spot is available * @return return whether of not that position is allowed * @requires that the row and column must be in the bounds of 0 to 7 * @ensures that there will be an error message if space is unavailable */ public boolean checkSpace(BoardPosition pos) { return ((pos.getRow() >= 0 && pos.getRow() < TABLE_VOLUME && pos.getCol() >= 0 && pos.getCol() < TABLE_VOLUME) && (table[pos.getRow()][pos.getCol()] == ' ')); } /** * outputs the character on the position asked for by the player * @param marker * @requires that the row and column must be in the bounds of 0 to 7 * @ensures that the position asked for will display the correct character for the player */ public void placeMarker(BoardPosition marker){ table[marker.getRow()][marker.getCol()] = marker.getPlayer(); } /** * to string board * @return a string that displays the tic tac toe table with the blank spaces * @requires the table does not equal null * @ensures a string that represents the board itself */ @Override public String toString() { String str = " "; for(int i = 0; i < TABLE_VOLUME; i++){ str +=i +" "; } str += " "; for(int i = 0; i < TABLE_VOLUME; i++) { str += i + " "; for (int j = 0; j < TABLE_VOLUME; j++) { str += table[i][j] + "|"; } str += " "; } return str; } /** * function checks to see if the lastPos placed resulted in a winner. * @param lastPos the marker that has been placed * @return true if winner , otherwise false. * @requires that the row and column must be in the bounds of 0 to 7 * @ensures a winner if any of the checks have been made */ public boolean checkForWinner (BoardPosition lastPos) { return (checkHorizontalWin(lastPos) || checkVerticalWin(lastPos) || checkLeftDiagonalWin(lastPos)|| checkRightDiagonalWin(lastPos)); } /** * function will check to see if the game has resulted in a tie. * @return true if draw , otherwise false. * @requires that the board is not empty * @ensures a draw if the entire board is filled with no winners */ public boolean checkforDraw() { for (int i = 0; i < 8; i++) { for (int j = 0; j < 8; j++) { if (table[i][j] == ' ') return false; } } return true; } /** * checks to see if the last marker placed resulted in 5 in a row horizontally. * @param lastPos the marker that has been placed * @return true if it does, otherwise false * @requires that the row and column must be in the bounds of 0 to 7 * @ensures a horizontal win if the there is five in a row of the same character either 'X' or '0' */ private boolean checkHorizontalWin(BoardPosition lastPos){ int count = 0; int minRow = 0; int maxRow = 7; if((lastPos.getRow() - 7 ) > minRow) minRow = lastPos.getRow() - 7; for(int i = minRow; i <= maxRow; i++){ if(table[lastPos.getRow()][i] == lastPos.getPlayer()){ count++; if(count == TO_WIN) return true; } else count = 0; } return false; } /** * checks to see if the last marker placed resulted in 5 in a row vertically. * @param lastPos the marker that has been placed * @return true if it does, otherwise false * @requires that the row and column must be in the bounds of 0 to 7 * @ensures a vertical win if the there is five in a row of the same character either 'X' or '0' */ private boolean checkVerticalWin(BoardPosition lastPos){ int count = 0; int minCol = 0; int maxCol = 7; if((lastPos.getCol() - 4) > minCol){ minCol = lastPos.getCol() - 4;} for(int i = minCol; i <= maxCol; i++){ if(table[i][lastPos.getCol()] == lastPos.getPlayer()){ count++; if(count == TO_WIN){ return true;} } else count = 0; } return false; } /** * checks to see if the last marker placed resulted in 5 in a row from left to right diagonally. * @param lastPos the marker that has been placed * @return true if it does, otherwise false * @requires that the row and column must be in the bounds of 0 to 7 * @ensures a diagonal win if the there is five in a row of the same character either 'X' or '0' */ private boolean checkLeftDiagonalWin(BoardPosition lastPos){ int i, j; int count = 0; int minRow = 0; int minCol = 0; int maxRow = 7; int maxCol = 7; if(lastPos.getRow() > lastPos.getCol()){ minRow = lastPos.getRow() - lastPos.getCol();} if(lastPos.getCol() > lastPos.getRow()){ minCol = lastPos.getCol() - lastPos.getRow();} if(lastPos.getRow() < lastPos.getCol()){ maxRow = lastPos.getRow() +(maxCol - lastPos.getCol());} if(lastPos.getCol() < lastPos.getRow()){ maxCol = lastPos.getCol() + (maxRow - lastPos.getRow());} if((minRow <= (3)) && (minCol <= (3))) { for (i = minRow; i <= maxRow; i++) { for(j = minCol; j <= maxCol; j++){ if (table[i][j] == lastPos.getPlayer()) { count++; if (count == TO_WIN) return true; } else count = 0; } } } return false; } /** * checks to see if the last marker placed resulted in 5 in a row from left to right reversed diagonally. * @param lastPos the marker that has been placed * @return true if it does, otherwise false * @requires that the row and column must be in the bounds of 0 to 7 * @ensures a diagonal win if the there is five in a row of the same character either 'X' or '0' */ private boolean checkRightDiagonalWin(BoardPosition lastPos){ int i, j; int count = 0; int minRow = 0; int minCol = 0; int maxRow = 7; int maxCol = 7; if((lastPos.getRow() + lastPos.getCol()) > maxCol) { minRow = lastPos.getRow() - (maxCol - lastPos.getCol()); minCol = minRow; } if((lastPos.getRow() + lastPos.getCol()) < maxRow) { maxRow = lastPos.getRow() + lastPos.getCol(); maxCol = maxRow;} if((maxRow >= (3)) && (minRow <= (3))) { for (i = maxRow; i >= minRow; i--) { for( j = minCol; j <= maxCol; j++){ if (table[i][j] == lastPos.getPlayer()) { count++; if (count == TO_WIN) return true; } else count = 0; } } } return false; } /** * checks to see if the last marker placed resulted in 5 in a row from right to left reversed diagonally. * @param lastPos the marker that has been placed * @return true if it does, otherwise false * @requires that the row and column must be in the bounds of 0 to 7 * @ensures a diagonal win if the there is five in a row of the same character either 'X' or '0' */ /** * @param other game board that will be checked for equality * @return whether the game boards are equal * @requires that both boards are not null * @ensures both are equal to one another */ public boolean equals(GameBoard other) { int count = 0; for (int i = 0; i < 8; i++) { for (int j = 0; j < 8; j++) { if (table[i][j] == other.table[i][j]) count++; } } return (count == (64)); } }
this is my boardPosition class:
package cpsc2150.labs.lab10; public class BoardPosition { private int row; private int column; private char player; /** * constructor * @param r represents row * @param c represents column * @param p represents player X or O * @requires that both row and column need to represent valid numbers and that player is not a null * @ensures that the assigned variables represent one another */ BoardPosition(int r, int c, char p){ row = r; column = c; player = p; } /** * getter * @return row location * @ensures row will be getRow() */ public int getRow(){ return row; } /** * getter * @return column location * @ensures column will be geCol() */ public int getCol(){ return column; } /** * getter * @return player character * @ensures player will be getPlayer() */ public char getPlayer(){ return player; } /** * getter * @return * @requires that the row and column must be in the bounds of 0 to 7 * @ensures */ @Override public String toString(){ String str = " "; str += "Player" + player + "at position" + row + ", " + column; return str; } /** * @param next * @return * @requires that the row and column must be in the bounds of 0 to 7 * @ensures */ public boolean equals(BoardPosition next){ return((row == next.row)&& (column == next.column) && (player == next.player)); } }
this is my GameScreen class:
package cpsc2150.labs.lab10; import java.util.*; public class GameScreen { public static void main(String[] args){ char startPlayer = 'X'; GameBoard newBoard = new GameBoard(); while(!endMatch){ System.out.println(newBoard.toString()); playersTurn(startPlayer, newBoard); startPlayer = changePlayer(startPlayer); } while(playAgain){ startPlayer = 'X'; endMatch = false; newBoard = new GameBoard(); while(!endMatch){ System.out.println(newBoard.toString()); playersTurn(startPlayer, newBoard); startPlayer = changePlayer(startPlayer); } } } private static boolean endMatch = false; private static boolean playAgain = false; private static char changePlayer(char p){ if(p == 'X') return 'O'; else return 'X'; } private static void playersTurn(char player, GameBoard g){ int row, column; String errorMessage = "That space is either out of bounds or taken, please pick again"; String enterRow = "Player " + player + " enter your row number: "; String enterCol = "Player " + player + " enter your column number: "; String winner = "Player " + player + " is the winner!!!"; String draw = "Its a draw!!!"; String playAgainMessage= "Would you like to play again? Y/N"; Scanner scanner = new Scanner(System.in); System.out.print(enterRow); row = scanner.nextInt(); System.out.print(enterCol); column = scanner.nextInt(); BoardPosition opponent = new BoardPosition(row, column, player); while(!g.checkSpace(opponent)){ System.out.println(g.toString()); System.out.println(errorMessage); System.out.print(enterRow); row = scanner.nextInt(); System.out.print(enterCol); column = scanner.nextInt(); opponent = new BoardPosition(row, column, player); } g.placeMarker(opponent); if (g.checkForWinner(opponent) || g.checkforDraw()) { String input; if (g.checkForWinner(opponent)) System.out.println(winner); if (g.checkforDraw()) System.out.println(draw); System.out.println(playAgainMessage); input = scanner.next(); playAgain = (input.equals("Y") || input.equals("y")); endMatch = true; } } }
this is my test cases class that im trying to work on/understand
import cpsc2150.labs.lab10.GameBoard; import org.junit.Test; import static org.junit.Assert.*;
public class TestGameBoard { private GameBoard newBoard; /************************************************************ Test checkSpace ************************************************************/ @Test public void testcheckSpace_row1_column10() { int row = 1; int column = 10; assertTrue(!); } @Test public void testcheckSpace_row16_column2() { int row = 16; int column = 2; } @Test public void testcheckSpace_row16_column10() { int row = 16; int column = 10; } @Test public void testcheckSpace_row1_columnNegative10() { int row = 1; int column = -10; } @Test public void testcheckSpace_rowNegative16_column2() { int row = -16; int column = 2; } @Test public void testcheckSpace_rowNegative16_columnNegative10() { int row = -16; int column = -10; } @Test public void testcheckSpace_row7_column7() { int row = 7; int column =7; } /************************************************************ Test placeMarker ************************************************************/ /************************************************************ Test checkforWinner ************************************************************/ @Test public void testcheckforWinner_row0() { } @Test public void testcheckforWinner_row1() { } @Test public void testcheckforWinner_row2() { } @Test public void testcheckforWinner_row3() { } @Test public void testcheckforWinner_row4() { } @Test public void testcheckforWinner_row5() { } @Test public void testcheckforWinner_row6() { } @Test public void testcheckforWinner_row7() { } @Test public void testcheckforWinner_column0() { } @Test public void testcheckforWinner_column1() { } @Test public void testcheckforWinner_column2() { } @Test public void testcheckforWinner_column3() { } @Test public void testcheckforWinner_column4() { } @Test public void testcheckforWinner_column5() { } @Test public void testcheckforWinner_column6() { } @Test public void testcheckforWinner_column7() { } /************************************************************ Test checkforDraw ************************************************************/ private String loanToString(int row, int column, char player) { String str = ""; /*str += str += str += str +=*/ return str; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
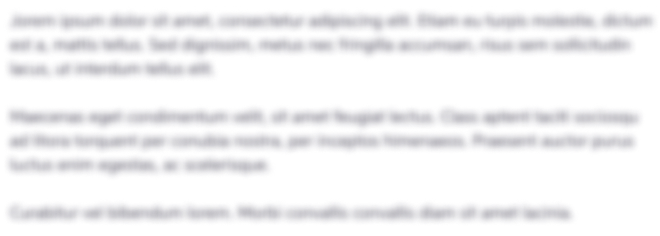
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started