Question
Hi, I'm working my way through a project that would be similar to a bank simulation. I've to use a RandomAccessFile to store records of
Hi,
I'm working my way through a project that would be similar to a bank simulation. I've to use a RandomAccessFile to store records of bank accounts. I've created a class to create the accounts and write them to the file and I have a class to read the account and display the account details. The class I've copied below also works for deleting an account. I'm now stuck at trying to figure out how I could make withdrawls or deposits to an account by accepting in an amount from the user and then adding or subtracting this from the balance field in the record.
Hope this nakes sense
Regards
Alex
//this class will allow the user to read data for a particular account
//and delete the account
import java.io.*;
import java.awt.*;
import javax.swing.*;
import java.awt.event.*;
import java.text.DecimalFormat;
public class CreditUnionClose extends JFrame implements ActionListener
{
//create GUI components
private JTextField idField, firstNameField, lastNameField, balanceField, overdraftField;
private JButton closeBut, exitBut;
private RandomAccessFile input, output;
private Record data;
public CreditUnionClose()
{
super ("Close Account");
try
{
//set up files for read & write
input = new RandomAccessFile( "credit1.dat", "rw" );
output = new RandomAccessFile( "credit1.dat", "rw" );
}
catch ( IOException e )
{
System.err.println(e.toString() );
System.exit(1);
}
data = new Record();
//create the components of the Frame
setSize( 600, 300 );
setLayout( new GridLayout(6, 2) );
add( new JLabel( "Account ID Number (1 - 100)" ) );
idField = new JTextField(15);
idField.setEditable( true );
add( idField );
idField.addActionListener(this);
add( new JLabel( "First Name" ) );
firstNameField = new JTextField(15);
firstNameField.setEditable( false );
add( firstNameField );
add( new JLabel( "Last Name" ) );
lastNameField = new JTextField(15);
lastNameField.setEditable( false );
add( lastNameField );
add( new JLabel( "Account Balance" ) );
balanceField = new JTextField(15);
balanceField.setEditable( false );
add( balanceField );
add( new JLabel( "Overdraft Facility" ) );
overdraftField = new JTextField(15);
overdraftField.setEditable( false );
add( overdraftField);
closeBut = new JButton( "Close Account");
closeBut.addActionListener( this );
add(closeBut);
closeBut.setEnabled(false);
exitBut = new JButton( "Exit" );
exitBut.addActionListener( this );
add(exitBut);
setVisible( true );
}
public void actionPerformed( ActionEvent e )
{
//read account details when account number entered
if (! idField.getText().equals(""))
{
readRecord(); //calling readRecord method
closeBut.setEnabled(true);
}
if (e.getSource() == exitBut) //exit maintenance menu
{
setVisible(false);
}
if (e.getSource() == closeBut)
{
try
{
int accountNumber = Integer.parseInt( idField.getText() );
data.setID( 0 );
data.setFirstName( null );
data.setLastName( null);
data.setBalance( 0);
data.setOverdraft( 0);
output.seek( (long) ( accountNumber-1 ) * Record.size() );
data.write( output );
JOptionPane.showMessageDialog(this, "Account has been deleted");
idField.setText("");
firstNameField.setText("");
lastNameField.setText("");
balanceField.setText("");
overdraftField.setText("");
}//end try
catch (NumberFormatException nfe )
{
System.err.println("You must enter an integer account number");
}
catch (IOException io)
{
System.err.println("error during write to file " + io.toString() );
}
}
} //end actionPerformed
public void readRecord()
{
DecimalFormat twoDigits = new DecimalFormat( "0.00" );
try
{
int accountNumber = Integer.parseInt(idField.getText());
if (accountNumber < 1 || accountNumber > 100)
{
JOptionPane.showMessageDialog(this, "Account does not exist");
}
else
{
input.seek( (accountNumber - 1)*Record.size());
data.read(input);
idField.setText(String.valueOf( data.getID() ) );
firstNameField.setText( data.getFirstName() );
lastNameField.setText( data.getLastName() );
balanceField.setText( String.valueOf( twoDigits.format( data.getBalance() ) ) );
overdraftField.setText( String.valueOf(twoDigits.format( data.getOverdraft() ) ) );
}
if (data.getID() == 0)
{
JOptionPane.showMessageDialog(this, "Account does not exist");
idField.setText("");
}
if (data.getBalance() != 0)
{
JOptionPane.showMessageDialog(this, "Balance needs to be Zero before Account can be removed");
idField.setText("");
}
}//end try statement
catch (EOFException eof )
{
closeFile();
}
catch (IOException e )
{
System.err.println("Error during read from file " + e.toString() );
System.exit( 1 );
}
} // end readRecord method
private void closeFile()
{
try
{
input.close();
System.exit( 0 );
}
catch( IOException e)
{
System.err.println( "Error closing file " + e.toString() );
}
}// end closeFile method
public static void main(String [] args)
{
new CreditUnionClose();
}
}
*******************************************************************************************************************************************
UPDATE
*********************************************************************************************************************************************
*********RAF CREATION*********************************************
// This section will create a random access file and // will write 100 empty records to file.
import java.io.*;
public class CreateRandomFile { private Record blank; private RandomAccessFile file;
public CreateRandomFile()
{ blank = new Record();
try {
file = new RandomAccessFile( "credit1.dat", "rw" );
for (int i=0; i<100; i++) blank.write( file );
}
catch(IOException e)
{
System.err.println("File not opened properly " + e.toString() );
System.exit( 1 );
} }
public static void main( String [] args )
{ CreateRandomFile accounts = new CreateRandomFile(); }
}
*************************************************************************************************************************************************
********RECORD***************************************************
//Initial program for creation of file to contain various accounts
import java.io.*;
import javax.swing.*;
public class Record
{
private int accountid;
private String firstName;
private String lastName;
private double balance;
private double overdraft;
public void read( RandomAccessFile file ) throws IOException
{
accountid = file.readInt();
char first[] = new char[15];
for ( int i=0; i < first.length; i++ )
first[ i ] = file.readChar();
firstName = new String (first);
char last[] = new char[15];
for (int i =0; i last[i] = file.readChar(); lastName = new String (last); balance = file.readDouble(); overdraft = file.readDouble(); } public void write( RandomAccessFile file) throws IOException { StringBuffer buf; file.writeInt( accountid ); if (firstName != null) buf = new StringBuffer( firstName); else buf = new StringBuffer( 15 ); buf.setLength( 15 ); file.writeChars( buf.toString() ); if ( lastName != null ) buf = new StringBuffer( lastName ); else buf = new StringBuffer( 15); buf.setLength( 15 ); file.writeChars( buf.toString() ); file.writeDouble( balance ); file.writeDouble( overdraft); } public void setID( int a ) { accountid = a; } public int getID() { return accountid;} public void setFirstName( String f) {firstName = f;} public String getFirstName() { return firstName; } public void setLastName ( String l) { lastName = l; } public String getLastName() { return lastName; } public void setBalance( double b) {balance = b;} public double getBalance() {return balance;} public void setOverdraft( double o) {overdraft = o;} public double getOverdraft() {return overdraft;} //determines size (bytes) of each file public static int size() { return 80;} }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
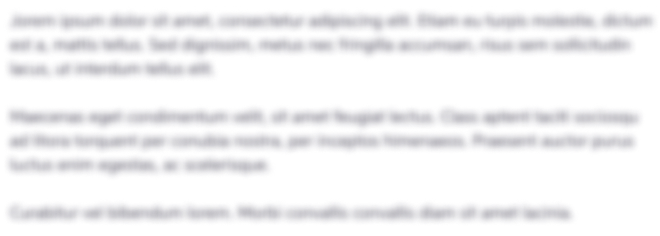
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started