Question
Hi please make sure the answer is right before you post the answer, The first two pictures are the one question and the rest of
Hi please make sure the answer is right before you post the answer, The first two pictures are the one question and the rest of the pics are info you need to do the problem correctly!!!
Driver.java
package com.company;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.PrintWriter;
import java.util.Scanner;
/**
* Created by naseef on 5/16/18.
*/
public class Driver {
public static void main(String[] args) {
Grades gradesA = initialize("inputHW9A.txt");
Grades gradesB = initialize("inputHW9B.txt");
Grades gradesC = initialize("inputHW9C.txt");
Grades repeat = initialize("inputRepeat.txt");
Grades mergedGrades = merge(gradesA, gradesB, gradesC);
Grades sortedGrades = sort(mergedGrades, repeat);
flush(sortedGrades);
}
public static Grades initialize(String section) {
// PLEASE IMPLEMENT THIS METHOD.
return null;
}
public static Grades merge(Grades gradesA, Grades gradesB, Grades
gradesC) {
Grades mergedGrades = new Grades();
// Identify which method in the Grades class can you use to take
these three Grades and merge them
return mergedGrades;
}
public static Grades sort(Grades mergedGrades, Grades repeat) {
// PLEASE COMPLETE THE METHOD USING THE FOLLOWING LOGIC
// 1: Create a an empty Grades list.
Grades sortedGrades = new Grades();
// 2: Loop through the mergedGrades list and find student with the
highest grade.
// 3: Add this to the sortedGrades list ( use Grades::add Method )
// 4: Remove it from the mergedGrades list ( use Grades::remove
Method)
// 5: Do this till mergedGrades list becomes empty
// If you find collision (two students with the same grade ) this
is how you will resolve it
// 1: if the student has repeated the course, then he will be
moved to the end ( within the GPA block )
// 2: If both students have repeated, or both have not repeated,
but their GPA is the same then sort them by First Name
// 3: If the first name is same, then sort by last name
return sortedGrades;
}
public static void flush(Grades sortedGrades) {
try {
PrintWriter writer = new PrintWriter("outputHW8.txt");
writer.write(sortedGrades.toString()); // Notice how I am
calling toString on Grades class
// Grades toString
calls toString on Grade class that you need to implement.
writer.close();
} catch (FileNotFoundException e) {
}
}
}
Grade.java
package com.company;
/**
* Created by naseef on 5/16/18.
*/
public class Grade {
private String id;
private double gpa;
private String firstName;
private String lastName;
// Please implement the following
// 1: Constructor that takes String id, double gpa, String firstName,
String lastName
// 2: Constructor that takes String id, double gpa, String firstName.
Sets Last Name to NLN
// Getters to get GPA, firstName and LastName. You will use it in the
sort Method in driver class.
// Override the equal method.
// You use it in the sort method in the Driver class to check if a
particular GradeObject exists in the repeat list
// Override the toString method.
// flush method in driver calls toString for Grades class.
// The Grades class has implemented toString override.
// It internally calls Grade::toString.
// So you need to implement this method so that the file is printed
correctly
// In addition, you will need the below two methods
public boolean isLessGPA(Grade grade) {
// implement logic
return false;
}
public boolean isSameGPA(Grade grade) {
// implement logic
return false;
}
}
Grades.java
package com.company;
/**
* Created by naseef on 5/21/18.
*/
public class Grades {
private int capacity;
private int size;
private Grade[] gradeArray;
public final int OFFSET = 4;
public Grades() {
this(4);
}
public Grades(int capacity) {
this.capacity = capacity;
this.gradeArray = new Grade[capacity];
this.size = 0;
}
public void add(Grade grade) {
if(size
gradeArray[size++] = grade;
} else {
this.capacity += OFFSET;
Grade[] temp = new Grade[this.capacity];
for(int i = 0; i
temp[i] = gradeArray[i];
}
temp[size++] = grade;
this.gradeArray = temp;
}
}
public void add(Grades grades) {
for(int i = 0; i
add(grades.get(i));
}
}
public void remove(int index) {
for(int i = index; i
this.gradeArray[i] = this.gradeArray[i+1];
}
this.gradeArray[size-1] = null;
this.size --;
}
public Grade get(int index) {
return gradeArray[index];
}
public int size() {
return this.size;
}
public boolean contains(Grade grade) {
for(int i = 0; i
if(this.gradeArray[i].equals(grade)) {
return true ;
}
}
return false;
}
@Override
public String toString() {
String data = "" ;
for(int i = 0; i
data += gradeArray[i].toString();
data += " ";
}
return data;
}
}
Inputhw9A.txt
S001 3.3 John Rodgers
S002 3.9 Jim
S003 3.9 Misty Fang
S004 3.9 Aseef Hernandez
S005 4.0 Stacy Lu
S006 3.9 Aseef Nilkund
S009 3.9 Steve Calderon
S010 3.8 Raj Singh
Inputhw9B.txt
S011 3.3 Jason Kramer
S012 3.5 Kathy Calderon
S013 3.2 Roopa Singh
S014 2.4 Amid Naveed
S015 1.0 Faith Williams
S016 3.9 Aseef Simmons
Inputhw9C.txt
S017 3.3 Hifza Nilkund
S018 3.5 Hamza Nilkund
S019 3.2 Chris Peach
S020 2.4 Ramona Luke
InputRepeat.txt
S001 3.3 John Rodgers
S013 3.2 Roopa Singh
S014 2.4 Amid Naveed
S016 3.9 Aseef Simmons
Outputhw8.txt
S005: Stacy, Lu 4.0
S004: Aseef, Hernandez 3.9
S006: Aseef, Nilkund 3.9
S002: Jim, NLN 3.9
S003: Misty, Fang 3.9
S009: Steve, Calderon 3.9
S016: Aseef, Simmons 3.9
S010: Raj, Singh 3.8
S018: Hamza, Nilkund 3.5
S012: Kathy, Calderon 3.5
S017: Hifza, Nilkund 3.3
S011: Jason, Kramer 3.3
S001: John, Rodgers 3.3
S019: Chris, Peach 3.2
S013: Roopa, Singh 3.2
S020: Ramona, Luke 2.4
S014: Amid, Naveed 2.4
S015: Faith, Williams 1.0
Step by Step Solution
There are 3 Steps involved in it
Step: 1
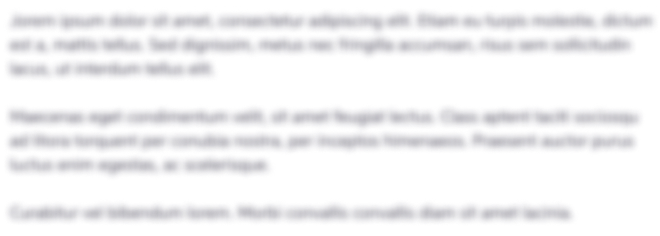
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started