Question
Hi! So I'm having trouble with this problem: You will notice in Activity 3 that there are several files that have been added to the
Hi! So I'm having trouble with this problem:
You will notice in Activity 3 that there are several files that have been added to the editor. Each of these files is responsible in part for creating a GUI version of the Celebrity Game. GUI, or Graphic User Interface, is simply a way for us to interact with programs!
The files StartPanel, CelebrityPanel, and CelebrityFrame are all responsible for creating an interactive environment for you to play the Celebrity game. You wont have to do anything to these files in order to get the code to work, but feel free to explore them to try to understand how the code is running in the console.
In this exercise and the following ones, we are going to make changes to the files responsible for starting the Celebrity Game using a graphics program. For this exercise, we are going to make edits to the CelebrityGame.java file. All of the code described in this activity will be added to CelebrityGame.java, unless explicitly stated otherwise.
Setting up Instance Variables:
-
In the declaration section add an instance variable named celebGameList that is an ArrayList of Celebrity objects. What visibility should this variable have? Write the statement to declare the celebGameList instance variable with the appropriate visibility and add this statement to the CelebrityGame class.
-
You also need an instance variable named gameCelebrity for the current Celebrity that is being used in the game. Will its visibility match celebGameList? If not, what should its visibility be?
-
In the declaration section add an instance variable named gameWindow that is a CelebrityFrame object. In the constructor initialize the CelebrityFrame using the line:
gameWindow = new CelebrityFrame(this);
The this keyword is used to provide a reference to the current game to the GUI window so it can be accessed from the GUI components. This GUI will not be used with multiple games, rather it will use the reference to the current game instance.
Setting up the constructor:
- In the CelebrityGame class, make sure the celebGameList is instantiated appropriately in the CelebrityGame constructor.
Important Note: This exercise will not run in the console, as there is no main method to run. This code will be used in future exercises, so make sure to test that it works in those exercises!
The code there is so far:
import java.util.ArrayList;
/** * The framework for the Celebrity Game project * */ public class CelebrityGame { /** * A reference to a Celebrity or subclass instance. */
/** * The GUI frame for the Celebrity game. */
/** * The ArrayList of Celebrity values that make up the game */
/** * Builds the game and starts the GUI */ public CelebrityGame() { }
/** * Prepares the game to start by re-initializing the celebGameList and having the gameFrame open the start screen. */ public void prepareGame() { }
/** * Determines if the supplied guess is correct. * * @param guess * The supplied String * @return Whether it matches regardless of case or extraneous external * spaces. */ public boolean processGuess(String guess) { return false; }
/** * Asserts that the list is initialized and contains at least one Celebrity. * Sets the current celebrity as the first item in the list. Opens the game * play screen. */ public void play() { }
/** * Adds a Celebrity of specified type to the game list * * @param name * The name of the celebrity * @param guess * The clue(s) for the celebrity * @param type * What type of celebrity */ public void addCelebrity(String name, String guess, String type) { }
/** * Validates the name of the celebrity. It must have at least 4 characters. * @param name The name of the Celebrity * @return If the supplied Celebrity is valid */ public boolean validateCelebrity(String name) { return false; }
/** * Checks that the supplied clue has at least 10 characters or is a series of clues * This method would be expanded based on your subclass of Celebrity. * @param clue The text of the clue(s) * @param type Supports a subclass of Celebrity * @return If the clue is valid. */ public boolean validateClue(String clue, String type) { return false; }
/** * Accessor method for the current size of the list of celebrities * * @return Remaining number of celebrities */ public int getCelebrityGameSize() { return 0; }
/** * Accessor method for the games clue to maintain low coupling between * classes * * @return The String clue from the current celebrity. */ public String sendClue() { return null; }
/** * Accessor method for the games answer to maintain low coupling between * classes * * @return The String answer from the current celebrity. */ public String sendAnswer() { return null; } }
Part of screenshot of code (just for reference):
Step by Step Solution
There are 3 Steps involved in it
Step: 1
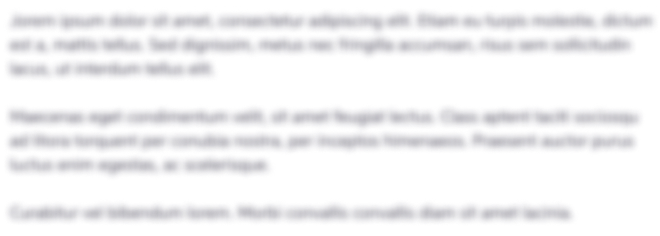
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started