Question
Hi there! I have a task where the finished program should have two simple classes: Date and Book. The first of these describes the date
Hi there!
I have a task where the finished program should have two simple classes: Date and Book. The first of these describes the date and is already implemented. However, it is not meant to be used outside of the class and is therefore introduced in the private section.
What remains for the implementation is the class Book, which describes a book in the library. For each book, the name and author are known, as well as whether the book is on loan or not. If the book is on loan, the loan date and return date (due date) are also known.
The book can be borrowed (if it is not already borrowed), it can also be renewed (if it has been borrowed before) and returned. If borrowing is successful, the borrowing method creates a borrowing date for the book (given as a parameter) and a due date, which is 28 days from the borrowing date. If the renewal is successful, the renewal method moves the due date forward by 28 days. (Note that here the renewal works differently than in the real library. In the real library renewal, the new due date is counted forward from the renewal date, but here it is counted from the old due date.)
Additionally, book information can be printed in the format author:name. If the book is not on loan, the print method prints the text available. If the book is on loan, the print method prints when the book has been borrowed and when it must be returned. A more detailed print outfit can be seen in the example below.
The codebase is given below. It shows how the Bookmethods of the class have been called. The task is to implement a class Book that contains information about an individual book and all methods that are called in the main program. Only one book entity appears in the main program, but there could be more.
It is not necessary to modify the Date class, but it is needed in the implementation of the Book class. In addition, you can see a model of what the header and implementation files of the class look like.
After the class Book is implemented, the program works with the given main program as follows:
Here is the Date:
#include "date.hh" #include
unsigned int const month_sizes[12] = { 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31 };
Date::Date(): day_(1), month_(1), year_(1) { }
Date::Date(unsigned int day, unsigned int month, unsigned int year): day_(day), month_(month), year_(year) { if( month_ > 12 || month_ month_sizes[month_ - 1] || ( month_ == 2 && is_leap_year() && day > month_sizes[month - 1] + 1 ) ) { day_ = 1; } }
Date::~Date() { }
void Date::advance(unsigned int days) { day_ += days; while ( day_ > month_sizes[month_ - 1] ) { if ( month_ == 2 && day_ == 29 ) { return; } day_ -= month_sizes[month_ - 1]; if ( month_ == 2 && is_leap_year() ) { --day_; } ++month_;
if ( month_ > 12 ) { month_ -= 12; ++year_; } } }
void Date::print() const { if(day_
bool Date::is_leap_year() const { return (year_ % 4 == 0) && (!(year_ % 100 == 0) || (year_ % 400 == 0)); }
And here is the main function:
#include "date.hh" #include "book.hh" #include
using namespace std;
int main() { // Creating a date Date today(5, 5, 2020);
// Creating a book Book book1("Kivi", "Seitseman veljesta"); book1.print();
// Loaning a book book1.loan(today);
// Two weeks later today.advance(14);
// Trying to loan a loaned book book1.loan(today); book1.print();
// Renewing a book book1.renew(); book1.print();
// Returning the book book1.give_back();
// Trying to renew an available book book1.renew(); book1.print();
// Loaning again (two weeks later) book1.loan(today); book1.print();
return 0; }
Kivi : Seitseman veljesta - available Already loaned: cannot be loane Kivi : Seitseman veljesta - loaned: 05.05.2020 - to be returned: 02.06.2020 Kivi : Seitseman veljesta - loaned: 05.05.2020 - to be returned: 30.06.2020 Not loaned: cannot be renewed Kivi : Seitseman veljesta - available Kivi : Seitseman veljesta - loaned: 19.05.2020 - to be returned: 16.06.2020
Step by Step Solution
There are 3 Steps involved in it
Step: 1
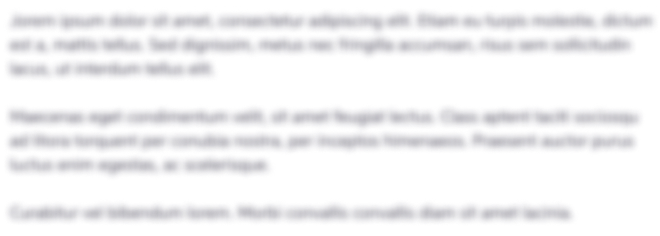
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started