Hi there! Please check the public E get and public E set methods in the third screenshot. Tell me if I implemented them correctly and how I can check if they are working correctly in the second last screenshot or in the driver's class. Basically, the task is to implement both of those methods which I do not know how to implement.
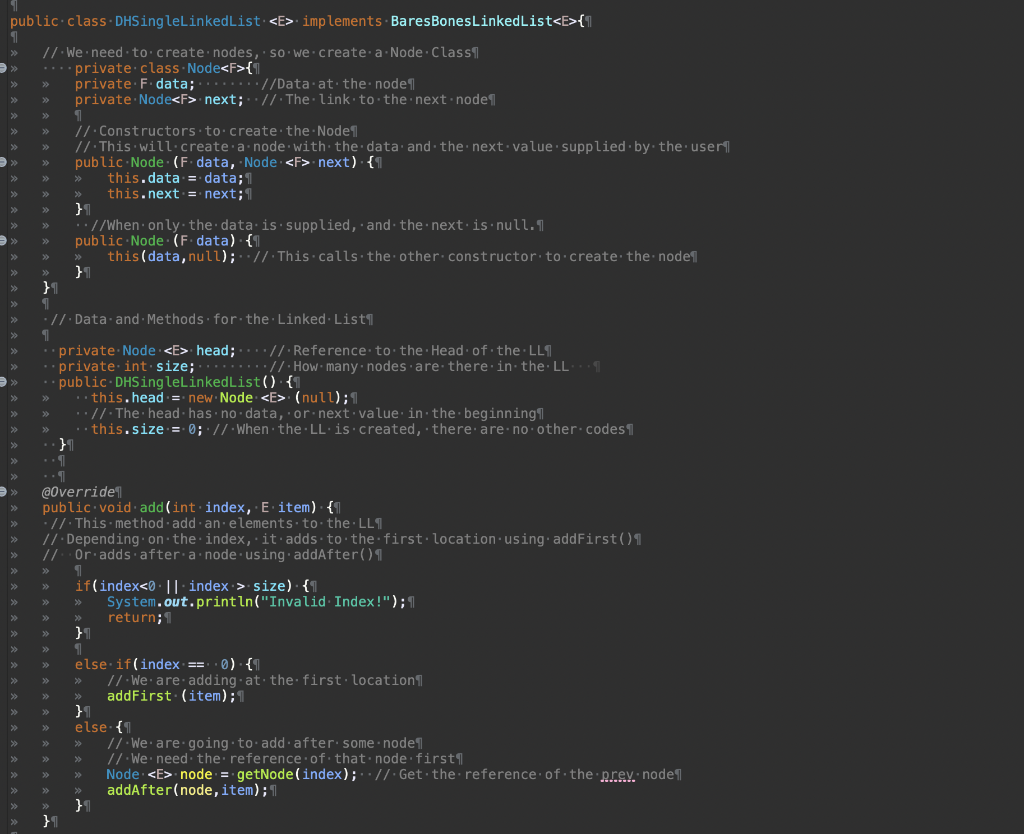
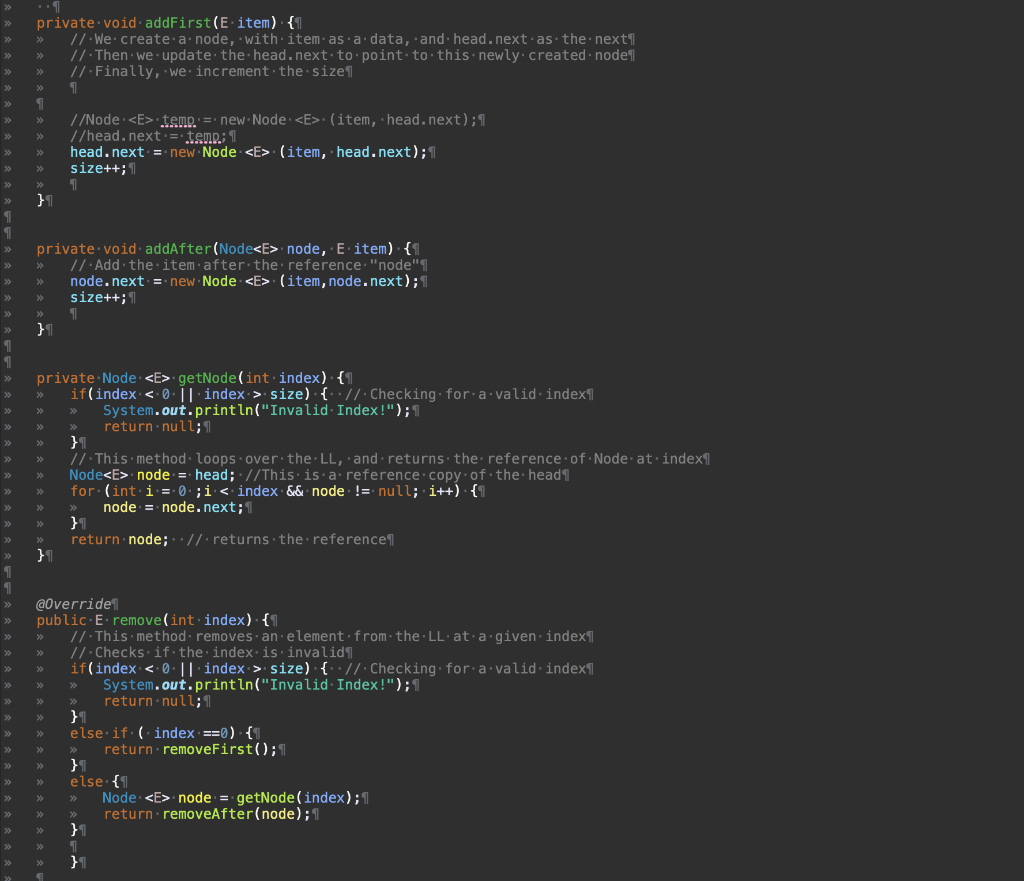
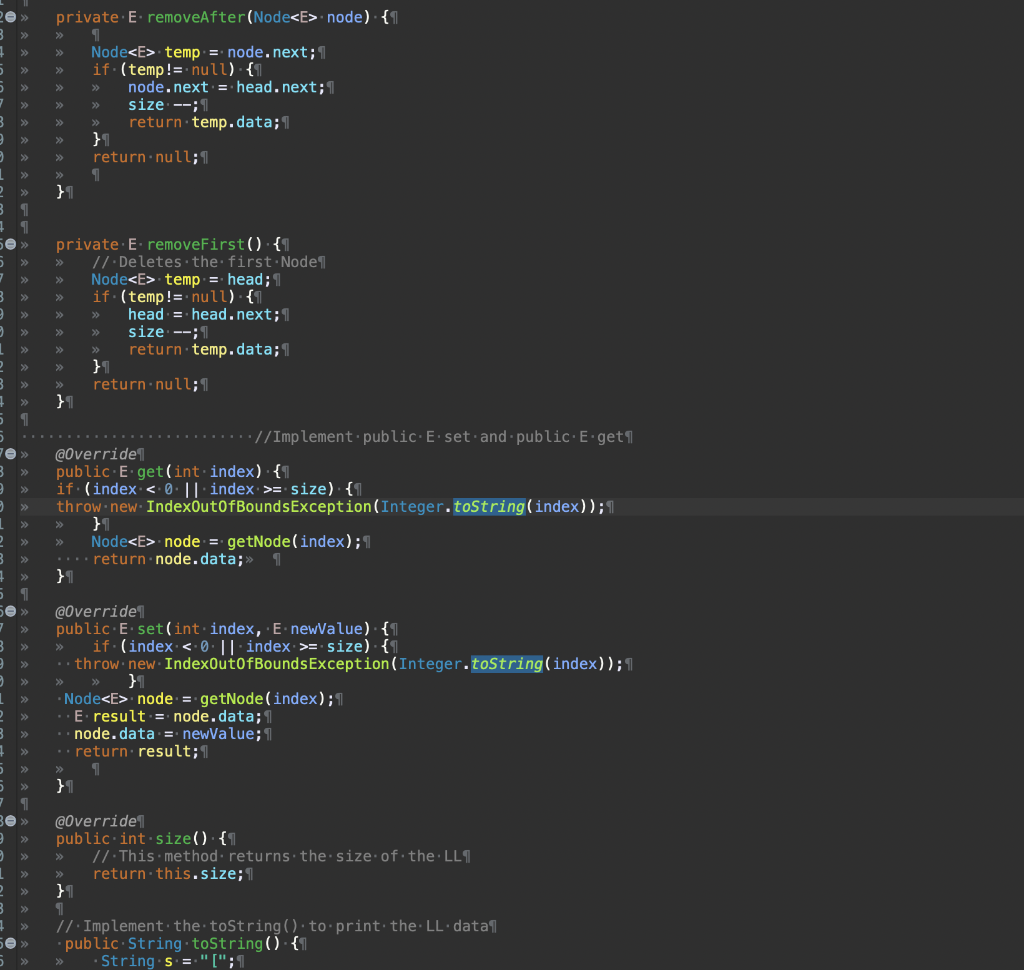
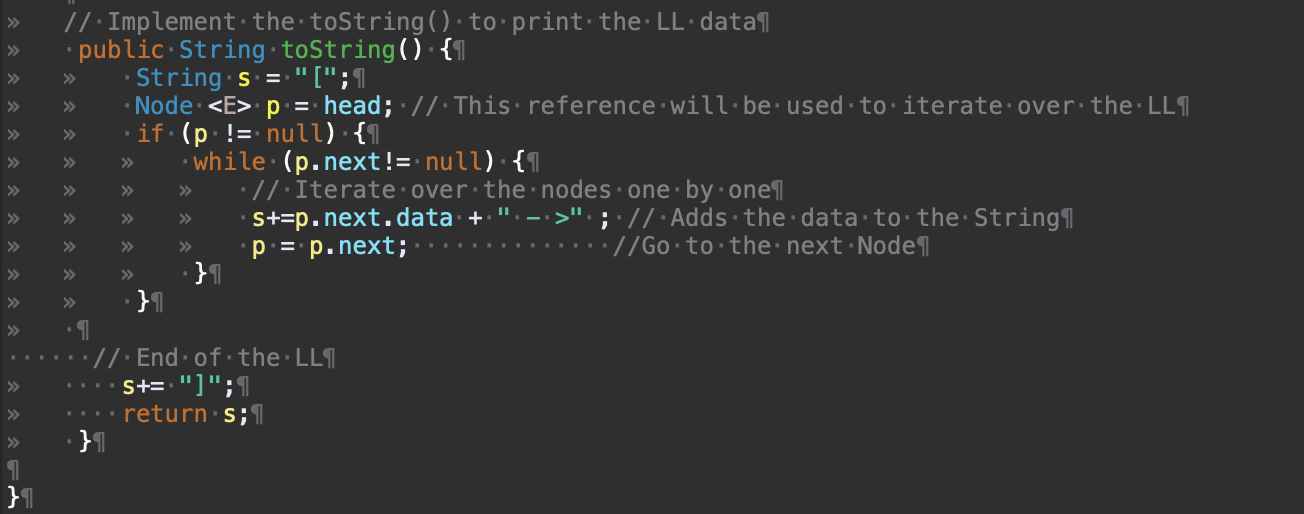
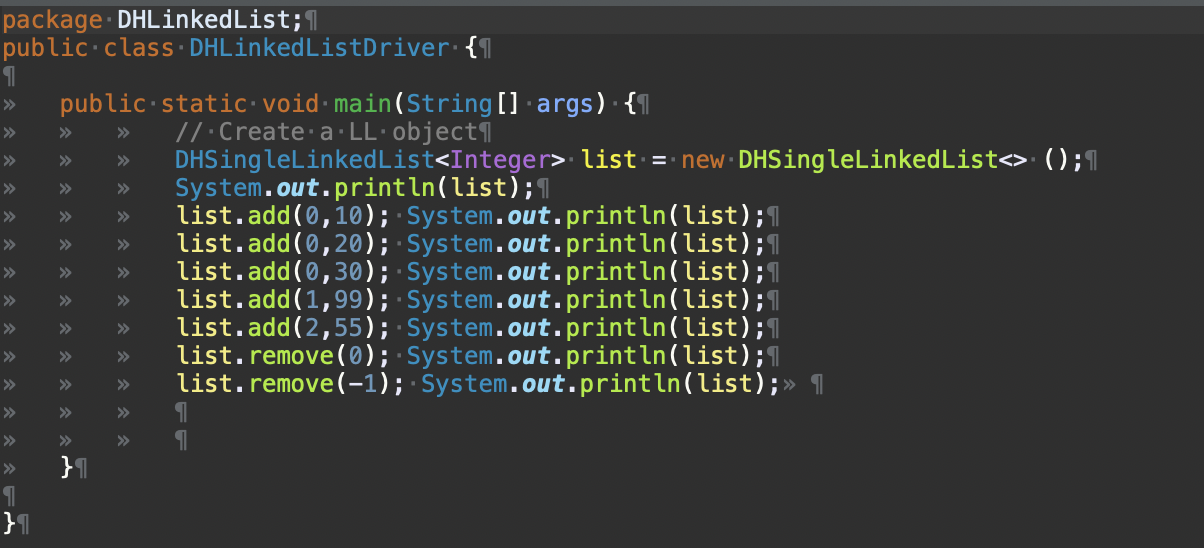
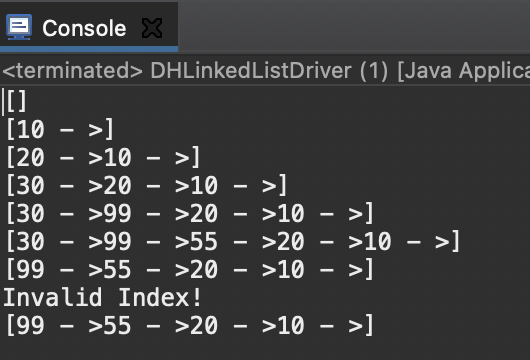
public class DHSingleLinkedList:
implements BaresBonesLinkedList{1 // We need to create nodes, so we create. a.Node. Class ...private class. Node{\ private. Fidata;........//Data at the node private.Node next; // The link to the next.node 11. Constructors to create the. Nodel // This will create a node with the data and the next value: supplied by the users public.Node: (F. data, Node: next) { this.data = data; this.next = next; } //When only. the data is supplied, and the next.is null.1 public.Node. (F.data) {1 this(data, null); // This calls the other constructor to create the nodel } // Data and Methods for the Linked. Listy private.Node head; // Reference to the Head of the LLI - private int size; // How many nodes are there in the LL . public. DHSingleLinkedList() { this.head = new Node (null); 1 // The head has no data,'or next value in the beginning this.size = 0; // When the LL is created, there are no other codes1 @Overridel public void.add(int index, E item) {1 // This method add an elements to the LLI // Depending on the index, it adds to the first.location using addFirst()1 // Or.adds after a node using addAfter()1 if(index size) {1 System.out.println("Invalid Index!"); 1 return; else if(index == 0) {1 //.We are adding at the first location addFirst (item); 1 else { //.We are going to add after some.nodel // We need the reference of that.node first Node node = getNode(index); // Get the reference of the prev node addAfter(node, item); | . private void.addFirst(E item) { // We create a node, with item as a data, and head.next.as the next // Then we update the head.next to point to this newly created.nodel // Finally, we increment the size 1/Node. . temp. := new.Node. (item, - head.next);1 //head. next = teno.: 1 head.next = new Node (item, head.next);1 size++; } private void addAfter(Node node, E item) {1 // Add the item after the reference: "node" node.next = new Node (item, node.next); size++; } private.Node. getNode(int index) {1 if(index size) { // Checking for a valid index| System.out.println("Invalid Index!"); return null; // This method loops over the LL, and returns the reference of Node at index| Node node = head; //This is a reference.copy of the headl for(int i = 0; i size) { // Checking for a valid index System.out.println("Invalid Index!"); 1 return null; } else if ( index : ==0) {1 return.removeFirst(); 1 } else { Node node = getNode(index); 1 return removeAfter(node); } >> private. E removeAfter(Node node) {1 1 Node temp = node.next; if (temp!= null) {! node. next = head.next; size --; return temp.data;1 } return null; 3 private. E removeFirst() {1 // Deletes the firstNode Node temp = head; 1 if (temp != null) {1 head = head.next;1 size --; return. temp.data; } return null; >> 9 >> >> //Implement public E set and public. E.get @Overridel public.Eget(int index) {1 if (index = size) {1 throw.new.IndexOutOfBoundsException(Integer.toString(index));1 Node node = getNode( index);1 ... return:node.data; } >> @Overridel public.E.set(int index, E newValue) {1 if (index = size) {1 throw.new.IndexOutOfBounds Exception(Integer.toString(index)); 1 } Node node = getNode(index); 1 Eresult = node.data; 1 .. node.data = newValue; return: result; @Overridel public int.size() { // This method returns the size of the LLT return this.size; se // Implement the.toString() to print the LL.datal - public String.toString() {1 String s = "[";1 >> >>> // Implement the toString() .to print the LL.datal public String.toString() {1 Strings. =:"[";1 Node. p = head; . // This reference will be used to iterate over the LLI if (p != null) {1 - while. (p.next!= null) {1 //. Iterate over the nodes one by one S+=p.next. data +.", ; // Adds the data to the String1 p = p.next; //Go to the next. Node 1 } >> >>> >> >> >> 1 // End of the LLI S+="]";1 return:s;1 }1 } package. DHLinkedList; 1 public class. DHLinkedListDriver: {I public static void main(String[] args) {1 // Create a .LL.objecti DHSingleLinkedList list = new DHSingleLinkedList ();1 System.out.println(list); 1 list.add(0, 10); System.out.println(list); 1 list.add(0,20); System.out.println(list);1 list.add(0,30); System.out.println(list); list.add(1,99); System.out.println(list);1 list.add(2,55); System.out.println(list); list.remove(0); System.out.println(list);1 list.remove(-1); System.out.println(list); & A ^^ ^ & ^ ^ ^ }1 } - Console X DHLinked ListDriver (1) [Java Applica [] [10 - >] [20 >10 - >] (30 >20 - >10 >10 - >] [30 >99 - >20 >10 >] [30 - >99 ->55 >20 >10 - >] [99 >55 - >20 - >10 >] Invalid Index! [99 >55 >20 - >10 - >]