Question
Hi,I'm having trouble with creating proper functions to fullfill the requirements listed below and l'm programming by using Python 3. An example script is needed
Hi,I'm having trouble with creating proper functions to fullfill the requirements listed below and l'm programming by using Python 3. An example script is needed to guide me. Thank you!
What is given:
Currently, all of the data on your students is stored in the file student_files.csv (*.csv means comma-separated-value file). Each line of data contains the students first and last name, their current grade for each of the subjects, as well as their objective grade for each of the subjects, as set after the discussion with the parents. The file also contains a warning grade for each subject.
The warning grade is higher than the objective grade and it serves as a buffer; if the students current grade dips below the warning grade, then its time to call the parents, before the students dip below their objective grade.
Objectives:
Implement simple file I/O commands to read data from a file, work with it, and write data onto a file.
Apply the concept of modular programming through the design of functions.
In the header of the file (the first row in the table), you will find the following fields:
Last Name,First Name,Math_cur,Math_obj,Math_war,Engl_cur,Engl_obj,Engl_war, Hist_cur,Hist_obj,Hist_war,Sci_cur,Sci_obj,Sci_war,Lang_cur,Lang_obj,Lang_war
Note: The field names occur on the same line in the file (the 1st line), but they did not fit on the page here, so the list is broken up. Also, you should note that none of these will be acceptable variable names to use since there are either spaces in the name, or they start with a capital letter. If you try and copy these names from this sheet and use them as variable names, they will not work.
Your task:
You need to generate a report that tells you two things about the students that are having difficulties achieving the grades they want:
1. Which courses do you need to be concerned about? A grade will be concerning only when the current grade is at or below
the warning level, but still above the semester objective (i.e. it is the score below which the student is in danger of falling below their desired grade).
2. Which courses where the student is in trouble? A grade will indicate trouble when the current grade is at or below
the objective for the semester. Note: These statuses are mutually exclusive, so courses that appear in the warning status should not appear in the trouble status and vice versa.
Here is an example of a students performance in Math and English:
This student would be considered to have a concerning status for Math, and a trouble status for English.
The report should contain a block for each student how has at least one concerning or trouble subject. The block should include the students full name on the first line, followed by a list of the concerning courses, followed by a list of trouble courses. For the concerning courses, the report should output the current grade and the warning grade for the subject in case, while for the trouble courses, the report should output the current grade and the objective grade.
To get the a consistent formatting, you will want to make use of the tab character \t, so an example of a line in your code would be:
print("\t Trouble Courses:")
There are some other things that you want to keep in mind for this. First of all, you do not want redundant data, so items that appear in the trouble section to not appear in the concerning section and vice versa. Second of all, while you do have access to the file, it can change on an almost daily basis (damn budget cuts!), so you want to write a program that will work for a file of any length.
Program Flow
1. Import the correct libraries 2. Open input file with student data 3. Create new output file grades_report.txt to write the reports into
(a) You can open files in the directory you are working in by using the open function: open("
The
a (append)
w (write)
r (read)
(b) WARNING: If the file grades_report.txt does not exist in the project directory, then the command open("grades_report.txt", "w") will create the file. If the file already exists in the project directory, then the command open("grades_report.txt", "w") will overwrite the existing file.
4. Ignore the THE HEADER line (the readline function is wonderful for this). You will need to remember however, the subjects evaluated and their order:
Math: columns 3-5
English: columns 6-8
History: columns 9-11
Science: columns 12-14
Language: columns 15-17
5. Iterate through each line of the input file: (a) For each line in the file, split the line into a list of 17 strings. Based on the values of the grades if each subject, decide whether or not a student has any grades in the concerning or trouble region.
Suggestion: since comparing each students grades is a task you will be doing
repeatedly, consider creating functions like has_trouble_course(
You may elect to pass the entire line as an argument to one of these functions, or just the 3 scores for one particular subject. Consider the advantages and disadvantages of each approach. Its your choice!
(b) If the student does have grades in the concerning or trouble region, you must append the students information to the report file, in the same format as the provided sample output file grades_report_sample.txt.
Suggestion: since writing to the report file is a task you will be doing repeatedly,
consider creating functions like add_trouble_to_report(
6. Once you are done iterating through the input file and have written the entire report to the grades_report.txt file, remember to CLOSE ANY FILES THAT ARE STILL OPEN (use the close function).
This is the example output:
Student name: Anita Abdo Concerning Courses: Current History grade: 81, Warning History grade: 83 Current Language grade: 77, Warning Language grade: 77 Trouble Courses: Current Science grade: 67, Objective Science grade: 91 Student name: Emmy Alfred Trouble Courses: Current Math grade: 66, Objective Math grade: 87 Current English grade: 76, Objective English grade: 91 Current History grade: 64, Objective History grade: 76 Current Science grade: 64, Objective Science grade: 71 Current Language grade: 66, Objective Language grade: 76 Student name: Nicholl Allyson Trouble Courses: Current Math grade: 70, Objective Math grade: 91 Current History grade: 64, Objective History grade: 92 Current Science grade: 65, Objective Science grade: 79
Here is how the grades of students in CSV look like:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
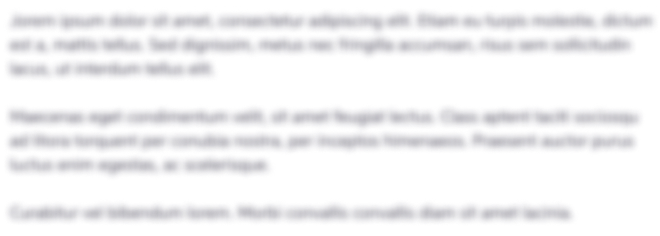
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started