Question
#HINT : I WANT TO IMPLEMENT THE DELETE LAST ( SHOW IN CODE ITS EMPTY ) */ public class CircularLinkedList { private class Node {
#HINT : I WANT TO IMPLEMENT THE DELETE LAST ( SHOW IN CODE ITS EMPTY ) */
public class CircularLinkedList {
private class Node {
int data; Node next;
public Node(int data, Node next) { this.data = data; this.next = next; }
public void setData(int data) { this.data = data; }
public void setNext(Node next) { this.next = next; }
public int getData() { return data; }
public Node getNext() { return next; } } Node head = null; Node tail = null; int size = 0;
public CircularLinkedList() { }
//Access Methods public int size() { return size; }
public boolean isEmpty() { return size == 0; }
public int first() { return head.getData(); }
public int last() { return tail.getData(); }
public void display() { if (isEmpty()) { System.out.println("Empty List.."); } Node current = head; int i = 1; do { System.out.println("Node " + (i++) + ": " + current.getData()); current = current.getNext(); } while (current != head); }
public void addLast(int value) { Node newNode = new Node(value, null);
if (isEmpty()) { head = newNode; newNode.next = head; tail = head; } else { newNode.next = head; tail.setNext(newNode); tail = newNode; } size++; }
public void find(int value) { if (isEmpty()) { System.out.println("Empty List.."); return; } int counter = 1; boolean flag = false; Node current = head; do { if (current.getData() == value) { flag = true; System.out.println("Found in Node# " + counter); break; } counter++; current = current.getNext(); } while (current != head); if (flag == false) { System.out.println("No record found"); }
} public void addFirst(int value) { Node newNode = new Node(value, null); if (size == 0) { head = newNode; newNode.next = head; tail = head; size++; return; } newNode.next = head; head = newNode; tail.next = newNode; size++; } void deleteLast() {
}
public static void main(String[] args) { CircularLinkedList list = new CircularLinkedList(); list.addFirst(10); list.addFirst(20); list.addFirst(30); list.addFirst(40); list.addFirst(50); list.addFirst(60); list.addFirst(70); list.addFirst(80); list.addFirst(90); list.addFirst(100); list.deleteLast(); list.display(); }
}
#HINT : I WANT TO IMPLEMENT THE DELETE LAST ( SHOW IN CODE ITS EMPTY )
Step by Step Solution
There are 3 Steps involved in it
Step: 1
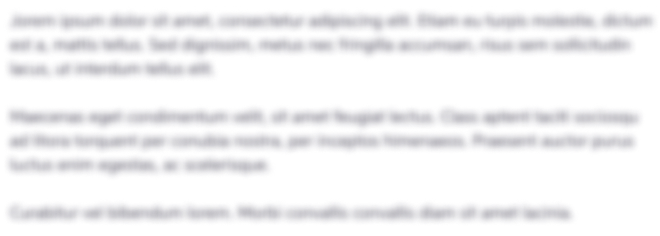
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started