Question
his assignment tests the concepts of: Pointers Dynamic memory Program Objective: Write and test a C++ program using dynamic memory allocation. You will not be
his assignment tests the concepts of:
Pointers
Dynamic memory
Program Objective:
Write and test a C++ program using dynamic memory allocation. You will not be using the bag class as we did in last lab. If at any time you need assistance, please get your professors attention. Please test your program between each step before moving on.
Write the code to your program
Save your program
Execute the program
Verify the output
Modify your program
DELIVERABLES:
Main program: lab02.cpp
Submitted assignments without the above file named correctly will render your assignment as uncompilable and will be detrimental to your assignment grade.
Instructions:
Reading in data:
Create a int pointer and set it to NULL or nullptr.
Create a size_t variable to maintain the current size of the dynamic array, initialize this variable to 0.
Create a for loop which iterates over all the command-line arguments
Do not sum the numbers in this loop (assuming you read ahead)
If first argument (argi == 1): Create an array of size 1 on the heap and set its value to the first command-line arguments numeric value (use atoi)
For all other arguments: Increase the size of the dynamic array by ONE. Grow the array without introducing any memory leaks
Create and use a function that resizes the array
place the numeric value of command-line argument to the empty location of the array
Processing of data:
In a separate loop located AFTER the one created in the last step.
Do not use argc in this loops condition
Calculate the mean of the numbers in the array
Display of results:
Display the mean of the numbers
Before the return 0 of your main function
Destroy the array and leave no memory on the heap
Additional requirements:
No STL containers may be used. Include only iostream and algorithm (if you wish to use copy).
No class creation and keep all code inside your one cpp file. Follow the best practices of C++ and utilize function prototypes separated from their implementation.
This is my code:
#include
using namespace std;
void resize(int * &a, size_t& oldSize, size_t newSize);
int main(int argc, char *argv[])
{
int *dp= nullptr;
*dp = new int
*dp = 1;
delete dp;
if (dp!= nullptr)
{delete dp;}
int argi;
if (argi==1)
size_t s = 0;
/*p= new int [0];
p [0]= 4;
p [1] = 3;
p [2] = 8;
s= 4;
int *np = new int [s + 1];
copy(p, p+s, np);
delete [] p;
p= np;*/
for ( size_t argi=1 ; argi < argc ; ++argi )
{
cout << "Arg_" << argi << ":" << argv[argi] << resize<< endl;
}
double value [1] = 89;
double sum =0;
for (int i =0; i < 1; ++i )
{
sum = sum + value[i];
}
cout << " The Mean of the Number: " << sum<< endl;
return 0;
}
void resize(int * &a, size_t& oldSize, size_t newSize)
{
if (newSize oldSize =newSize; int *p = new int [oldSize]; copy (a, a + oldSize, p); delete [] a; a=p; oldSize = newSize; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
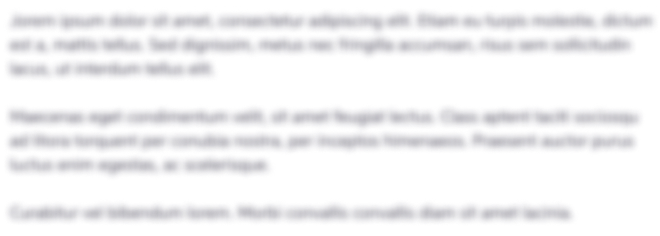
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started