Question
Holidays Using the date class below, Date.h, Date.cpp. main.cpp; create a list of at least 5 holidays. You may use either a vector or an
Holidays
Using the date class below, Date.h, Date.cpp. main.cpp; create a list of at least 5 holidays. You may use either a vector or an array.
Write a function that will receive the current year and return the date for Thanksgiving. Add that to the list.
Sort the list and print the name of the holiday and the day of the week for each holiday. (Hint: use an array for the days "Sunday", "Monday", etc.!)
Thanksgiving falls on the fourth Thursday of November (not the last Thursday as some months have five Thursdays). Additionally, Thanksgiving falls between the 22nd and the 28th of the month. There are some exceptions to these rules, specifically in 1940 and 1941 when Thanksgiving was held on the third Thursday.
Method: One way is to find the day of the week for Nov. 1 using the day of week method. Then figure out the date for the first Thursday. Then add 21 to get the date of the 4th Thursday. You may use any method that calculates the date.
Hint: A nice solution to this is to use an array with the day of the week of the first day of the week as the index. If Nov. 1 is a Sunday, then Thanksgiving is 26. If Nov. 1 is a Monday, then Thanksgiving is 25.
//Main.cpp
#include "Date.h" #include *********************************************************************** //Date.cpp #include "Date.h" #include //constructor to assign todays date to date char data[9]; //holder for the date _strdate(data); //gets the current date mm/dd/yy string date(data); //copy to a string for parsing if(date[0]=='0') month = stoi(date.substr(1,1)); else month = stoi(date.substr(0,2)); //gets characters 0 and 1 of date and converts to int if(date[3]=='0') day = stoi(date.substr(4,1)); else day = stoi(date.substr(3,2)); //day=stoi(date.substr(3,2)); //gets characters 3 and 4 of date and converts to int year=stoi(date.substr(6,2))+2000; //gets characters 6 and 7 of date and converts to int if(leapYear()) days2[2]=29; else days2[2]=28; for(int m=0;m<13;m++) days[m]=days2[m]; }//constructor for today bool validDate(int m, int d, int y) { bool valid=true; //assume it is valid until found to be invalid if(y<1000) valid=false; if(m<1 || m>12) valid=false; if(leapYear(y)) days2[2]=29; else days2[2]=28; if(d<1 || d>days2[m]) valid=false; return valid; }//validDate Date::Date(int m, int d, int y) { //constructor to assign values to month day and year if(validDate(m,d,y)) { month=m; day=d; year=y; } else { month=day=1; year=1970; //Unix time starting point } //not valid: set to default valid date for(int m=0;m<13;m++) days[m]=days2[m]; } //constructor with assigned values Date::Date(int julian) { //Fliegel-Van Flandern algorithm to convert Julian date to Gregorian number month, day, and year gregorian(julian,month,day,year); if(leapYear()) days2[2]=29; else days2[2]=28; for(int m=0;m<13;m++) days[m]=days2[m]; }//Date Julian Date::Date (string str) { //constructor for todays date as "mm/dd/year //Parse str by adding one char at a s time to the token until a "/" is encounter. //When "/" is encountered start the next token //int p=0; int count=0; int num[3]; string token[3]; int len=str.length(); for(int p=0; p if(str.substr(p,1)=="/") count++; else token[count]+=str.substr(p,1); }//parse str to create array of tokens bool error=false; for(int p=0;p<3;p++) { try { num[p]=stoi(token[p]); }//try to convert to int catch(invalid_argument&) { num[p]=-1; error=true; } //catch }//each of the 3 tokens if(!error && validDate(num[0],num[1],num[2])) { month=num[0]; day=num[1]; year=num[2]; } //no error else { month=day=1; year=1970; //Unix time starting point } //not valid: set to default valid date for(int m=0;m<13;m++) days[m]=days2[m]; }//constructor with string such as "10/31/2016" Date Date::operator=(const Date& otherDate) { //assigns another instance of the date class to this. month=otherDate.month; day=otherDate.day; year=otherDate.year; return *this; //allows date1=date=date3; }//overloaded operator = Date Date::operator+(int numDays) { //Adds the number of days to the Julian date. Date other(month,day,year); //make copy of the date int jd=other.julian(); //find the Julian date jd+=numDays; //add the number of days to the Julian date gregorian(jd,other.month,other.day,other.year); //Convert the Julian date back to Gregorian if(other.leapYear()) days2[2]=29; else days2[2]=28; for(int m=0;m<13;m++) other.days[m]=days2[m]; return other; } //operator + int Date::dayofYear() const { //returns the day of the year, ie 2/1/???? is the 32 day of the year int total=day; for(int m=1;m return total; }//dayofYear void gregorian(int julian, int &mth, int &d, int &y) { //Fliegel-Van Flandern algorithm to convert Julian date to Gregorian month, day, and year int p,q,r,s,t,u,v; p = julian + 68569; q = 4*p/146097; r = p - (146097*q + 3)/4; s = 4000*(r+1)/1461001; t = r - 1461*s/4 + 31; u = 80*t/2447; v = u/11; y = 100*(q-49)+s+v; mth = u + 2 - 12*v; d = t - 2447*u/80; } //Gregorian int Date::julian() const { int jd= day-32075+1461*(year+4800+(month-14)/12)/4+ 367*(month-2-(month-14)/12*12) /12-3*((year+4900+(month-14)/12)/100)/4; return jd; } bool Date::leapYear() const { bool leap=false; if(year%4==0) leap=true; if(year%100==0 && year%400!=0) leap=false; return leap; }//leapYear bool leapYear(int yr) { bool leap=false; if(yr%4==0) leap=true; if(yr%100==0 && yr%400!=0) leap=false; return leap; }//leapYear int Date::weekday() const { //returns 0 for Sunday, 1 for Monday, etc. static int t[] = { 0, 3, 2, 5, 0, 3, 5, 1, 4, 6, 2, 4 }; int y =year; y-= month < 3; return ( y + y/4 - y/100 + y/400 + t[month-1] + day) % 7; }//weekday int Date::getMonth() const { //private variables cannot be accessed directly but require "getter" functions return month; }//getMonth int Date::getDay() const { return day; }// int Date::getYear() const { return year; }//getYear string Date::toString() const { stringstream oss; //a stream to append the values oss< return oss.str(); } bool Date::operator==(const Date& otherDate) { return (month==otherDate.month && day==otherDate.day && year==otherDate.year); }//operator == bool Date::operator<(const Date& otherDate) { //A date is less than another date if is earlier bool result=false; //assume false until proven true if(year else if(year==otherDate.year && month else if(year==otherDate.year && month==otherDate.month && day return result; }//operator bool Date::operator>(const Date& otherDate) { //Convert both dates to Julian and compare the Julian dates int jd1=julian(); int jd2=otherDate.julian(); return jd1>jd2; }//operator ostream& operator << (ostream &output, const Date &d) { output << d.toString(); return output; } // operator << istream& operator >> (istream &input, Date &d) { string s; input >> s; Date other(s); //create a new Date d=other; //assign the new Date to d return input; } // operator >> ************************************************ //Date.h #ifndef Date_H #define Date_H #include using namespace std; class Date { private: int month, day, year; int days[13]; public: //constructors Date(); //constructor for todays date Date(int m, int d, int y); //constructor to assign date Date(string str); //constructor for todays date as "mm/dd/year Date(int gregorian); //constructor to convert a Gregorian date to Date //methods int getMonth() const; //returns the private variable month int getDay() const; //returns the private variable day int getYear() const; //returns the private variable year string toString() const; //returns the string mm/dd/yyyy bool leapYear() const; //determines if the year is a leap year int dayofYear() const; //returns the day of the year: ie 2/1/???? is the 32 day of year int julian() const; int weekday() const; //returns 0 for Sunday, 1 for Monday, etc. //overloaded operators bool operator==(const Date& otherDate); //2 dates are equal if month, day and year are equal bool operator<(const Date& otherDate); //a date is < another date if it is earlier bool operator>(const Date& otherDate); //a date is > another date if it is later Date operator=(const Date& otherDate); //let's you copy one date to another. Date operator+(int); //Assign new values to the date after adding the number of days friend ostream& operator << (ostream &output, const Date &d); friend istream& operator >> (istream &input, Date &d); }; bool validDate(int m, int d, int y); //test other date bool leapYear(int y); //let's you test any year, not just the year for the instance int julian(int m, int d, int y); //convert any date to Julian void gregorian(int jd, int &mth, int &d, int &y); static int days2[]={0,31,28,31,30,31,30,31,31,30,31,30,31}; #endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
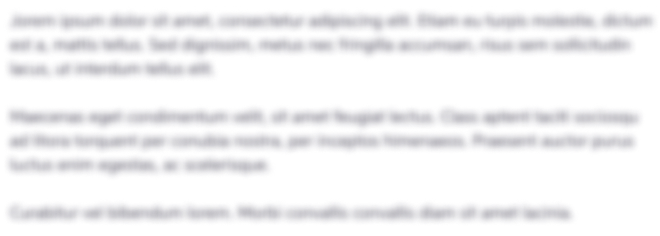
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started