Question
home / study / engineering / computer science / questions and answers / my assignment asked the following. i am unable ... Question: My assignment
home / study / engineering / computer science / questions and answers / my assignment asked the following. i am unable ...
Question: My assignment asked the following. I am unable to ...
Bookmark
My assignment asked the following. I am unable to get the UI working and having trouble with the rest of the bullet points below. Answer in code only is fine, once I see it, it usually makes a lot more sense. Any help would be appreciated!!
Create a user interface for the Library application that allows the user (i.e., the Librarian) to enter Book objects.
Create a business layer manager, BookMgr, with the following stubbed-out method: public Book storeBook(Book book). The add button will cause the user-supplied information to be packaged into a domain Book object. It will also:
Pass the domain Book object to the BookMgr.storeBook function.
Display the state of the Book using its overridden toString() method; e.g System.out.println(book.toString());
CODE
package libraryapp;
public class Book { private String[] author; private int ISBN; private String title;
// Constructor public Book(String authors[], int ISBN, String title) { this.author = new String[authors.length]; for (int i = 0; i < author.length; i++) { author[i] = authors[i]; } this.ISBN = ISBN; this.title = title; }
public Book() { throw new UnsupportedOperationException("Not supported yet."); }
public String[] getAuthor() { return this.author; }
public int getIsbn() { return this.ISBN; }
public String getTitle() { return this.title; }
public void setAuthor(String[] author) { this.author = author; }
public void setISBN(int ISBN) { this.ISBN = ISBN; }
public void setTitle(String title) { this.title = title; }
public boolean validate() { if (author == null || author.equals("")) return false; if (title == null || title.equals("")) return false; if (ISBN == 0) return false; return true; } }
FORM
package libraryapp;
public class BookForm extends javax.swing.JFrame {
/** * Creates new form BookForm */ public BookForm() { initComponents(); }
/** * This method is called from within the constructor to initialize the form. * WARNING: Do NOT modify this code. The content of this method is always * regenerated by the Form Editor. */ @SuppressWarnings("unchecked") // private void initComponents() {
jLabel1 = new javax.swing.JLabel(); jTextField1 = new javax.swing.JTextField(); jLabel2 = new javax.swing.JLabel(); jLabel3 = new javax.swing.JLabel(); jTextField2 = new javax.swing.JTextField(); jButton2 = new javax.swing.JButton();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
jLabel1.setFont(new java.awt.Font("Arial", 1, 24)); // NOI18N jLabel1.setText("Library Application");
jTextField1.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jTextField1ActionPerformed(evt); } });
jLabel2.setText("Enter Book Title");
jLabel3.setText("Entered Book");
jButton2.setText("OK");
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane()); getContentPane().setLayout(layout); layout.setHorizontalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addContainerGap() .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false) .addComponent(jLabel1) .addComponent(jTextField1) .addComponent(jLabel2) .addComponent(jLabel3, javax.swing.GroupLayout.PREFERRED_SIZE, 85, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(jTextField2)) .addComponent(jButton2)) .addContainerGap(170, Short.MAX_VALUE)) ); layout.setVerticalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addContainerGap() .addComponent(jLabel1) .addGap(33, 33, 33) .addComponent(jLabel2) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED) .addComponent(jTextField1, javax.swing.GroupLayout.PREFERRED_SIZE, 27, javax.swing.GroupLayout.PREFERRED_SIZE) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED) .addComponent(jButton2) .addGap(14, 14, 14) .addComponent(jLabel3) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED) .addComponent(jTextField2, javax.swing.GroupLayout.PREFERRED_SIZE, 29, javax.swing.GroupLayout.PREFERRED_SIZE) .addContainerGap(78, Short.MAX_VALUE)) );
pack(); }//
private void jTextField1ActionPerformed(java.awt.event.ActionEvent evt) { // TODO add your handling code here: }
/** * @param args the command line arguments */ public static void main(String args[]) { /* Set the Nimbus look and feel */ // try { for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) { if ("Nimbus".equals(info.getName())) { javax.swing.UIManager.setLookAndFeel(info.getClassName()); break; } } } catch (ClassNotFoundException ex) { java.util.logging.Logger.getLogger(BookForm.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } catch (InstantiationException ex) { java.util.logging.Logger.getLogger(BookForm.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } catch (IllegalAccessException ex) { java.util.logging.Logger.getLogger(BookForm.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } catch (javax.swing.UnsupportedLookAndFeelException ex) { java.util.logging.Logger.getLogger(BookForm.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } //
/* Create and display the form */ java.awt.EventQueue.invokeLater(new Runnable() { public void run() { new BookForm().setVisible(true); } }); }
// Variables declaration - do not modify private javax.swing.JButton jButton2; private javax.swing.JLabel jLabel1; private javax.swing.JLabel jLabel2; private javax.swing.JLabel jLabel3; private javax.swing.JTextField jTextField1; private javax.swing.JTextField jTextField2; // End of variables declaration }
TEST
package libraryapp;
import org.junit.Test; import static org.junit.Assert.*;
public class BookTest { public BookTest() { } /** * Test of getAuthor method, of class Book. */ @Test public void testGetAuthor() { System.out.println("getAuthor"); Book instance = new Book(new String[]{"Mouse"},123, "Mickey Mouse"); String[] expResult = new String[]{"Mouse"}; String[] result = instance.getAuthor(); assertEquals(expResult[0], result[0]); } /** * Test of getIsbn method, of class Book. */ @Test public void testGetIsbn() { System.out.println("getIsbn"); Book instance = new Book(new String[]{"Mickey","Mouse"},123, "Mickey Mouse"); int expResult = 123; int result = instance.getIsbn(); assertEquals(expResult, result); } /** * Test of getTitle method, of class Book. */ @Test public void testGetTitle() { System.out.println("getTitle"); Book instance = new Book(new String[]{"Mickey","Mouse"},123, "Mickey Mouse"); String expResult = "Mickey Mouse"; String result = instance.getTitle(); assertEquals(expResult, result); } /** * Test of setAuthor method, of class Book. */ @Test public void testSetAuthor() { System.out.println("setAuthor"); String author = "Mouse"; Book instance = new Book(new String[]{},123, "Micky Mouse"); instance.setAuthor(new String[]{author});
assertEquals(author, instance.getAuthor()[0]); } /** * Test of setISBN method, of class Book. */ @Test public void testSetISBN() { System.out.println("setISBN"); int ISBN = 0; Book instance = new Book(new String[]{"Mickey"}, 123, "Mickey Mouse"); instance.setISBN(ISBN); assertEquals(ISBN, instance.getIsbn()); } /** * Test of setTitle method, of class Book. */ @Test public void testSetTitle() { System.out.println("setTitle"); String title = "Mickey"; Book instance = new Book(new String[]{"Mickey"}, 123, "Mickey Mouse"); instance.setTitle(title);
assertEquals(title, instance.getTitle()); } /** * Test of validate method, of class Book. */ @Test public void testValidate() { System.out.println("validate"); Book instance = new Book(new String[]{"Mickey"}, 0, "Mickey Mouse"); boolean expResult = false; boolean result = instance.validate(); assertEquals(expResult, result); }
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
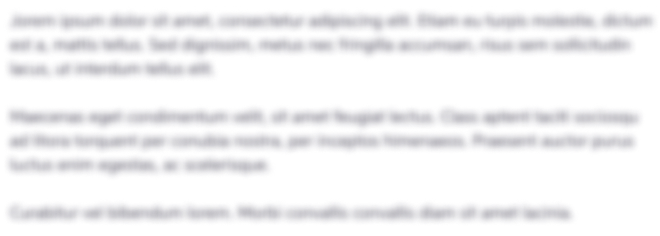
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started