Question
Homework 4-1 Textbook Section 9.9 Take the Passenger class from the previous Homework 3-1 and add the following to it: Passenger height : double Passenger(String,
Homework 4-1 Textbook Section 9.9
Take the Passenger class from the previous Homework 3-1 and add the following to it:
Passenger
-
height : double
-
Passenger(String, int, double, double, char, int)//name, birthYear, weight, height, gender, numCarryOn
-
getHeight() : double
-
setHeight(double) : void //if input value is negative set height to -1
-
calculateBMI() : double //using pounds and inches BMI = ( weight * 703) / (height2)
-
printDetails() : void // prints Passenger attributes in the following format:
// "Name: %20s | Year of Birth: %4d | Weight: %10.2f | Height: %10.2f | Gender: %c | NumCarryOn: %2d "
-
toString() : String @Override //see Note1 for format
-
equals(Object) : boolean @Override //see Note2 for equality checks
NOTE1:
Format the data for the toString() method as
"Name: %20s | Year of Birth: %4d | Weight: %10.2f | Height: %10.2f | Gender: %c | NumCarryOn: %2d ""
NOTE2:
For the equals(Object) method, two Passenger objects are considered equal if
both have the same values for birthYear, gender, name
and if their weight, height values are within .5 of the others weight, height values
For more detailed instructions view javadoc for Passenger and Airplane classes HERE
Homework 4-2 Textbook Section 9.10
Create a class to represent a Person object that inherits from (extends) the Passenger class.
Use the description provided below in UML.
Person
-
numOffspring : int
-
Person() //default values for numOffspring should be 0
-
Person(int)
-
Person(String, int, double, double, char, int, int) //pass values to parents overloaded constructor
//and assign valid values to numOffspring or 0 if invalid
-
setNumOffspring(int) : void //if the value is invalid set numOffspring to 0
-
getNumOffspring() : int
-
printDetails() : void @Override //see Note1 below:
-
toString() : String @Override //see Note1 below:
-
equals(Object) : boolean @Override //see Note2:
NOTE1:
For both the printDetails() and toString() methods include the data from the Passenger class, as well as Person attributes. Format the Person data as Person: Number of Offspring: %4d
Hint: Use super.printDetails() and super.toString()
NOTE2:
For the equals(Object) method, two Person objects are considered equal if the
parent classs equals method returns true, if their numOffspring are equal
For more detailed instructions view javadoc for Person, Passenger, and Airplane classes HERE
Homework 4-3 Textbook Section 9.11
Create a class to represent a Infant object that inherits from (extends) the Person class. Use the description provided below in UML. Create a nested class named InfantToy within the Infant class
Infant
InfantToy //an inner class (non-static)
-
infantToyName: String
-
infantToyRating: int
+ InfantToy(String, int)
+ toString() : String
Infant (continued)
-
toys : BabyToy [ ]
-
numBabyToys : int
-
Infant() //an Infant with zero InfantToys but array capacity of 10
-
Infant(String, int, double, double, char, int) //pass values to parent classs overloaded constructor
//Infant cannot have offSpring therefore pass a zero to the parent constructor
-
addInfantToy(String,int) //uses the InfantToy constructor, do not resize array
-
getInfantToyAsString(int) : String
-
getNumInfantToys() : int
-
getInfantToyName(int) : String
-
getInfantToyRating(int) : int
-
getHighestInfantToyRating(): int //should return the highest rating, or 0 if the array is empty
-
printDetails() : void @Override //see NOTE below for formatting
-
toString() : String @Override //see NOTE below for formatting
NOTE1:
For both the printDetails() and toString() methods include the data from the Passenger class, as well as Person attributes.
Format the Infant data as Infant: Number of Toys: %4d | Infant Toys: followed by the
formatted InfantToy String for each toy on its own line as InfantToy: Toy Name: %20s | Rating %4d
NOTE2:
In the Infant class do not override the equals method inherited from the Person class
NOTE3:
In the Infant class do not resize the toys array. The max capacity should remain 10
Hint1: Use super.printDetails() and super.toString()
Hint2: InfantToy is immutable (i.e. no setter methods, its values cannot be changed)
Hint3: InfantToy belongs to Infant only, which is why it is a nested class
Hint4: toString() inside the InfantToy should be formatted as InfantToy: Toy Name: %20s | Rating %4d
Hint5: toString() inside the Infant should iterate through toys and append the String returned from each InfantToy
This work must be completed in your textbook ZYBooks -- CMP-168: Programming Methods II
No other forms of submission will be accepted.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
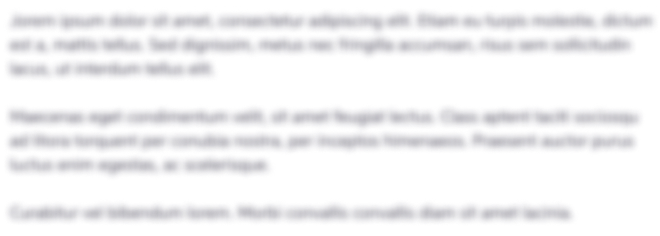
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started