Question
/* Homework Lab 37 In this program the user will enter the following information for historical baseball players (up to the maximum number of players
/* Homework Lab 37
In this program the user will enter the following information for historical baseball players (up to the maximum number of players indicated by MAX_NUM_OF_PLAYERS):
The player's name The number of hits they got in their career The number of at bats during their career
The information that the user enters must be stored in parallel arrays (one array for each statistic listed above). When the user says they are done entering players -OR- they have entered the maximum number of players, then a chart must be produced on the screen showing each player's name and their batting average. Batting average is calculated as follows (and will be calculated with a function you prototype, implement, and call):
batting average = # of hits
Note: Don't forget that hits and at bats will be stored in arrays
In addition to displaying the batting average chart, the program also needs to determine which player has the highest batting average and display the name of the batting champion.
To complete this assignment, follow the TODO steps listed below. */
#include
//------------------------------------------------------------------------------
// TODO - Step 1a // Declare function prototype // Name: BattingAverage // Parameters: an int for the number of hits // an int for the number of at bats // Returns: a float for the calculated batting average (hits
int main() { const int MAX_NUM_OF_PLAYERS = 10;
/* TODO - Step 2 Declare 4 arrays, with the data types listed below - each holding as many values as determined by the constant declared above:
- array of string, to hold the names of the players - array of int, to hold the hits for each player - array of int, to hold the number of at bats for each player - array of float, to hold the calculated batting average for each player */
// Parallel array declarations
// These variables will hold array index values int currentPlayerIndex = 0; // used when user enters data, to retain current array index int battingChampIndex = 0; // array index that points to the player with the highest average
// Loop control variable, holds 'y' if player wants to add another player char again;
/* TODO - Step 3 Complete the DO...WHILE loop that has been started here. You will need to scroll down (after STEP 11) to where the following line is commented out:
//} while (expression);
Uncomment the line and change "expression" so the loop repeats if: - the user indicated they want to add another player, AND - fewer than MAX_PLAYERS have been added */
do { /* TODO - Step 4 Output prompt to tell user which player they are entering stats for */
/* TODO - Step 5 - Prompt the user for the player's name - Read the user's answer and store to the correct array in the correct location */
/* TODO - Step 6 - Prompt the user for the number of hits for this player - Read the user's answer and store to the correct array in the correct location */
/* TODO - Step 7 Write a WHILE loop to validate the user's entry. If the user's entry for hits was 0 or less: - Display an error message (example in 2nd player Sample Output below) - Read the user's answer again and store to the correct array in the same location */
/* TODO - Step 8 - Prompt the user for the number of at bats for this player - Read the user's answer and store to the correct array in the correct location */
/* TODO - Step 9 Write a WHILE loop to validate the user's entry. If the user's entry for at bats less than the number of hits: - Display an error message (example in 3rd player Sample Output below) - Read the user's answer again and store to the correct array in the same location */
/* TODO - Step 10 Increment the index that is being used for the parallel arrays, readying it for the next time around loop */
/* TODO - Step 11 If the parallel array index is still less than the max number of players allowed (as identified by the MAX_NUM_OF_PLAYERS constant), then - display a prompt to ask the user if they want to enter another player - read the user's answer from the keyboard (into the 'again' variable declared above) - convert the user's answer to lower case and store it back to the same 'again' variable
*/
cout << endl;
// ---------------------------------------------- // This is where you come for TODO - STEP 2 // ----------------------------------------------
//} while (expression);
// END OF THE DO...WHILE LOOP // Uncomment the line and change "expression" so the loop repeats if: // -the user indicated they want to add another player, AND // - fewer than MAX_NUM_OF_PLAYERS have been added
// Display the header for the chart cout << endl; cout << "PLAYER AVG" << endl; cout << "=================" << endl; cout << left << fixed << setprecision(3);
/* TODO - Step 12 Write a for loop that will loop based on the number of players entered above. Think about what variable contains the number of players entered. Also, you will need to declare your own loop control variable for the for loop */
//for (initialize; test; increment) { /* TODO - Step 13 Calculate the player's batting average by calling the BattingAverage function
Note: don't forget that hits and at bats are stored in arrays - also, the batting average should ALSO be stored to an array in this step once it is calculated here! */
/* TODO - Step 14 Output the player's name and average as a single line to the console, make sure columns are aligned correctly. */
/* TODO - Step 15 If the batting average for the current player is higher than the batting average for the player pointed to by battingChampIndex, then - save the current for loop index as the new battingChampIndex
Note: we are working with the INDEX here, not the average... */
} // End of the for loop
/* TODO - Step 16 Display the line identifying the batting champ (as determined in the loop above) */
} // End of main()
// TODO - Step 1b // Define BattingAverage function // Purpose: Function to calculate and return the a baseball batting average, // which is hits
/* Sample Output
Enter Player #1 stats: Name: Aaron Total Hits: 3771 Total At Bats: 12364 Enter another player (y/n): y
Enter Player #2 stats: Name: Cobb Total Hits: 0 Reenter Hits: 4189 Total At Bats: 11434 Enter another player (y/n): y
Enter Player #3 stats: Name: Gehrig Total Hits: 2721 Total At Bats: 801 Reenter At Bats: 8001 Enter another player (y/n): y
Enter Player #4 stats: Name: Ruth Total Hits: 2873 Total At Bats: 8398 Enter another player (y/n): n
PLAYER AVG ================= Aaron 0.305 Cobb 0.366 Gehrig 0.340 Ruth 0.342
Batting champion: Cobb, 0.366 career average! Press any key to continue . . .
*/
Step by Step Solution
There are 3 Steps involved in it
Step: 1
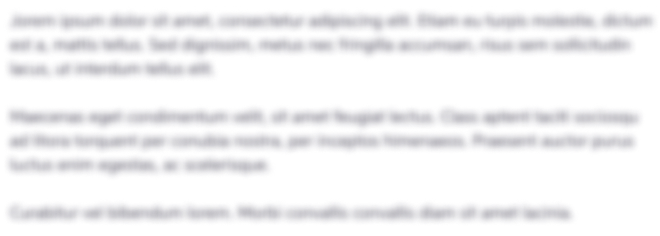
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started