Question
How can i do this ? public class Heap extends AbstractQueue { private List theData = new ArrayList (); public Heap () {} /** An
How can i do this ?
public class Heap
private List
public Heap () {}
/** An optional reference to a Comparator object. */
Comparator comparator = null;
/** Creates a heap-based priority queue with that orders its
elements according to the specified comparator.
@param comp The comparator used to order this priority queue
*/
public Heap (Comparator comp) {
comparator = comp;
}
public int size () { return theData.size(); }
/** Compare the items with index i and index j in theData using
either a Comparator object's compare method or their natural
ordering using method compareTo.
@param i index of first item in theData
@param j index of second item in theData
@return Negative int if first less than second,
0 if first equals second,
positive int if first > second
@throws ClassCastException if items are not Comparable
*/
private int compare (int i, int j) {
if (comparator == null)
return ((Comparable
else
return comparator.compare(theData.get(i), theData.get(j));
}
/** Swap the items with index i and index j in theData.
@param i index of first item in theData
@param j index of second item in theData
*/
private void swap (int i, int j) {
E temp = theData.get(i);
theData.set(i, theData.get(j));
theData.set(j, temp);
}
/** Insert an item into the priority queue.
pre: theData is in heap order.
post: The item is in the priority queue and
theData is in heap order.
@param item The item to be inserted
@throws NullPointerException if the item to be inserted is null.
*/
public boolean offer (E item) {
if (item == null)
throw new NullPointerException();
theData.add(item);
int child = size() - 1;
int parent = (child - 1) / 2;
while (child > 0 && compare(child, parent)
swap(parent, child);
child = parent;
parent = (child - 1) / 2;
}
return true;
}
/** Remove an item from the priority queue
pre: The ArrayList theData is in heap order.
post: Removed smallest item, theData is in heap order.
@return The item with the smallest priority value or null if empty.
*/
public E poll() {
// IMPLEMENT
// Return null if queue is empty.
if(size()==0)
return null;
// Save the top of the heap.
E result = theData.get(0);
// If only one item then remove it and you are done.
if(size() == 1) {
theData.remove(0);
return result;
}
/* Remove the last item from the ArrayList and place it into
the first position. */
E last = theData.get(size()-1);
theData.set(0, last);
theData.remove(size()-1);
int parent = 0;
int left = 2*(parent)+1;
int right = 2*(parent)+2;
while(right
if (right 0){
swap(parent,right);
parent=right;
}
else{
swap (parent,left);
parent = left;
}
left = 2*(parent)+1;
right = 2*(parent)+2;
}
return result;
/* Use swaps to move that item into the correct position. */
/* See the lab notes for a hint on this loop. */
}
public E peek() {
if (size()==0){
return null;
}
return theData.get(0);
}
public Iterator
return theData.iterator();
}
/** Remove a specified item from the priority queue.
If it appears more than once, only one is removed.
@param o The item to be removed.
@returns true if item remove, false if it was not there.
*/
public boolean remove (Object o) {
// Get the index of the object to be removed.
int index = theData.indexOf(o);
// If it is not there, done.
if (index == -1)
return false;
// If it is the last item, just remove it.
if (index == theData.size() - 1) {
theData.remove(index);
return true;
}
// Copy the item at size-1 to index and then remove the item at size-1.
// If the item at index is better than its parent, swap it upward
// as in offer. Otherwise, swap it downward as in poll().
return true;
}
public String toString () {
return toString(0, 0);
}
private String toString (int root, int indent) {
if (root >= size())
return "";
String ret = toString(2 * root + 2, indent + 2);
for (int i = 0; i
ret = ret + " ";
ret = ret + theData.get(root) + " ";
ret = ret + toString(2 * root + 1, indent + 2);
return ret;
}
public static void main (String[] args) {
int[] data = { 3, 1, 4, 1, 5, 9, 2, 6 };
Heap
for (int i = 0; i
queue.offer(data[i]);
System.out.println(queue);
System.out.println("----------------");
System.out.println();
}
for (int i = 0; i
queue.poll();
System.out.println(queue);
System.out.println("----------------");
System.out.println();
}
}
@Override
public Iterator
// TODO Auto-generated method stub
return null;
}
}
Now switch to using your Heap using your Comparator. It should run the same. Switch back to PriorityQueue for the next partStep by Step Solution
There are 3 Steps involved in it
Step: 1
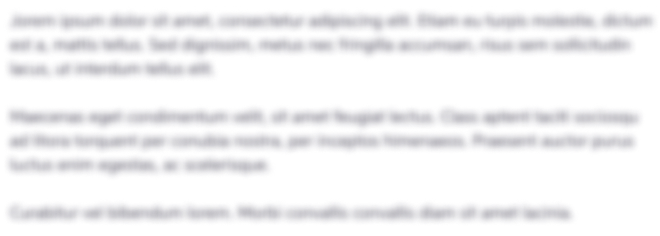
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started