Question
How can I make the date in number format look like 01/21/2018 instead of the output below which comes out as 21/01/118 import java.util.*; public
How can I make the date in number format look like 01/21/2018 instead of the output below which comes out as 21/01/118
import java.util.*;
public class date {
/*
* this method increases given date by one day.
* */
@SuppressWarnings("deprecation")
public void increaseDate(Date d){
d.setDate(d.getDate()+1);
System.out.println("Date after increment by one day");
System.out.println(d);
}
/*
* this method prints date in number format
*
* */
@SuppressWarnings("deprecation")
public void printDateInNumber(Date d)
{
System.out.println("Date in Number format");
System.out.println(d.getDate()+"-"+d.getMonth()+1+"-"+d.getYear());
}
/*
* this method prints date in string format
*
* */
public void printDateInString(Date d)
{
System.out.println("Date in String format");
System.out.println(d.toString());
}
/*main method will be the steps for the program to ask for the information that the other method will implement.
*
*
*/
@SuppressWarnings("deprecation")
public static void main(String[] args) {
date d=new date();
int day,month,year;
Scanner scan=new Scanner(System.in);//for input form user
System.out.print("enter Date in DD Format:-");
day= scan.nextInt();
System.out.print("enter Month in MM format:-");
month= scan.nextInt();
System.out.print("enter year in YYYY format:-");
year= scan.nextInt();
scan.close(); //close the input channel
Date thisDay=new Date(); //create a current date instance
//set date to given inputs from user
thisDay.setDate(day);
thisDay.setMonth(month-1);
thisDay.setYear(year-1900);
//display date in number format
d.printDateInNumber(thisDay);
//display date in string format
d.printDateInString(thisDay);
//increase the date by one day
d.increaseDate(thisDay);
}
}
**********************************************
OUTPUT
enter Date in DD Format:-21 enter Month in MM format:-01 enter year in YYYY format:-2018 Date in Number format 21-01-118 Date in String format Sun Jan 21 12:16:26 HST 2018 Date after increment by one day Mon Jan 22 12:16:26 HST 2018
Step by Step Solution
There are 3 Steps involved in it
Step: 1
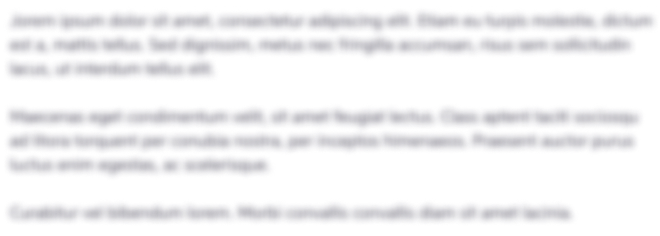
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started