Question
How can I modify my code (see below) to meet the requirements that follows?: In this assignment we will change how memory is allocated, with
How can I modify my code (see below) to meet the requirements that follows?:
In this assignment we will change how memory is allocated, with the goal to call realloc() less often. When the user adds the first item to the list, we will now allocate memory for 3 items instead of one. This means that we dont need to allocate memory or call realloc() again until we add a 4th item. When the 4th item is added, the program should again allocate memory for 3 new items so we dont need to call realloc() until a 7th item is added, and so forth. In order to make this work, you need to add a new member to the ShoppingList struct called allocated. This new member variable contains how many grocery items that are currently allocated, while length is still the current length of the list. allocated should initially be 0 (because no memory has been allocated). After the first item has been added, length should be 1, but allocated should be 3. Throughout the program allocated should always be at least as large as length. (2p) So, this means that realloc()should only be called when length exceeds the current amount of allocated elements, and should extend the memory allocated by 3 items instead of just 1. (3p) Finally, we want the program to show information about how many items are in the list as well as how many spaces are allocated when the user prints the list (see the example run). (1p) Example run on the next page.
Example run (user input in bold, comments in italics): Welcome to the shopping list manager! ===================================== 1. Add an item 2. Print an item 3. Remove an item 4. Change an item 5. Save list 6. Load list 7. Exit What do you want to do? 1 Enter name: Potatoes Enter amount: 1.5 Enter unit: kg Potatoes was added to the shopping list. Welcome to the shopping list manager! ... What do you want to do? 2 Your list contains 1 items, 3 spaces are allocated: this is new! 1. Potatoes 1.5 kg ... After filling the list with 3 more items... What do you want to do? 2 Your list contains 4 items, 6 spaces are allocated: 1. Potatoes 1.5 kg 2. Candy 200 g 3. Salmon 300 g 4. Milk 1 kg
My code:
#define _CRT_SECURE_NO_WARNINGS #include"ShoppingList.h" #include
void addItem(struct ShoppingList* list) { printf("=============================== "); printf("Your list contains %d items ", list->length);
struct GroceryItem* allocated = 0;
list->length++; struct GroceryList* newList = (struct GroceryItem*)realloc(list->itemList, sizeof(struct GroceryItem) * list->length);
if (newList == NULL) { printf("Error"); } else { list->itemList = newList;
while (getchar() != ' '); printf("Enter name: "); gets(list->itemList[list->length - 1].productName);//minus 1 fr att hamna p rtt plats
int item_index = -1; for (int i = 0; i < list->length - 1; i++) { if (strcmp(list->itemList[i].productName, list->itemList[list->length - 1].productName) == 0) { item_index = i; break; } }
// If the item is already in the list, update the amount if (item_index != -1) { list->itemList[item_index].amount += list->itemList[list->length - 1].amount; list->length--; printf("This item is already in the list! Enter new amount: "); scanf_s("%f", &list->itemList[item_index].amount);
while (list->itemList[item_index].amount <= 0.0) //if the amount is negative, enter new/valid amount { printf("Invalid number. "); printf("Enter amount: "); scanf_s("%f", &list->itemList[item_index].amount); } } // Otherwise, add the new item to the list else { printf("Enter amount: "); scanf_s("%f", &list->itemList[list->length - 1].amount);
while (list->itemList[list->length - 1].amount <= 0.0) //om amount r negativ eller =0, be om ny amount { printf("Invalid number. "); printf("Enter amount: "); scanf_s("%f", &list->itemList[list->length].amount); }
while (getchar() != ' '); printf("Enter unit: "); gets(list->itemList[list->length - 1].unit); } }
printf("The list now contains %d items ", list->length); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
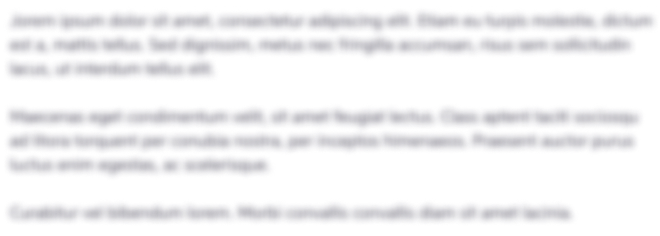
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started