Question
How can make this class in two separate classs, the menu inside this class should stand as a separate calss like Menu.java import java.io.File; import
How can make this class in two separate classs, the menu inside this class should stand as a separate calss like "Menu.java"
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.PrintWriter;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Date;
import java.util.Scanner;
public class Driver {
static ArrayList
static ArrayList
static File dogsFile;
static File coursesFile;
static Scanner scanner;
public static void main(String[] args) {
dogsFile = new File("c://temp/dogs.txt");
coursesFile = new File("c://temp/courses.txt");
dogs = new ArrayList
courses = new ArrayList
scanner = new Scanner(System.in);
try {
loadData();
showMenu();
} catch (FileNotFoundException e) {
System.out.println("File not found");
}
}
/**
* method to load data from datafiles
*/
static void loadData() throws FileNotFoundException {
Scanner fileScanner = new Scanner(coursesFile);
while (fileScanner.hasNextLine()) {
String line = fileScanner.nextLine();
/**
* Splitting the line by white spaces
*/
String[] fields = line.split(" ");
String name = fields[0].trim();
double maxTime = Double.parseDouble(fields[1].trim());
/**
* creating a new Courses instance
*/
Courses c = new Courses(name, maxTime);
/**
* Adding to the arraylist
*/
courses.add(c);
}
fileScanner = new Scanner(dogsFile);
while (fileScanner.hasNextLine()) {
String line = fileScanner.nextLine();
/**
* Splitting the line by white spaces
*/
String[] fields = line.split(" ");
String id = fields[0].trim();
String name = fields[1].trim();
double runningTime = Double.parseDouble(fields[2].trim());
double penalty = Double.parseDouble(fields[3].trim());
char code = fields[4].trim().charAt(0);
/**
* creating a new Dogs instance
*/
Dogs d = new Dogs(id, name, runningTime, penalty, code);
/**
* Adding to the arraylist
*/
dogs.add(d);
}
}
/**
* method to display the menu and get user choice repeatedly until user
* quits
*/
static void showMenu() {
System.out.println("1. Print report");
System.out.println("2. Add a Dog");
System.out.println("3. Exit");
System.out.println("Enter a choice: ");
try {
int choice = Integer.parseInt(scanner.nextLine());
switch (choice) {
case 1:
printReport();
break;
case 2:
addDog();
break;
case 3:
System.out.println("Good bye!");
System.exit(0);
default:
System.out.println("Invalid choice");
break;
}
} catch (Exception e) {
System.out.println("Invalid choice, try again");
showMenu();
}
showMenu();
}
/**
* method to print report this will prompt the user to select a course, and
* then will call printReport(coursecode, maxtime) method, which will
* calculate and print the statistics
*/
static void printReport() {
System.out.println("Choose the course:");
System.out
.println("Enter G for Gamblers, J for Jumpers, or T for Titling");
String choice = scanner.nextLine();
if (choice.equalsIgnoreCase("G")) {
for (Courses c : courses) {
if (c.getName().equalsIgnoreCase("Gamblers")) {
printReport('G', c.getMaximumTime());
}
}
} else if (choice.equalsIgnoreCase("J")) {
for (Courses c : courses) {
if (c.getName().equalsIgnoreCase("Jumpers")) {
printReport('J', c.getMaximumTime());
}
}
} else if (choice.equalsIgnoreCase("T")) {
for (Courses c : courses) {
if (c.getName().equalsIgnoreCase("Titling")) {
printReport('T', c.getMaximumTime());
}
}
} else {
System.out.println("Invalid choice");
}
}
/**
* this method will be called by the printReport() method by supplying
* course code and maxtime. this will calculate and print the report of the
* specified course
*/
static void printReport(char courseCode, double maxTime) {
String courseName = "";
if (courseCode == 'G') {
courseName = "Gamblers";
} else if (courseCode == 'J') {
courseName = "Jumpers";
} else if (courseCode == 'T') {
courseName = "Titling";
}
System.out.println("Report\t" + courseName + "\tCourse Time: "
+ maxTime);
Date date = new Date();
System.out.println("Date: "
+ new SimpleDateFormat("MMMMM dd,yyyy").format(date));
System.out.printf("%10s %15s %15s %10s %10s %15s", "ID", "Name",
"Running Time", "Penalty", "Total", "Over/Under Time");
/**
* two Dogs objects to represent winning dog and the dog with most
* penalties
*/
Dogs winningDog = null;
Dogs dogWithMostPenalty = null;
for (Dogs d : dogs) {
char c = d.getCourseCode();
/**
* Considering only those dogs who participated in this course
*/
if (Character.toUpperCase(c) == courseCode) {
/**
* Calculating the total time
*/
double totalTime = d.getRunningTime() + d.getPenalty();
if (winningDog == null) {
winningDog = d;
} else {
/**
* checking if the total time is less than that of the
* currently assumed winning dog's if yes, assign current
* dog as the winning dog
*/
if (totalTime < (winningDog.getRunningTime() + winningDog
.getPenalty())) {
winningDog = d;
}
}
if (dogWithMostPenalty == null && d.getPenalty() > 0) {
dogWithMostPenalty = d;
} else {
if (d.getPenalty() > dogWithMostPenalty.getPenalty()) {
dogWithMostPenalty = d;
}
}
double over_underTime = totalTime - maxTime;
String overUnderTimeString = "";
if (over_underTime > 0) {
/**
* appending the + sign to the overtime string
*/
overUnderTimeString = "+"
+ String.format("%.2f", over_underTime);
} else {
overUnderTimeString = String.format("%.2f", over_underTime);
}
/**
* Displaying the stats
*/
System.out.printf(" %10s %15s %15.2f %10.2f %10.2f %15s",
d.getId(), d.getName(), d.getRunningTime(),
d.getPenalty(), totalTime, overUnderTimeString);
}
}
System.out.println();
if (winningDog == null) {
/**
* No dogs
*/
System.out.println("No data");
} else {
System.out.println("Winning Dog: " + winningDog.getName()
+ ", Time: "
+ (winningDog.getRunningTime() + winningDog.getPenalty()));
}
if (dogWithMostPenalty != null) {
System.out.println("Dog with most penalty: "
+ dogWithMostPenalty.getName() + ", Penalty: "
+ dogWithMostPenalty.getPenalty());
}
}
/**
* method to add a dog to the arraylist and to the datafile
*/
static void addDog() {
System.out
.println("Enter ID: (1 digit followed by a letter, followed by 3 digits)");
String id = scanner.nextLine();
if (id.length() != 5) {
System.out.println("Invalid ID");
return;
} else {
/**
* Checking if id is in proper format
*/
if (!(Character.isDigit(id.charAt(0))
&& Character.isLetter(id.charAt(1))
&& Character.isDigit(id.charAt(2))
&& Character.isDigit(id.charAt(3))
&& Character.isDigit(id.charAt(4)))) {
System.out.println("Invalid ID");
return;
}
}
System.out.println("Enter dog name: ");
String name = scanner.nextLine();
try {
System.out.println("Enter running time");
double runTime = Double.parseDouble(scanner.nextLine());
if (runTime <= 0) {
System.out.println("Invalid run time");
return;
}
System.out.println("Enter penalty: ");
double penalty = Double.parseDouble(scanner.nextLine());
if (penalty < 0) {
System.out.println("Invalid penalty");
return;
}
System.out.println("Enter course code: ");
String code = scanner.nextLine();
if (code.equalsIgnoreCase("G") || code.equalsIgnoreCase("J")
|| code.equalsIgnoreCase("T")) {
Dogs d = new Dogs(id, name, runTime, penalty, code.charAt(0));
dogs.add(d);
/**
* Defining a printwriter object to append data to the file
*/
PrintWriter writer = new PrintWriter(new FileOutputStream(dogsFile,true));
writer.append(" " + id + " " + name + " " + runTime + " "
+ penalty + " " + code);
writer.close();
System.out.println("Dog added");
}
} catch (NumberFormatException e) {
System.out.println("Invalid input");
} catch (Exception e) {
System.out.println("Some error occured");
return;
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
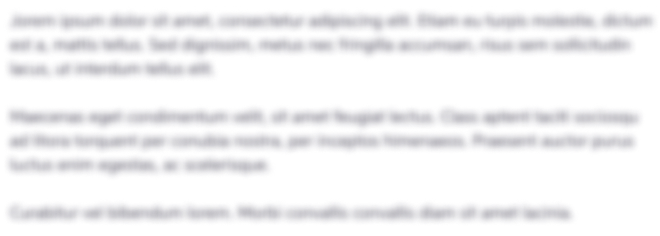
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started