Question
How do I change the position of an object in a Java Singly Linked List from one position to another. I have tried every way
How do I change the position of an object in a Java Singly Linked List from one position to another. I have tried every way and nothing works so far. Here is my problem and is here is my code. Everything compiles, but the changePosition method.
Implement the "Change position of song" menu option. Prompt the user for the current position of the song and the desired new position. Valid new positions are 1 - n (the number of nodes). If the user enters a new position that is less than 1, move the node to the position 1 (the head). If the user enters a new position greater than n, move the node to position n (the tail). 6 cases will be tested:
Moving the head node (1 pt)
Moving the tail node (1 pt)
Moving a node to the head (1 pt)
Moving a node to the tail (1 pt)
Moving a node up the list (1 pt)
Moving a node down the list (1 pt)
public class SongEntry {
// Private fields private String uniqueID; private String songName; private String artistName; private int songLength; private SongEntry headNode; // Reference to the head node private SongEntry currNode; // Reference to the current node private SongEntry nextNode; // Reference to the next node // Default constructor public SongEntry() { uniqueID = "none"; songName = "none"; artistName = "none"; songLength = 0; headNode = null; currNode = null; nextNode = null; } // Parameterized constructor public SongEntry(String idInit, String songNameInit, String artistInit, int songLgthInit) { uniqueID = idInit; songName = songNameInit; artistName = artistInit; songLength = songLgthInit; } // Mutators public void setUniqueID(String setUniqueID) { this.uniqueID = setUniqueID; } public void setSongName(String setSongName) { this.songName = setSongName; } public void setArtistName(String setArtistName) { this.artistName = setArtistName; } public void setSongLength(int setSongLgth) { this.songLength = setSongLgth; }
// Function to set link to head node public void setHeadNode(SongEntry firstLoc) { this.headNode = firstLoc; } // Function to set link to current node public void setCurrNode(SongEntry currLoc) { this.currNode = currLoc; } // Function to set link to next node public void setNext(SongEntry nextLoc) { this.nextNode = nextLoc; } // Accessor for songID public String getID() { return uniqueID; } // Accessor for song name public String getSongName() { return songName; } // Accessor for artist name public String getArtistName() { return artistName; } // Accessor for song length public int getSongLength() { return songLength; } public SongEntry getHead() { return this.headNode; } public SongEntry getCurrNode() { return this.currNode; } public SongEntry getNext() { return this.nextNode; } /* Insert node after this node. * Before: this --> next * After: this --> node --> next */ public void insertAfter(SongEntry currNode) { SongEntry tmpNext; tmpNext = this.nextNode; this.nextNode = currNode; currNode.nextNode = tmpNext; return; } public void addSong(SongEntry songList) { // Create node objects SongEntry headObj; // Create SongEntry objects SongEntry lastObj; headObj = new SongEntry(); lastObj = headObj; int i = 0; // Loop index lastObj.insertAfter(songList); } public SongEntry isEmpty() { return headNode = null; } // Given a Playlist head, find out length of linked list public int length(SongEntry head) { if (head == null) { return 0; } // Create a count variable to hold length int count = 0; // loop each element and increment the count till list ends SongEntry currNode = head; while(currNode != null) { count++; // move to next node currNode = currNode.getNext(); } return count; } public int findPosition(SongEntry head, String songID) { int position = -1; int count = 0; boolean find = false; SongEntry currNode = head; int length = length(head); //get the length of linked list while (find != true) { count++; if (count == length - 1) { find = true; } currNode = currNode.getNext(); if (currNode.getID().equals(songID)) { position = count; find = true; } } return position; } public String deleteSong(SongEntry head, String songID) { //delete a song String songRemoved = ""; int position = findPosition(head, songID); int length = length(head); //get the length of linked list if(position > length || position < 1) { //make sure item is in list if not comes back as -1 System.out.println("Song not found"); } else { SongEntry prevNode = head; int count = 1; while(count < position ) { prevNode = prevNode.nextNode; count++; } SongEntry curNode = prevNode.nextNode; songRemoved = curNode.getSongName(); prevNode.nextNode = curNode.nextNode; curNode.nextNode = null; System.out.println("\"" + songRemoved + "\"" + " removed."); System.out.println(""); } return songRemoved; } // end deleteSong
public SongEntry getEntryAtIndex(SongEntry head, int currPos) { if (currPos == 0) return null;
SongEntry entry = head; for (int i = 0; i < currPos; ++i) { entry = entry.getNext(); } return entry; } public SongEntry changePosition(SongEntry head, int currPos, int newPos) { SongEntry linkedList = head; int length = length(head); SongEntry currNode = getEntryAtIndex(linkedList, currPos); String songName = currNode.getSongName(); //SongEntry prevNode = getEntryAtIndex(linkedList, newPos); //String prevSongName = prevNode.getSongName(); //System.out.println("Current song is " + songName + " and previous song name is " + prevSongName); //Empty List - Returned newly created node or null if (head==null){ return head; } // This did nothing int count = 0; while(count < newPos) { count++; } return head; } // end change position method public String removeSong(SongEntry head, String songID) { //delete a song String songRemoved = ""; int position = findPosition(head, songID); int length = length(head); //get the length of linked list if(position > length || position < 1) { //make sure item is in list if not comes back as -1 //System.out.println("Song not found"); } SongEntry prevNode = head; int count = 1; while(count < position ) { prevNode = prevNode.nextNode; count++; } SongEntry curNode = prevNode.nextNode; songRemoved = curNode.getSongName(); prevNode.nextNode = curNode.nextNode; curNode.nextNode = null; return songRemoved; //System.out.println("Song position is " + count); } // end removeSong method public void findArtist(SongEntry head, String artistName) { int count = 0; // loop each element and increment the count till list ends SongEntry currNode = head; while(currNode != null) { if(currNode.getArtistName().equals(artistName)) { currNode.printSongEntry(currNode, count); } currNode = currNode.nextNode; count++; } } public void totalTime(SongEntry head) { int time = 0; SongEntry currNode = head; while(currNode != null) { currNode = currNode.getNext(); if (currNode != null) { time += currNode.getSongLength(); } } System.out.println("Total time: " + time + " seconds"); System.out.println(""); } public void printSongEntry(SongEntry currObj, int count) { System.out.println(count + "."); System.out.println("Unique ID: " + this.uniqueID); System.out.println("Song Name: " + this.songName); System.out.println("Artist Name: " + this.artistName); System.out.println("Song Length (in seconds): " + this.songLength); System.out.println(""); return; } // Given a ListNode, print all elements it hold // This is for testing purposes public void display(SongEntry head) { if(head == null) { return; } SongEntry currNode = head; // loop each element till end of the list // last node points to null while(currNode != null) { System.out.print(currNode.songName + " --> "); // print current element's data currNode = currNode.nextNode; } System.out.print(currNode); // here current will be null }
} // end SongEntry class
import java.util.Scanner;
public class Playlist { public static String title; static Scanner scnr = new Scanner(System.in);
public static void main(String[] args) { Scanner scnr=new Scanner(System.in); SongEntry song; System.out.println("Enter playlist's title: "); title = scnr.nextLine(); song = new SongEntry(); printMenu(song, scnr); scnr.close(); } // end main // Method to display a menu public static void menu() { // Print menu System.out.println(title + " PLAYLIST MENU"); System.out.println("a - Add song"); System.out.println("d - Remove song"); System.out.println("c - Change position of song"); System.out.println("s - Output songs by specific artist"); System.out.println("t - Output total time of playlist (in seconds)"); System.out.println("o - Output full playlist"); System.out.println("q - Quit"); System.out.println(""); } // Method to display a menu and perform appropriate action public static void printMenu(SongEntry songList, Scanner scnr) { // Local variables String choice; String uniqueID, songName, artistName; int songLength; boolean selectOption = true; // Create node objects SongEntry headObj; // Create SongEntry objects SongEntry currObj; SongEntry lastObj; headObj = new SongEntry(); lastObj = headObj; while(true) { // display menu menu(); do { System.out.println("Choose an option:"); choice = scnr.next().trim(); switch(choice.toLowerCase().charAt(0)) { case 'q': selectOption = false; return; case 'a': scnr.nextLine(); // remove newline character from keyboard System.out.println("ADD SONG"); System.out.println("Enter song's unique ID:"); uniqueID = scnr.nextLine(); System.out.println("Enter song's name:"); songName = scnr.nextLine(); System.out.println("Enter artist's name:"); artistName = scnr.nextLine(); System.out.println("Enter song's length (in seconds):"); songLength = Integer.parseInt(scnr.nextLine()); System.out.println(""); // assign class object and song values to accessors SongEntry song = new SongEntry(); song.setUniqueID(uniqueID); song.setSongName(songName); song.setArtistName(artistName); song.setSongLength(songLength); song.setNext(song); // Add item to linked list song.addSong(song); lastObj.insertAfter(song); lastObj = song; // display menu menu(); break; case 'd': scnr.nextLine(); // remove newline character from keyboard System.out.println("REMOVE SONG"); System.out.println("Enter song's unique ID:"); uniqueID = scnr.nextLine(); currObj = headObj; //starts loop at head node lastObj.deleteSong(currObj, uniqueID); // display menu menu(); break; case 'c': scnr.nextLine(); //remove newline character from keyboard System.out.println("CHANGE POSITION OF SONG"); System.out.println("Enter song's current position:"); int position = scnr.nextInt(); System.out.println("Enter new position for song:"); int newPosition = scnr.nextInt(); lastObj.changePosition(headObj, position, newPosition); // display menu menu(); break; case 's': scnr.nextLine(); // eliminate newline char System.out.println("OUTPUT SONGS BY SPECIFIC ARTIST"); System.out.println("Enter artist's name:"); String artistID = scnr.nextLine(); lastObj.findArtist(headObj, artistID); // display menu menu(); break; case 't': scnr.nextLine(); // remove newline character from keyboard System.out.println("OUTPUT TOTAL TIME OF PLAYLIST (IN SECONDS)"); lastObj.totalTime(headObj); // display menu menu(); break; case 'o': int count = 0; currObj = headObj; currObj = currObj.getNext(); //skip past headObj position System.out.println(title + " - OUTPUT FULL PLAYLIST"); while (currObj != null) { count++; currObj.printSongEntry(currObj, count); currObj = currObj.getNext(); } if (count == 0) { System.out.println("Playlist is empty "); } // display menu menu(); break; } // end switch } while (!choice.equals('q')); } // end while }
} // end Playlist class
Step by Step Solution
There are 3 Steps involved in it
Step: 1
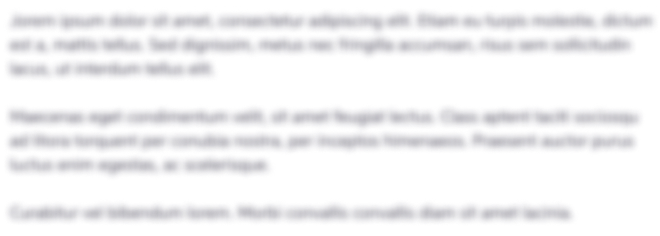
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started