Question
How Do I fix this code? /* * To change this license header, choose License Headers in Project Properties. * To change this template file,
How Do I fix this code?
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package finalproject;
/**
*
* @author Athena
*/
public class FinalProject {
private String nameOfIngredient;
private float numberCups;
private int numberCaloriesPerCup;
private double totalCalories;
/**
* @return the nameOfIngredient
*/
public String getNameOfIngredient() {
return nameOfIngredient;
}
/**
* @param nameOfIngredient the nameOfIngredient to set
*/
public void setNameOfIngredient(String nameOfIngredient) {
this.nameOfIngredient = nameOfIngredient;
}
/**
* @return the numberCups
*/
public float getNumberCups() {
return numberCups;
}
/**
* @param numberCups the numberCups to set
*/
public void setNumberCups(float numberCups){
this.numberCups = numberCups;
}
/**
* @param numberCaloriesPerCup the numberCaloriesPerCup to set
*/
public void setNumberCaloriesPerCup(int numberCaloriesPerCup) {
this.numberCaloriesPerCup = numberCaloriesPerCup;
}
/**
* @return the totalCalories
* I basically set up all the individual codes with the total calories
* to make sure that they will go together accordingly.
*/
public double getTotalCalories() {
return totalCalories;
}
/**
* @param totalCalories the totalCalories to set
*/
public void setTotalCalories(double totalCalories) {
this.totalCalories = totalCalories;
}
public FinalProject() {
this.nameOfIngredient = "";
this.numberCups = 0;
this.numberCaloriesPerCup = 0;
this.totalCalories = 0.0;
}
public FinalProject(String nameOfIngredient, float numberCups,
int numberCaloriesPerCup, double totalCalories) {
this.nameOfIngredient = nameOfIngredient;
this.numberCups = numberCups;
this.numberCaloriesPerCup = numberCaloriesPerCup;
this.totalCalories = totalCalories;
}
public static void main(String[] args) {
int numberCups = -1;
final int MAX_CUPS = 100;
Scanner scnr = new Scanner(System.in);
System.out.printIn("Please enter the number of cups (between 1 and 100): ");
if (scnr.hasNextInt()) {
numberCups = scnr.nextInt();
if (numberCups >= 1 && numberCups <= MAX_CUPS) {
System.out.printIn(numberCups + " is a valid number of cups!");
} else {
System.out.printIn(numberCups + " is a not valid number of cups!");
System.out.printIn("Please enter another number of cups between 1 and 100: ");
numberCups = scnr.nextInt();
if (numberCups >= 1 && numberCups <= MAX_CUPS) {
System.out.printIn(numberCups + " is a valid number of cups!");
} else if (numberCups < 1) {
System.out.printIn(numberCups + " is less than 1. Sorry you are out of tries.");
} else {
System.out.printIn(numberCups + " is larger than 100. Sorry you are out of tries.");
} else {
System.out.printIn("Error: That is not a number. Try again.");
/**
* I made the code so that there will be a clean setup of amount of cups per recipe.
*/
}
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
String recipeName = "";
ArrayList
String newIngredient = "";
boolean addMoreIngredients = true;
System.out.println("Please enter the recipe name: ");
recipeName = scnr.nextLine();
do {
System.out.println("Would you like to enter an ingredient?: ");
String reply = scnr.next().toLowerCase();
if (reply.equals("y")) {
System.out.println("What is the ingredient?");
newIngredient = scnr.next();
ingredientList.add(newIngredient);
} else if (!reply.equals("n")) {
System.out.println("Please enter y or n");
} else {
addMoreIngredients = false;
}
} while (addMoreIngredients) ;
for (int i = 0; i < ingredientList.size(); i++ ) {
System.out.println(ingredientList.get(i));
}
/**
*Getting a system of the whether or not the ingredients go in the recipe.
*/
private String recipeName;
private int servings;
private ArrayList
private double totalRecipeCalories;
/**
* @return the recipeName
*/
public String getRecipeName() {
return recipeName;
}
/**
* @param recipeName the recipeName to set
*/
public void setRecipeName(String recipeName) {
this.recipeName = recipeName;
}
/**
* @return the servings
*/
public int getServings() {
return servings;
}
/**
* @param servings the servings to set
*/
public void setServings(int servings) {
this.servings = servings;
}
/**
* getting the servings setup.
*/
/**
* @return the recipeIngredients
*/
public ArrayList
return recipeIngredients;
}
/**
* @param recipeIngredients the recipeIngredients to set
*/
public void setRecipeIngredients(ArrayList
this.recipeIngredients = recipeIngredients;
}
/**
* @return the totalRecipeCalories
*/
public double getTotalRecipeCalories() {
return totalRecipeCalories;
}
/**
*getting the calorie count up.
*/
/**
* @param totalRecipeCalories the totalRecipeCalories to set
*/
public void setTotalRecipeCalories(double totalRecipeCalories) {
this.totalRecipeCalories = totalRecipeCalories;
}
public SteppingStone5_Recipe() {
this.recipeName = "";
this.servings = 0;
this.recipeIngredients = new ArrayList<>();
this.totalRecipeCalories = 0;
}
public SteppingStone5_Recipe(String recipeName, int servings, ArrayList
this.recipeName = recipeName;
this.servings = servings;
this.recipeIngredients = recipeIngredients;
this.totalRecipeCalories = totalRecipeCalories;
}
public void printRecipe() {
double singleServingCalories = totalRecipeCalories / servings;
System.out.println("Recipe: " + getRecipeName());
System.out.println("Yield: " + getServings() + " servings");
System.out.println("Ingredients:");
/*
for (Ingredient currentIngredient: recipeIngredients)
System.out.println(currentIngredient.getIngredientName());
*/
for (int i = 0; i < recipeIngredients.size(); i++) {
Ingredient currentIngredient = recipeIngredients.get(i);
String currentIngredientName = currentIngredient.getIngredientName();
System.out.println(currentIngredientName);
}
System.out.println("Total Calories per serving: " + singleServingCalories);
}
public SteppingStone5_Recipe createNewRecipe() { //Comment out for Stepping Stone 6
//public SteppingStone5_Recipe createNewRecipe() { //uncomment for Stepping Stone 6
double totalRecipeCalories = 0;
ArrayList
boolean addMoreIngredients = true;
Scanner scnr = new Scanner(System.in);
System.out.println("Please enter the recipe name: ");
String recipeName = scnr.nextLine();
System.out.println("How many servings: ");
int servings = scnr.nextInt();
do {
System.out.println("Please enter the ingredient name or type 'e' if you are done: ");
String ingredientName = scnr.next();
if (ingredientName.toLowerCase().equals("e")) {
addMoreIngredients = false;
}
else {
Ingredient tempIngredient = new Ingredient().enterNewIngredient(ingredientName);
recipeIngredients.add(tempIngredient);
}
} while (addMoreIngredients);
for (int i = 0; i < recipeIngredients.size(); i++) {
Ingredient currentIngredient = recipeIngredients.get(i);
float ingredientAmount = currentIngredient.getIngredientAmount();
int ingredientCalories = currentIngredient.getIngredientCalories();
double ingredientTotalCalories = ingredientAmount * ingredientCalories;
totalRecipeCalories += ingredientTotalCalories;
}
//Declaring instance variables private ArrayList of the type of SteppingStone5_Recipe
private ArrayList
//Accessors and Mutators for listOfRecipes
/**
* @return the listOfRecipes
*/
public ArrayList
return listOfRecipes;
}
/**
* @param listOfRecipes the listOfRecipes to set
*/
public void setListOfRecipes(ArrayList
this.listOfRecipes = listOfRecipes;
}
/**
* @param listOfRecipes
*/
//Contructors for SteppingStone6_RecipeBox()
public SteppingStone6_RecipeBox(ArrayList
this.listOfRecipes = listOfRecipes;
}
public SteppingStone6_RecipeBox() {
this.listOfRecipes = new ArrayList<>();
}
//custom method to printAllRecipeDetails
void printAllRecipeDetails(String selectedRecipe){
for (SteppingStone6_RecipeBox recipe : listOfRecipes){
if(recipe.().equalsIgnoreCase(selectedRecipe)) {
recipe.printRecipe();
return;
}
}
//custom method to AddRecipe
public void addRecipe(SteppingStone5_Recipe tempRecipe){
listOfRecipes.add(tempRecipe);
}
/**
* getting the recipe code set up with the list of recipes
*/
/**
* @param args the command line arguments
*/
public static void main(String[]args) {
//Create a Recipe Box
SteppingStone6_RecipeBox myRecipeBox = new SteppingStone6_RecipeBox();
Scanner menuScnr = new Scanner(System.in);
//printing menu for user to select the available options
System.out.printIn("Menu/n" + "1. Add Recipe/n" + "2. Print All Recipe Details/n" + "3. Print All Recipe Names/n" + "/nPlease select a menu item');"
while (menuScnr.hasNextInt() || menuScnr.hasNextLine()) {
int input = menuScnr.nextInt();
/**
* The code below has two variations:
* 1. Code used with the SteppingStone6_RecipeBox_tester.
* 2. Code used with the public static main() method
*
* One of the sections should be commented out depending on the use.
*/
/**
* This could should remain uncommented when using the
* SteppingStone6_RecipeBox_tester.
*
* Comment out this section when using the
* public static main() method
*/
/** if (input == 1) {
recipe1();
} else if (input == 2) {
System.out.println("Which recipe? ");
String selectedRecipeName = menuScnr.next();
printAllRecipeDetails(selectedRecipeName);
} else if (input == 3) {
for (int j = 0; j < listOfRecipes.size(); j++) {
System.out.println((j + 1) + ": " + listOfRecipes.get(j).getRecipeName());
}
} else {
System.out.println(" Menu " + "1. Add Recipe " + "2. Print Recipe " + "3. Adjust Recipe Servings " + " Please select a menu item:");
}
**/
/**
* This could should be uncommented when using the
* public static main() method
*
* Comment out this section when using the
* SteppingStone6_RecipeBox_tester.
*
**/
//Using if-else to select three custom methods.
if (input == 1) {
myRecipeBox.recipe1();
} else if (input == 2) {
System.out.println("Which recipe? ");
String selectedRecipeName = menuScnr.next();
myRecipeBox.printAllRecipeDetails(selectedRecipeName);
} else if (input == 3) {
for (int j = 0; j < myRecipeBox.listOfRecipes.size(); j++) {
System.out.println((j + 1) + ": " + myRecipeBox.listOfRecipes.get(j).getRecipeName());
}
} else {
System.out.println(" Menu " + "1. Add Recipe " + "2. Print Recipe " + "3. Adjust Recipe Servings " + " Please select a menu item:");
}
System.out.println("Menu " + "1. Add Recipe " + "2. Print All Recipe Details " + "3. Print All Recipe Names " + " Please select a menu item:");
}
}
public void recipe1() {
double totalRecipeCalories = 0.0;
ArrayList
boolean addMoreIngredients = true;
Scanner scnr = new Scanner(System.in);
System.out.println("Please enter the recipe name: "); //prompt user to enter recipe name.
String recipeName = scnr.nextLine(); //checks if valid input was entered.
System.out.println("Please enter the number of servings: "); //prompt user to enter number of servings for recipe.
int servings = scnr.nextInt(); //checks if valid input was entered.
do {
//prompt user to enter ingredients or type end to finish loop.
System.out.println("Please enter the ingredient name or type end if you are finished entering ingredients: ");
String ingredientName = scnr.next().toLowerCase();
//if user type end loop will end and print the recipe with ingredient, serving and calories.
if (ingredientName.toLowerCase().equals("end")) {
addMoreIngredients = false;
} else {
//if user enter the ingredient loop will start ask for ingredient details.
recipeIngredients.add(ingredientName); //creating array list for recipe ingredients.
//prompt user to enter ingredient amount
System.out.println("Please enter an ingredient amount: ");
float ingredientAmount = scnr.nextFloat();
//prompt user to enter measuring unit of ingredient
System.out.println("Please enter the measurement unit for an ingredient: ");
String unitMeasurement = scnr.next();
//promt user to enter ingredient calories
System.out.println("Please enter ingredient calories: ");
int ingredientCalories = scnr.nextInt();
//Expression to calculate total calories
totalRecipeCalories = (ingredientCalories * ingredientAmount);
//prints the ingredient name with required amount, measuring unit and total calories.
System.out.println(ingredientName + " uses " + ingredientAmount + " " + unitMeasurement + " and has " + totalRecipeCalories + " calories.");
} //end else loop.
} while (addMoreIngredients);
// Now create a recipe object and add it to the list
SteppingStone5_Recipe recipe = new SteppingStone5_Recipe(recipeName, servings, recipeIngredients, totalRecipeCalories);
addRecipe(recipe);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
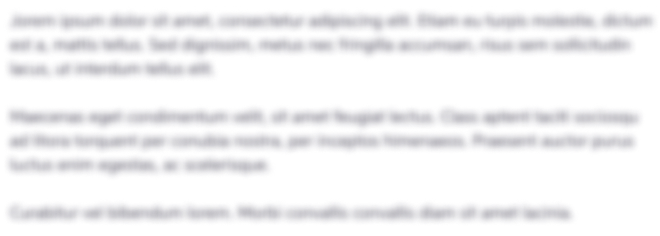
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started