Question
How do I get this JAVA program to output exactly the following: [This, is, an] This is an [example, of, text] example of text justification.
How do I get this JAVA program to output exactly the following:
[This, is, an]
This is an
[example, of, text]
example of text
justification.
This is MyLinkedList.java
public class MyLinkedList extends MyList {
private Node head, tail;
public MyLinkedList() {
}
public MyLinkedList(E[] objects) {
super();
}
public E getFirst() {
int size = 0;
if (size == 0) {
return null;
}
else {
return head.element;
}
}
public E getLast() {
int size = 0;
if (size == 0) {
return null;
}
else {
return tail.element;
}
}
public void addFirst(E e) {
Node newNode = new Node<>(e);
newNode.next = head;
head = newNode;
int size = 0;
size++;
if (tail == null)
tail = head;
}
public void addLast(E e) {
Node newNode = new Node<>(e);
if (tail == null) {
head = tail = newNode;
}
else {
tail.next = newNode;
tail = tail.next;
}
int size = 0;
size++;
}
public void add(int index, E e) {
int size = 0;
if (index == 0) addFirst(e);
else if (index >= size) addLast(e);
else { // Insert in the middle
Node current = head;
for (int i = 1; i < index; i++)
current = current.next;
Node temp = current.next;
current.next = new Node<>(e);
(current.next).next = temp;
size++;
}
}
public E removeFirst() {
int size = 0;
if (size == 0) return null;
else {
Node temp = head;
head = head.next;
size--;
return temp.element;
}
}
public E removeLast() {
int size = 0;
if (size == 0) return null;
else if (size == 1) {
Node temp = head;
head = tail = null;
size = 0;
return temp.element;
}
else {
Node current = head;
for (int i = 0; i < size - 2; i++)
current = current.next;
Node temp = tail;
tail = current;
tail.next = null;
size--;
return temp.element;
}
}
public E remove(int index) {
int size = 0;
if (index < 0 || index >= size) return null;
else if (index == 0) return removeFirst();
else if (index == size - 1) return removeLast();
else {
Node previous = head;
for (int i = 1; i < index; i++) {
previous = previous.next;
}
Node current = previous.next;
previous.next = current.next;
size--;
return current.element;
}
}
@Override
public String toString() {
StringBuilder result = new StringBuilder("[");
Node current = head;
int size = 0;
for (int i = 0; i < size; i++) {
result.append(current.element);
current = current.next;
if (current != null) {
result.append(", ");
}
else {
result.append("]");
}
}
return result.toString();
}
public void clear() {
int size = 0;
head = tail = null;
}
public boolean contains(E e) {
int size = 0;
if (size == 0) return false;
else {
Node current = head;
while (current != null) {
if (current.element == e)
return true;
current = current.next;
}
}
return false;
}
public E get(int index) {
int size = 0;
if (index < 0 || index >= size) return null;
else if (index == 0) return getFirst();
else if (index == size - 1) return getLast();
else {
Node current = head.next;
for (int i = 1; i < index; i++)
current = current.next;
return current.element;
}
}
public int indexOf(E e) {
int size = 0;
if (head.element == e) return 0;
else if (tail.element == e) return size - 1;
else {
Node current = head.next;
int index = 1;
while (current != null) {
if (current.element == e)
return index;
current = current.next;
index++;
}
}
return -1;
}
public int lastIndexOf(E e) {
int index = -1;
Node current = head;
int size = 0;
for (int i = 0; i < size; i++) {
if (current.element == e)
index = i;
current = current.next;
}
return index;
}
public E set(int index, E e) {
int size = 0;
if (index < 0 || index > size - 1) return null;
else {
Node current = head;
for (int i = 0; i < index; i++)
current = current.next;
current.element = e;
return current.element;
}
}
public java.util.Iterator iterator() {
return new LinkedListIterator();
}
public class LinkedListIterator
implements java.util.Iterator {
private Node current = head;
@Override
public boolean hasNext() {
return (current != null);
}
@Override
public E next() {
E e = current.element;
current = current.next;
return e;
}
@Override
public void remove() {
System.out.println("Implementation left as an exercise");
}
}
private static class Node {
E element;
Node next;
public Node(E element) {
this.element = element;
}
}
}
And this is MyListTest.java
public class MyListTest{
public static void main(String[] args)
{
MyList myList1 = new MyList();
String[] words1 = {"This", "is", "an", "example", "of", "text", "justification."};
int maxWidth1 = 16;
System.out.println("Input: ");
printInput(words1, maxWidth1);
System.out.println(" Output: ");
myList1.formatText(words1, maxWidth1);
myList1.printFormatedList();
}
public static void printInput(String[] words, int maxWidth)
{
System.out.print("Words = [");
for(int i = 0; i < words.length; i++)
{
System.out.print("\"" + words[i] + "\"");
if(i < words.length-1)
System.out.print(", ");
}
System.out.println("]");
}
}
Thank you.
*Edit*
MyList.java
public class MyList {
MyArrayList myList;
public MyList()
{
myList = new MyArrayList();
}
public MyArrayList formatText(String[] words, int maxWidth)
{
if(words == null || words.length == 0)
{
return myList;
}
StringBuilder strBldr;
int allCharsCount = 0;
int wordWidth;
int spacesWidth;
int lastIndex;
int eachLength;
int extraLength;
int count;
lastIndex = 0;
for(int i = 0; i < words.length; i++)
{
allCharsCount = allCharsCount + words[i].length();
if(allCharsCount + i - lastIndex > maxWidth)
{
wordWidth = allCharsCount - words[i].length();
spacesWidth = maxWidth - wordWidth;
eachLength = 1;
extraLength = 0;
if(i - lastIndex - 1 > 0)
{
eachLength = spacesWidth / (i - lastIndex - 1);
extraLength = spacesWidth % (i - lastIndex - 1);
}
strBldr = new StringBuilder();
for(int k = lastIndex; k < i - 1; k++)
{
strBldr.append(words[k]);
count = 0;
while(count < eachLength)
{
strBldr.append(" ");
count++;
}
if(extraLength > 0)
{
strBldr.append(" ");
extraLength--;
}
}
strBldr.append(words[i - 1]);
while(strBldr.length() < maxWidth)
{
strBldr.append(" ");
}
myList.add(strBldr.toString());
lastIndex = i;
allCharsCount = words[i].length();
}
}
strBldr = new StringBuilder();
for(int i = lastIndex; i < words.length - 1; i++)
{
allCharsCount = allCharsCount + words[i].length();
strBldr.append(words[i] + " ");
}
strBldr.append(words[words.length - 1]);
while(strBldr.length() < maxWidth)
{
strBldr.append(" ");
}
myList.add(strBldr.toString());
return myList;
}
public void printFormatedList()
{
System.out.println("[");
for(int i = 0; i < myList.size(); i++)
{
System.out.println("\"" + myList.get(i) + "\"");
}
System.out.println("]");
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
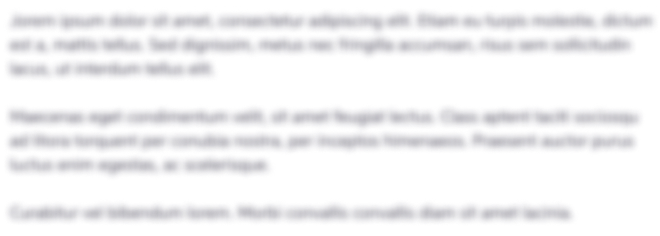
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started