Question
How do I randomly create ten Account/CDAccount objects and store them in one Hashmap and Treemap? I'm supposed to add on to this code from
How do I randomly create ten Account/CDAccount objects and store them in one Hashmap and Treemap?
I'm supposed to add on to this code from a past java class but I don't know where to start or how to do it. The requirements are:
- Provide 10 account numbers and search for the specific objects from Hashmap and Treemap
- For Account objects, print out the account number and balance; for CDAccount objects, print out the account number and mature balance; for objects that can't be found print out "The Account Number doesn't exist".
- Print out all Accounts created in Hashmap and Treemap.
Any help will be greatly appreciated, thank you.
//Account class
import java.util.Date;
//public class Account implements Comparable{ public class Account{ private long id; private double balance; private Date dateCreated; private static double annualInterestRate = 0.05; final static int number = 5;
Account() { dateCreated = new Date(); } Account(int ID, double init_balance) { id = ID; balance = init_balance; dateCreated = new Date(); } /*public Account(String first, String last, String state, long People, double startBalance) throws Exception { super(); balance = startBalance; id = People; }*/
public Date getdateCreatedObject() { return dateCreated; } public void setdateCreated(Date dateCreated) { this.dateCreated=dateCreated; } public String getdateCreated() { return dateCreated.toString(); } public long getid() { return id; } public void setid(int ID) { id = ID; } public double getbalance() { return balance; } public void setbalance(double new_balance) { balance = new_balance; } public static double annualInterestRate() { return annualInterestRate; } public static void setannualInterestRate(double new_interestRate) { annualInterestRate = new_interestRate; } public static double getannualInterestRate() { return annualInterestRate; } public double getMonthlyInterestRate() { return annualInterestRate/12; } public double getMonthlyInterest() { return balance * getMonthlyInterestRate(); } public void withdraw(double withdraw_amount) { setbalance(getbalance() - withdraw_amount); } public void deposit(double deposit_amount) { setbalance(getbalance() + deposit_amount); } /*@Override public int compareTo(Object o) { Account a = (Account)o; if (id > a.id) return 1; else if ( id < a.id) return -1; else return 0; }*/ @Override public boolean equals(Object o) { if ( o instanceof Account) { Account a = (Account) o; return (this.id == a.id); } return false; } @Override public String toString() { return ("Account id: " + this.id + "Account balance: " + this.balance); } }
// CDAccount
public class CDAccount extends Account { private double CDannualInterestRate=0.0; private int duration; CDAccount() { setDuration(0); setCDAnnualInterestRate(); } CDAccount(int duration, double CDAnnualInterestRate) { setDuration(duration); setCDAnnualInterestRate(); } CDAccount(int id, double balance, int duration) { super(id, balance); setDuration(duration); setCDAnnualInterestRate(); } public int getDuration() { return duration; } public void setDuration(int duration) { this.duration = duration; } public double getCDAnnualInterestRate() { return CDannualInterestRate; } public void setCDAnnualInterestRate() { this.CDannualInterestRate = Account.getannualInterestRate() + getDuration()/3 * 0.005; } @Override public double getMonthlyInterestRate() { return CDannualInterestRate/12; } @Override public double getMonthlyInterest() { return getbalance() * getMonthlyInterestRate(); } public String toString() { return String.format("%15s%20s%15s%30s ", "Account Number", "Initial Balance", "Rate(%)", "Date Created") + "================================================================================================ " + String.format("%15d%20.2f%15.2f%35s ", this.getid(), this.getbalance(), this.getCDAnnualInterestRate()*100, this.getdateCreated()); } }
// main class
public class main extends Account { public main(String[] args) { ArrayList
Step by Step Solution
There are 3 Steps involved in it
Step: 1
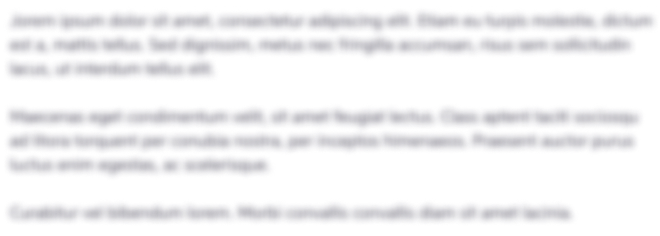
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started